Mathematics in Programming: Essential Insights

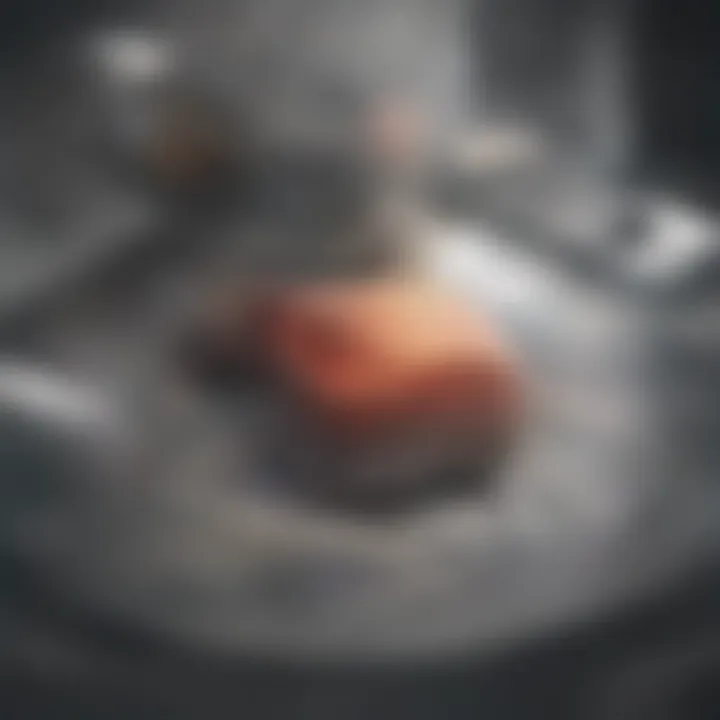
Intro
Mathematics serves as the backbone for much of what happens in programming. It creates a framework in which programmers can navigate complex problems and develop efficient solutions. The relevance of mathematical concepts in programming is not merely an academic consideration; it directly influences the quality and performance of software applications. This article intends to explore the subset of mathematics that equips developers with essential skills in problem-solving, algorithm design, and data structure management.
Guiding us through this intricate relationship, we will look at both foundational topics and advanced mathematical theories. With applications ranging from data analysis to machine learning and cryptography, mathematics remains integral to diverse programming disciplines. This discourse aims to offer valuable insights to students, researchers, educators, and professionals keen on grasping the necessary mathematical principles that enhance programming capabilities.
Key Research Findings
Overview of Recent Discoveries
Recent studies underscore how mathematical reasoning enhances algorithm efficiency. Computational complexity, a vital area of research, examines the resources required for algorithms. This research emphasizes the efficiency of mathematical methods in proving algorithmic performance. For instance, analysis of sorting algorithms reveals polynomial time complexity in various scenarios, affecting how developers choose methods based on efficiency.
Mathematics is also pivotal in areas such as data analysis and machine learning, where linear algebra and statistics play crucial roles. The ability to manipulate systems of equations and understand probability distributions is essential for crafting algorithms that learn from data. Notably, advanced topics such as Fourier transforms and optimization techniques are increasingly significant in artificial intelligence applications.
Significance of Findings in the Field
Mathematical insights are not just theoretical; they translate into practical programming skills. The evolving landscape of technology demands a workforce fluent in both programming and mathematics. Companies increasingly seek candidates who possess not only coding skills but also an acute understanding of underlying mathematical concepts.
"Mathematics is the language in which the laws of nature are written."
This statement highlights the fundamental nature of mathematics in comprehending and developing algorithms, models, and data structures.
Breakdown of Complex Concepts
Simplification of Advanced Theories
Breaking down complex mathematical theories into manageable portions is essential for programmers. For instance, concepts like graph theory or combinatorics can seem daunting. However, understanding basic concepts like vertices, edges, and paths can provide a strong foundation. Programmers tasked with optimizing network connectivity or creating efficient routes can leverage this understanding effectively.
Visual Aids and Infographics
For a clearer grasp of these principles, visual representation often aids comprehension. Infographics can illustrate connections between algorithms and their mathematical underpinnings. Simple visuals can effectively represent trees, graphs, and other structures, thereby aiding retention and understanding.
Prologue to Mathematics in Programming
Mathematics forms a significant foundation for programming, often intertwined in ways that are not immediately obvious. Understanding mathematical concepts allows programmers to approach problems with logic and rigor. This section of the article aims to elucidate the importance of mathematics in programming, focusing on the fundamental elements that enhance a programmer's ability to design algorithms and analyze data structures effectively.
Mathematics helps in breaking down complex problems into simpler parts. With mathematical literacy, programmers can apply various theories and concepts to devise efficient solutions. The connection between programming and mathematics is evident in many areas, from developing algorithms to optimizing code performance. As we explore this intersection, we will see why a solid mathematical background is not just advantageous but essential for success in programming.
"Mathematics is the key and door to the sciences." β Galileo
The Intersection of Math and Computer Science
The link between mathematics and computer science is profound. Mathematics provides the language to formally express concepts in computer science. At its core, programming often revolves around logical operations and problem-solving strategies that are fundamentally mathematical in nature. For instance, logic, which is a branch of mathematics, directly informs algorithm design and efficiency.
Logical structures, often represented as flowcharts or pseudocode, rely on a mathematical understanding of how conditions lead to different outcomes. Knowledge in discrete mathematics, for example, unveils concepts such as sets, graphs, and combinatorics that are vital in data structures and algorithms.
Moreover, mathematical proofs validate algorithms' correctness. When we analyze the performance and complexity of algorithms, we lean heavily on mathematical principles, utilizing notation and methods from calculus and algebra, ensuring our programs run effectively.
Why Programming Requires Mathematical Skills
Programming necessitates certain mathematical skills, which facilitate better analytic thinking and systematic approaches to problem-solving. A programmer who grasps mathematical principles can break down complex tasks, reason abstractly, and engage with algorithms more effectively. Some of the critical mathematical skills that are beneficial for a programmer include:
- Problem Solving: The ability to tackle problems logically and methodically.
- Analytical Thinking: Evaluating data and algorithms critically to assess effectiveness and efficiency.
- Statistical Understanding: Essential for fields like data science and machine learning, where interpreting data correctly is crucial.
- Logical Reasoning: Foundational for creating logical structures in programming.
- Numerical Methods: Useful in applications requiring optimization and approximations.
By honing these skills, programmers can enhance their programming capabilities and improve their employability. Mathematical literacy is not merely a recommendation; it is a requirement for those aspiring to excel in the programming field. It equips developers with the tools necessary to understand and utilize complex systems, thereby adding significant value to their work.
Basic Mathematical Concepts for Programmers
Mathematical concepts form the backbone of effective programming. These concepts enable programmers to think logically and solve problems systematically. A strong grasp of basic math allows for better comprehension of algorithms, data structures, and the underlying principles of computer science. This section will explore fundamental topics, including arithmetic operations, algebraic principles, and the significance of functions and graphs.
Arithmetic Operations
Arithmetic operations are the foundation of all mathematics and programming. Understanding how to perform addition, subtraction, multiplication, and division is crucial.
- Addition combines numbers, producing a sum.
- Subtraction finds the difference between numbers.
- Multiplication scales numbers and finds the product.
- Division splits numbers into equal parts, providing a quotient.
In programming, these operations are frequently used. For example, calculating the cost of items in an online store involves summing prices or applying discounts through subtraction. Additionally, algorithms often require these operations for manipulating data and controlling flow.
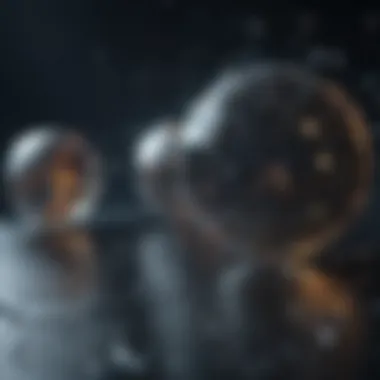
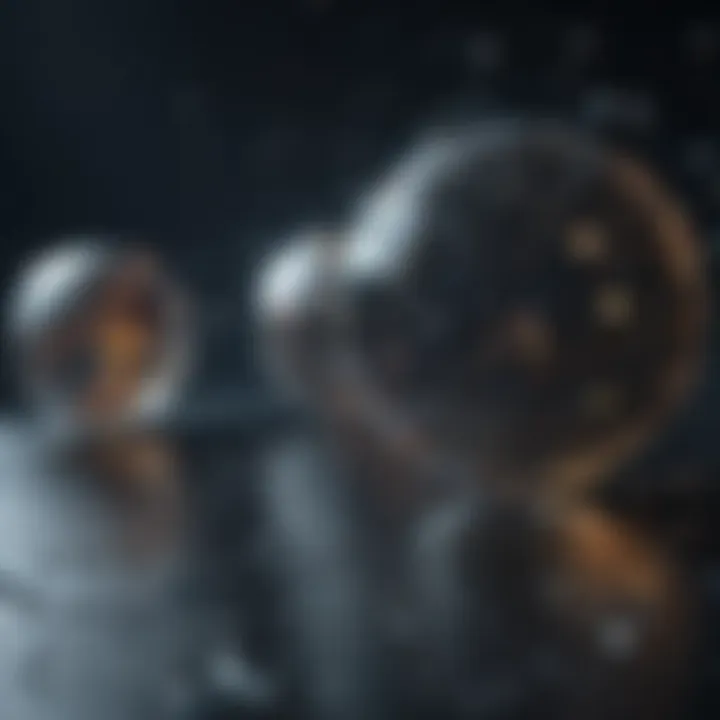
Algebra and Variables
Algebra introduces the concept of variables, which are essential in programming. A variable acts as a placeholder for data values. Understanding how to manipulate variables through algebraic expressions enables programmers to create dynamic and flexible code.
- Expression Evaluation: In programming, expressions often include variables. For instance, if and , then the expression evaluates to . This applies in defining functions where outputs depend on input values.
- Equations: Setting equations helps in problem-solving. For example, the equation represents a linear function, which can model various programming tasks, like linear regression.
Algebra also aids in understanding loops and conditional statements, allowing programmers to derive values during execution.
Functions and Graphs
Functions represent a core concept in mathematics and programming. Functions map inputs to outputs, serving as reusable blocks of code. Understanding the behavior of functions is vital for programming.
- Defining Functions: A function takes parameters and yields a result. In Python, a simple function can be defined as:This function demonstrates basic arithmetic through reusable code.
- Graph Representation: Graphs visualize function behavior. Analysts often use graphs to observe trends or relationships. For example, plotting a function can illustrate how output changes with different inputs, assisting in debugging and optimizing code.
Discrete Mathematics and Its Relevance
Discrete Mathematics serves as a cornerstone for numerous programming principles. It includes various topics such as logic, set theory, relations, and graph theory, all of which are pivotal in computer science. Understanding these concepts is essential for intertwining mathematical thinking with programming logic. In contrast to continuous mathematics, which deals with real numbers and calculus, discrete mathematics focuses on distinct, separate values. This makes it particularly relevant in fields like algorithms, data structures, and computer networks.
Moreover, knowledge of discrete mathematics enables programmers to think critically and systematically. It aids in designing algorithms, understanding data structures, and optimizing performance. As a programmer, mastering discrete mathematics can directly enhance both the efficiency and effectiveness of the solutions you create.
"Discrete Mathematics is the foundation upon which much of computer science is built."
Sets, Relations, and Functions
Sets are fundamental units in discrete mathematics. A set is a collection of distinct objects, considered as an object in its own right. For example, a set of programming languages can be written as
Languages = Python, Java, C++, JavaScript
Relations describe the connections between elements in sets. For instance, a relation can illustrate how students relate to their courses. A function, which is a specific type of relation, associates each element of a set with exactly one element of another set. Understanding these concepts is crucial for data organization and manipulation in programming.
Logic and Boolean Algebra
Logic forms the backbone of programming. Boolean algebra operates on binary variables, using logical operations like AND, OR, and NOT. Programmers use these operations to construct logical statements that control the flow of a program.
The use of Boolean logic helps in decision-making processes within algorithms. For example, if a program checks whether the input is valid, it might use a Boolean equation:
if (input is valid) // proceed // handle error
Thus, a strong grasp of logic and Boolean algebra is necessary for every programmer. It supports the development of efficient and effective algorithms.
Graph Theory in Programming
Graph theory explores relationships through graphs, made up of vertices and edges. This concept applies to various programming areas such as networking, where nodes represent devices, and edges symbolize connections between them. Many algorithms, like Dijkstra's for finding the shortest path, rely heavily on graph theory.
Understanding graph properties such as trees, cycles, and connectivity is vital for optimizing solutions in data structures and network design. Programmers who are adept in graph theory can devise algorithms for efficiently navigating and manipulating data relationships.
Algorithms and Mathematical Principles
Algorithms are central to programming. They provide a systematic approach to solving problems, enabling programmers to create efficient and effective solutions. Understanding mathematical principles is essential for developing optimal algorithms as it allows for informed decision-making regarding performance and resource usage.
The Role of Algorithms in Problem Solving
Algorithms serve as a blueprint for problem-solving in programming. They break down complex tasks into manageable steps. For example, sorting a list or searching for an element in a dataset can be achieved through specific algorithms like QuickSort or binary search. These algorithms dictate how data is manipulated and can significantly affect the overall program efficiency.
Key Contributions of Algorithms:
- Efficiency: Algorithms determine the speed of execution and resource consumption.
- Correctness: A well-constructed algorithm ensures accurate results.
- Scalability: Efficient algorithms can handle increased data sizes without drastic degradation in performance.
Complexity Analysis
Complexity analysis assesses an algorithm's efficiency. Two primary considerations are time complexity and space complexity. Time complexity measures how the execution time of an algorithm changes with the size of the input. Space complexity evaluates the amount of memory required by an algorithm.
To effectively analyze algorithms, Big O notation is used. It characterizes the algorithm's performance in the worst-case scenario, providing insights into scalability. For instance:
- O(1) - constant time
- O(log n) - logarithmic time
- O(n) - linear time
- O(n^2) - quadratic time
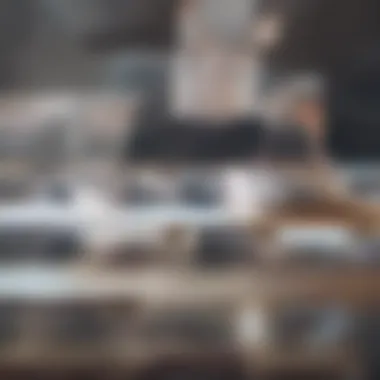
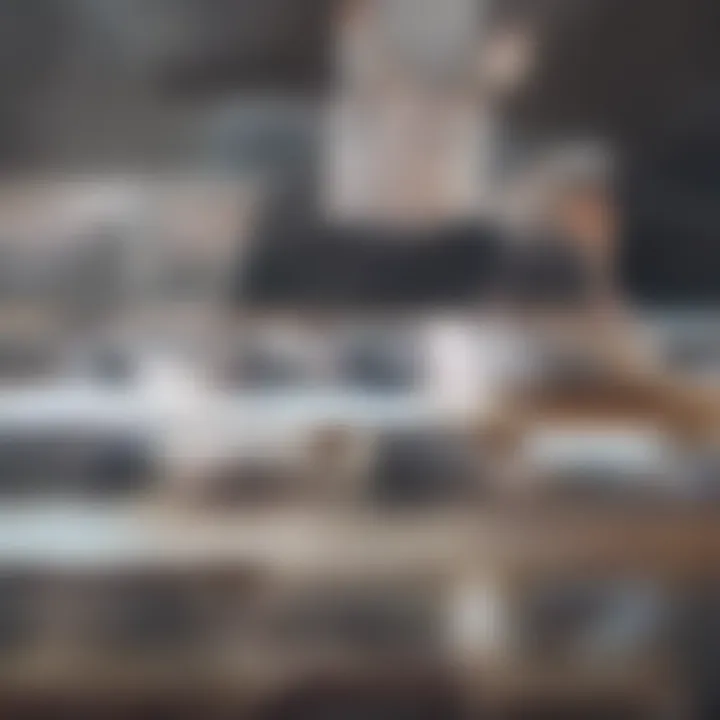
Understanding these can help developers make better choices while coding.
Mathematical Induction and Recursion
Mathematical induction is a method of proof that is essential in computer science for establishing the correctness of algorithms. It consists of two parts: the base case and the inductive step. The base case verifies that the statement holds for the initial value, while the inductive step demonstrates that if it holds for an arbitrary case, it holds for the next. This often parallels recursive algorithms where the solution to a problem depends on the solution to smaller instances of the same problem.
Recursion is a programming technique where a function calls itself to solve smaller instances of the same problem. This method can lead to elegant solutions, particularly for tasks like tree traversal or sorting. However, care must be taken to avoid excessive memory use or infinite loops by ensuring that the recursive calls ultimately lead to a base case.
A well-designed algorithm not only performs well but also remains comprehensible and maintainable.
Statistical Methods for Data Science
Statistical methods are crucial in the field of data science, especially in programming. They supply the tools necessary for analyzing data sets, making predictions, and drawing conclusions. Understanding statistical concepts is essential for programmers working with data, as it informs decision-making based on empirical evidence.
Key Elements of Statistical Methods
Statistical methods can be broadly categorized into descriptive and inferential statistics, each serving unique purposes in data analysis. Descriptive statistics help summarize and describe the characteristics of data through measures such as mean, median, and standard deviation. It gives a quick overview of the data's trends and patterns, aiding in initial assessments. On the other hand, inferential statistics allow for conclusions about a population based on a sample. This involves hypothesis testing, confidence intervals, and regression analysis. These methods are vital for making predictions and facilitating data-driven decision-making.
Benefits of Statistical Methods in Programming
- Enhanced Analysis: Statistical methods provide frameworks for understanding complex data sets, enabling programmers to glean insights from raw data.
- Prediction and Forecasting: With inferential statistics, programmers can create models that predict future outcomes based on historical data trends.
- Data Validation: Statistical techniques provide means to validate hypotheses, ensuring that conclusions drawn from data analysis are sound.
- Improved Decision Making: By applying statistical methods, developers can create software that supports users in making informed choices backed by data analytics.
Statistical methods are not merely add-ons in the programming toolkit. They form the backbone of effective data handling processes, influencing both software design and user experience.
Descriptive and Inferential Statistics
Descriptive statistics play a foundational role in any data science project. They serve as essential tools for summarizing data. Common metrics include:
- Mean: The average of the dataset, offering a central value.
- Median: The middle value when the data is ordered, providing insights into the distribution.
- Mode: The most frequently occurring value, useful in categorical data.
- Standard Deviation: A measure of variability, indicating how much individual data points differ from the mean.
These metrics help programmers understand the distribution of data before any in-depth analyses. In contrast, inferential statistics enables programmers to draw conclusions beyond the immediate data set. This includes methods such as:
- Hypothesis Testing: It assists in determining whether a premise about a dataset holds true.
- Regression Analysis: Used for predicting the relationship between variables and understanding data trends.
Both types are integral to developing robust data-driven applications.
Probability Theory
Probability theory underpins many statistical methods. It provides a formal framework for quantifying uncertainty. Essentially, it allows programmers to model the likelihood of various outcomes based on the data available.
Some key concepts include:
- Random Variables: Represent outcomes in probabilistic terms.
- Probability Distributions: Describe the likelihood of various outcomes within a dataset, such as normal or binomial distributions.
- Bayesian Methods: Focus on updating the probability of a hypothesis as more evidence becomes available.
Probability not only helps programmers assess risks but also enhances predictive models used in machine learning and AI.
Statistical methods empower programmers to make informed decisions and derive meaning from complex data sets. They transform raw numbers into actionable insights, leading to better software solutions and innovative applications.
Linear Algebra in Programming
Linear algebra plays a significant role in programming, especially within the realms of computer science and software development. It provides the mathematical framework required to understand and implement algorithms that manipulate data structures effectively. As such, a solid grasp of linear algebra is beneficial for programmers, data scientists, and engineers alike. Understanding this discipline facilitates the representation and transformation of data in multidimensional spaces. This can greatly enhance the capability to process complex datasets and develop robust algorithms.
In programming, key components like vectors and matrices foster the representation of vast amounts of data. They serve as fundamental building blocks in a variety of applications, particularly in data analysis, scientific computing, and artificial intelligence. Notably, the use of linear algebra significantly optimizes computation performance, enabling efficient data manipulation and retrieval operations.
Additionally, knowledge of linear algebra can help programmers design and analyze algorithms more rigorously, from basic sorting techniques to advanced machine learning models. As programming continues to evolve, its integration with linear algebra remains crucial.
Vectors and Matrices
Vectors and matrices are core concepts in linear algebra that find extensive use in programming. A vector can be thought of as an ordered array of numbers, while a matrix represents a two-dimensional array of numbers. Both structures are pivotal for representing data geometrically.
Key properties of vectors include:
- Direction and Magnitude: They can represent both a point in space and a direction, which is vital for 3D graphics and simulations.
- Operations: Basic operations such as addition, subtraction, and scaling can be performed, allowing for complex computations on datasets.
Matrices facilitate numerous mathematical operations:
- Transformations: They allow for the transformation of shapes and images, a critical aspect of graphical programming.
- Solving Systems of Equations: Matrices provide efficient methods to solve linear equations, which are fundamental in many algorithms.
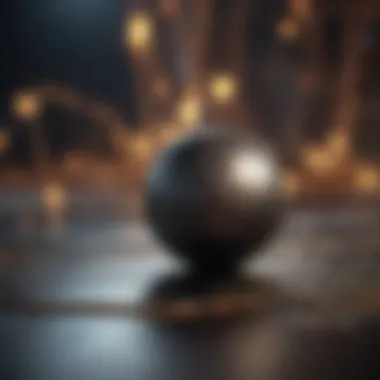
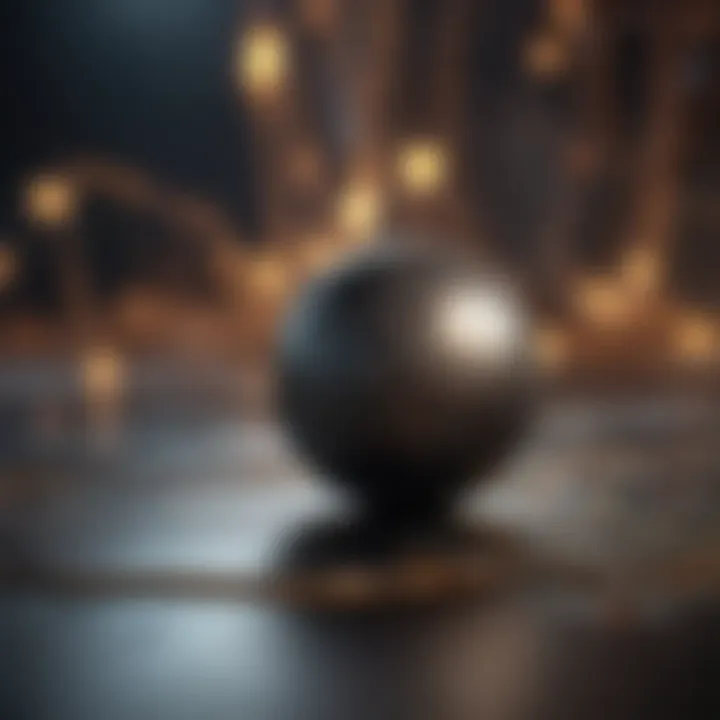
The representations of data using these structures can dramatically affect performance and storage. For instance, vectors and matrices can enable programmers to harness SIMD (Single Instruction, Multiple Data) operations, which significantly enhances processing speed.
Applications in Machine Learning
Machine Learning heavily relies on linear algebra, most prominently vectors and matrices, to handle large datasets. One primary application is in representing data points. Each data point can be viewed as a vector in a high-dimensional space. This concept allows algorithms to perform calculations such as distances and angles, essential for many ML models.
Some important applications of linear algebra in machine learning include:
- Neural Networks: In deep learning, weights and biases are represented as matrices. The training of these networks involves matrix multiplications and operations.
- Principal Component Analysis (PCA): PCA uses linear algebra to reduce the dimensionality of data, enhancing interpretability while preserving essential information.
- Support Vector Machines (SVM): SVMs utilize hyperplanes defined in high-dimensional spaces, which can be expressed and manipulated using vectors and matrices.
Understanding linear algebra not only aids in developing machine learning algorithms, but also empowers programmers to think critically about the data structures they use and the operations they perform. In summary, the effectiveness of programming in data science and machine learning hinges on a clear understanding of linear algebra principles!
Calculus and Its Applications
Calculus is an essential branch of mathematics that focuses on change. Its applications in programming are manifold, influencing various domains like physics simulations, graphics rendering, and data analysis. By understanding calculus, programmers can develop more sophisticated algorithms and enhance their problem-solving capabilities. In this section, we shall explore derivatives and integrals, and optimization strategies relevant to programming.
Understanding Derivatives and Integrals
Derivatives represent the rate of change of a function with respect to a variable. For programmers, the concept of derivatives translates to measuring how outputs are affected by small changes in inputs. For example, in a machine learning context, derivatives help in understanding how a modelβs prediction improves as it learns from new data.
Integrals, on the other hand, deal with accumulation. They allow one to compute areas under curves, which is crucial in various programming applications such as animations, graphical modeling, and statistical calculations. In practical code implementations, functions can be represented and calculated using integral calculus to determine probabilities or assess risk in algorithms.
"Understanding these concepts not only aids in theoretical knowledge but also empowers developers to create more efficient and accurate computational models."
Optimization in Programming
Optimization is the process of making a system or design as effective or functional as possible. In programming, this often relates to enhancing the performance of algorithms, reducing execution time, or minimizing resource consumption. Optimizing code frequently involves applying methods derived from calculus.
Key considerations in optimization include:
- Finding Local and Global Minima: Using derivatives, programmers can identify points at which functions achieve minimum values. This is crucial for making decisions based on cost, timings, or resource use.
- Gradient Descent: This algorithm is widely used in machine learning to optimize the performance of models. By iteratively moving towards the lowest gradient, it efficiently fine-tunes the parameters of a model.
- Constraint Optimization: Many real-world problems carry constraints. Calculus aids in formulating these constraints to suit specific programming needs by means of Lagrange multipliers and other techniques.
Mathematics in Cryptography
Mathematics is fundamental to the field of cryptography, underpinning its theories and operational methods. Understanding the mathematical principles behind cryptographic algorithms is essential for grasping how data security mechanisms work. Cryptography relies on various mathematical branches, especially number theory and combinatorics, to protect information from unauthorized access. The use of mathematical models and algorithms ensures that sensitive information remains confidential, thus forming the backbone of modern secure communications.
The importance of mathematics in cryptography lies in its ability to create robust systems that can prevent attacks. Mathematical constructs help in designing encryption algorithms, managing keys, and generating secure protocols. A solid mathematical foundation allows cryptographers to analyze the strength of algorithms against potential vulnerabilities. As technology evolves, mathematical methods continue to adapt, enabling stronger cryptographic solutions.
"Cryptography is as much about the correct application of mathematics as it is about the mathematics itself."
Basic Concepts of Cryptography
Cryptography involves a range of basic concepts that help protect information from adversaries. One of the fundamental concepts is the notion of plaintext and ciphertext. Plaintext refers to the original message that needs protection, whereas ciphertext is the scrambled version that results from applying an encryption algorithm.
Another vital element is the key, which is a piece of information used in the encryption and decryption processes. The security of the cryptographic scheme often hinges on the complexity of this key. There are symmetric and asymmetric key systems. In symmetric systems, the same key is used for both encryption and decryption. In contrast, asymmetric systems use a pair of keys β a public key for encryption and a private key for decryption.
Additionally, hash functions play a significant role in cryptography, providing a mechanism to ensure data integrity. A hash function takes an input and produces a fixed-size string, representing the original data. Comparing hash values can determine if data has been tampered with or altered.
The Role of Number Theory
Number theory is vital in cryptography, particularly in the development of encryption algorithms. It involves the study of integers and their properties, making it suitable for creating secure keys and algorithms. Prime numbers are a central focus within number theory and are used extensively in schemes like RSA (RivestβShamirβAdleman).
RSA, one of the first public-key cryptosystems, relies on the difficulty of factoring the product of two large prime numbers. This complexity provides a strong basis for security since, while it is easy to multiply two primes, it is extremely challenging to reverse the process. Other cryptographic algorithms, like Diffie-Hellman, also leverage properties of further number theory concepts to establish secure communications.
Moreover, modular arithmetic, which involves integers and their remainders, is crucial in cryptographic processes. Operations in modular arithmetic facilitate efficient calculations, mandatory for executing algorithms smoothly and securely.
Understanding number theory grants cryptographers an insight into potential weaknesses in existing structures. As these mathematical theories evolve, they will introduce innovations that can strengthen future cryptographic methods.
Finale and Future Directions
Mathematics plays an integral role in the domain of programming as it offers critical frameworks and tools essential for effective problem solving. This article has elaborated on various mathematical concepts, their applications, and their relevance in programming. As technology evolves, so does the necessity for a deeper comprehension of these mathematical foundations. One pivotal consideration is that mathematical literacy enhances a programmer's capacity to engage with advanced computational problems.
In future developments, the importance of mathematics will only increase. New domains, like artificial intelligence and quantum computing, will demand stronger mathematical insights and skills. Additionally, educators and industry professionals must prioritize teaching fundamental mathematics alongside programming languages, tools, and technologies.
The Ongoing Importance of Mathematics in Technology
The relationship between mathematics and technology is becoming increasingly pronounced. As technology advances, the models and algorithms that underpin software necessitate more complex mathematical foundations. Whether programming for data analysis, cryptography, or machine learning, mathematical concepts remain at the center.
Here are a few key points to consider regarding the ongoing importance of mathematics in technology:
- Mathematical models aid in developing predictive algorithms used in various industries, from finance to healthcare.
- Understanding linear algebra is critical for implementing machine learning algorithms, which rely on large datasets and various computations.
- The principles of calculus are essential when calculating changes and optimizing function outcomes in software applications.
- Cryptography relies heavily on number theory, ensuring security in communications and transactions through mathematical proofs.
"In the evolving landscape of technology, mathematical fluency is not just an asset; it is a necessity that underpins the efficacy and security of programming practices."
As programming becomes more intertwined with mathematics, professionals must stay abreast of advancements in both fields. Continuous learning will allow programmers to adapt and implement mathematical concepts effectively, ensuring successful outcomes in their projects.