Exploring Graph Shortest Path Algorithms in Depth
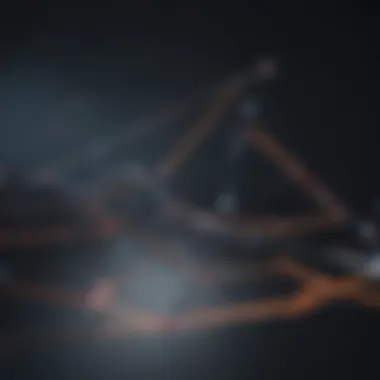
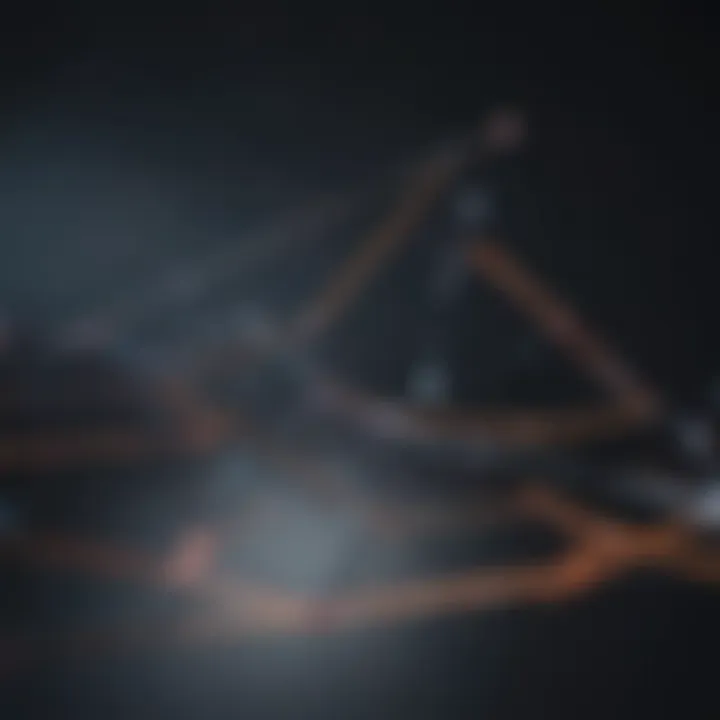
Intro
Graph shortest path algorithms play a crucial role in a variety of fields, from computer science to transportation networks. These algorithms allow for optimal navigation within complex structures, thereby enhancing efficiency in both digital and physical realms. Understanding the underlying principles of these algorithms not only illuminates their practical applications but also raises awareness about their limitations and challenges.
In the modern age of data and connectivity, the importance of shortest path calculations continues to grow. Applications range from routing software that directs drivers to their destinations, to social network analysis that reveals the quickest paths of interaction among users. The exploration of these algorithms provides valuable insight into their mechanics, relevance, and the latest innovations in this rapidly evolving domain.
This article will unpack the mechanics of graph shortest path algorithms, dissect their significance in distinct fields, and outline both established and emerging techniques. While diving into the mathematical and computational aspects, we will ensure that concepts remain approachable, providing readers with both clarity and depth in understanding.
Prelude to Graph Theory
Graph theory serves as a foundational cornerstone in the realm of computer science and data analysis. Understanding the behavior of graphs is crucial for various applications, including network design, optimization problems, and algorithm development.
The importance of graph theory stems from its ability to model complex relationships and interactions within a multitude of systems. Whether it be social networks, transportation logistics, or telecommunications, the principles derived from graph theory apply universally. By delving into graph structures and their properties, we gain insights that can lead to more efficient algorithms, specifically in the context of shortest path calculations.
Definition and Importance
Graph theory studies the nature of graphs, which are mathematical representations consisting of vertices connected by edges. A vertex, or node, represents an entity, while an edge symbolizes a relationship or connection between these entities. The understanding of these elements helps in solving practical problems in ways that are both efficient and effective.
The relevance of graph theory cannot be overstated. It assists in optimizing resource allocation and pathfinding, enabling systems to function more smoothly. Key areas that benefit from graph theory include:
- Network Routing: Determining optimal paths in data transmission networks.
- Urban Planning: Analyzing traffic flow and infrastructure design.
- Social Network Analysis: Understanding relationships and influences within communities.
Graph Structures
The structures of graphs are diverse, encompassing various forms that serve distinct purposes. The main types of graphs include:
- Directed Graphs: Where edges have a direction, indicating a one-way relationship. This is applicable in scenarios like web pages and citations.
- Undirected Graphs: In which edges have no direction, reflecting mutual relationships. Consider this for social networks.
- Weighted Graphs: Edges are assigned weights, allowing for more intricate analysis like cost minimization.
- Unweighted Graphs: All edges are treated equally, simplifying representation and analysis in many algorithms.
The choice of graph structure directly influences the algorithmic approach and computational complexity. Thus, comprehending the various graph structures enhances our ability to devise and apply effective algorithms in the search for shortest paths. Stakeholders such as researchers and developers must carefully consider these aspects to optimize the performance of their applications.
Graph Representation Techniques
Graph representation techniques are foundational to understanding how graph algorithms operate, particularly shortest path algorithms. The choice of representation affects both the efficiency of algorithm execution and the complexity of implementation. Understanding these various techniques is crucial for students and professionals who aim to optimize their use of graphs in computational tasks.
Adjacency List
An adjacency list is an efficient way to represent a graph in computer science. In this representation, each vertex (or node) has a list of the nodes it is directly connected to. This method is particularly beneficial for sparse graphs where the number of edges is much lower than the number of vertices.
The benefits of using an adjacency list include:
- Memory Efficiency: Less memory is needed compared to other representations like the adjacency matrix, especially for large graphs with few edges.
- Dynamic Updates: It allows for easy addition and removal of edges without restructuring the entire graph.
- Fast Traversal: Algorithms that need to explore the neighbors of a vertex can do so quickly, which is essential in shortest path algorithms.
While effective, adjacency lists can also have drawbacks. Accessing the adjacent vertices of a specific node may require more time than other representations, particularly in dense graphs.
Adjacency Matrix
An adjacency matrix is a two-dimensional array used to represent a graph. In this matrix, both rows and columns represent vertices, and the value at each cell signifies whether an edge exists between those vertices. This representation provides constant time complexity for checking the existence of an edge, making it useful for algorithms requiring frequent edge queries.
Key aspects of the adjacency matrix include:
- Simplicity: Straightforward structure that is easy to understand and implement.
- Quick Edge Lookup: Checking for the presence of an edge between any two vertices is simple and takes constant time.
- Dense Graphs: More efficient to use for graphs where the number of edges is close to the maximum possible.
However, there are limitations. The memory usage can be substantial for sparse graphs since the size of the matrix scales with the square of the number of vertices. This often leads to wasted space when many cells contain no data.
Edge List
An edge list is another representation of a graph that consists of a list of all edges. Each edge can be represented as a pair (or triplet, if weights are included) that determines the connection between two vertices. This representation is simple and provides a clear view of all connections.
Consider the following key points about edge lists:
- Simplicity and Clarify: Easy to implement and understand, making it ideal for educational purposes.
- Flexibility: Simple to modify when edges change, which is particularly useful when dealing with dynamic graphs.
- Space Efficiency: Consumes less space compared to an adjacency matrix in sparse graphs, since it directly lists only existing edges.
Nevertheless, edge lists have their downsides. Efficient traversal can be more complicated, as one must iterate through the entire list to find neighboring vertices. This could affect the performance of algorithms relying on direct access to neighbors.
In summary, the choice of graph representation technique plays a crucial role in algorithm efficiency and implementation ease. Each method has its strengths and weaknesses; understanding them enables better application of graph algorithms in real-world scenarios.
Overview of Shortest Path Algorithms
Shortest path algorithms serve a crucial role in graph theory, particularly in solving problems that require the identification of the most efficient route between two nodes. In a world increasingly driven by data and connectivity, understanding these algorithms is essential across various disciplines such as computer science, transportation, and operations research.
By optimizing routes, these algorithms not only save time but also reduce costs and resource consumption. Their importance is not limited to theoretical applications; they manifest in real-world systems including GPS navigation, network routing protocols, and urban traffic management. Thus, delving into shortest path algorithms unveils significant benefits, as well as challenges that must be navigated for successful implementation.
The purpose of this section is to provide a foundational understanding of the role that shortest path algorithms play, alongside their inherent challenges and the complexities designers must consider in their use.
Purpose and Challenges
The primary objective of shortest path algorithms is to identify the least costly path between two nodes within a weighted graph. Here, 'cost' can represent various factors such as distance, time, or any resource that is finite and subject to minimization.
However, practitioners face several challenges:
- Dynamic Graphs: Changes in graph structure may affect path calculations. For example, in a traffic scenario, new roadblocks might require the algorithm to reroute.
- Negative Edge Weights: Certain algorithms, such as Dijkstraโs, struggle with graphs containing negative weights, complicating the outcome of route calculations.
- Computational Complexity: Some algorithms may not perform efficiently on large datasets, leading to performance issues in systems that depend on real-time calculations.
Understanding these challenges aids developers in selecting the appropriate algorithm or modifying existing methods to suit their needs. It also prompts researchers to innovate and create more efficient tools.
Complexity Considerations
Complexity remains a fundamental component when discussing shortest path algorithms. It serves as a measure of the resources required to execute an algorithm, particularly in terms of time and space.
- Time Complexity: Different algorithms exhibit varied performance patterns. For instance, Dijkstraโs algorithm is typically efficient for sparse graphs, whereas Floyd-Warshall, which calculates all pairs shortest path, has a time complexity of O(n^3), making it less suitable for large datasets.
- Space Complexity: The amount of memory required for storing data structures also varies. Algorithms that use adjacency matrices may consume more space compared to those utilizing adjacency lists, especially in sparse graphs.
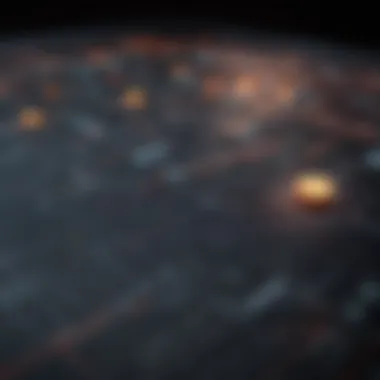
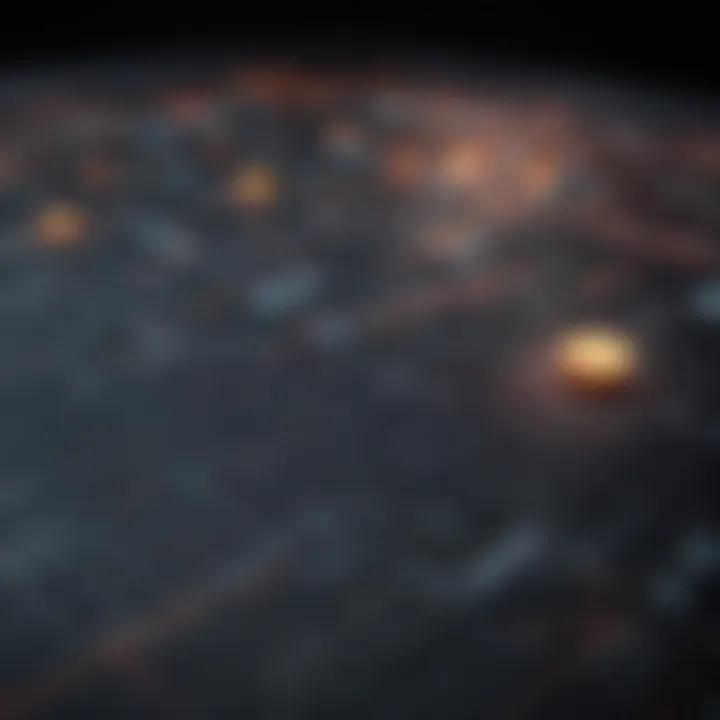
As the demands for real-time data processing grow, the focus on optimizing both time and space complexities becomes paramount in algorithm design. Being aware of these complexities can lead to more informed choices about which algorithm to deploy in specific scenarios.
Dijkstra's Algorithm
Dijkstra's Algorithm is a cornerstone in the domain of graph theory and shortest path calculations. Its significance in this article lies in its widespread use and effectiveness in finding the shortest paths from a single source to all other vertices in a weighted graph. Notably, it handles non-negative weights, making it essential in fields such as networking, transportation, and geographical mapping.
One of the main advantages of Dijkstra's Algorithm is its relatively efficient performance for smaller and medium-sized graphs. The simplicity of implementation, combined with its mathematical foundation, grants users confidence in its reliability. However, it is crucial to acknowledge the limitations when applied to certain types of graphs, particularly those containing negative weight edges. Understanding both the strengths and weaknesses can illuminate the best scenarios for using this algorithm.
Algorithm Functionality
The core functionality of Dijkstra's Algorithm revolves around the technique of maintaining a priority queue. Hereโs how it operates:
- Initialization: Start by assigning a distance value to every node. Set the distance to 0 for the source node and infinity for all other nodes.
- Priority Queue Utilization: Use a priority queue to fetch the node with the smallest distance value.
- Relaxation Process: For each adjacent node, check if the current known distance is greater than the distance through the new node. If it is, update the distance.
- Repeat: Continue this process until every node's shortest distance is found.
This systematic approach allows Dijkstra's Algorithm to efficiently compute the shortest paths in graphs without negative cycles.
Pseudocode Analysis
To better understand how Dijkstra's Algorithm functions, here is a simplified version of its pseudocode:
This pseudocode encapsulates the main elements of the algorithm, illustrating how distances are calculated, updated, and how nodes are processed in order to derive the final shortest path results.
Time Complexity
The time complexity of Dijkstraโs Algorithm is primarily influenced by the data structure used for the priority queue. When a binary heap is used, the time complexity is generally O((V + E) log V), where V is the number of vertices and E is the number of edges in the graph. However, if a Fibonacci heap is employed, this can be improved to O(E + V log V).
This logarithmic growth in performance is invaluable for dealing with large graphs, but it is important to remain mindful of the algorithm's limitations, particularly its inappropriate application to graphs with negative weight edges. Such considerations emphasize the need for comprehensive understanding when choosing to implement Dijkstra's Algorithm in practical applications.
Bellman-Ford Algorithm
The Bellman-Ford algorithm is integral to the study of shortest path algorithms in graph theory. This algorithm stands out because it can handle graphs with negative weight edges, unlike Dijkstraโs algorithm. Its importance lies in its versatility and capability, providing solutions in scenarios that involve uncertainties in edge weights, which are common in various fields such as telecommunications and finance.
Algorithmic Approach
The Bellman-Ford algorithm operates through a systematic relaxation process across all edges in the graph. It iteratively updates the shortest path estimates for each vertex. The primary steps are as follows:
- Initialization: Set the distance to the source vertex to zero and all other vertices to infinity, signifying that they are initially unreachable.
- Relaxation: For each edge in the graph, if the distance to the destination vertex can be shortened by taking the edge from the source vertex, update it.
- Repeat: This process repeats for a total of |V| - 1 times, where |V| is the number of vertices.
- Negative Cycle Detection: After the relaxation steps, an additional pass through the edges checks for further updates. If any distance can still be decreased, a negative weight cycle exists.
This algorithm provides a clear procedural framework that facilitates effective analysis in various practical applications, particularly in fields where negative weights can arise, such as in cost estimations and modeling complex systems.
Applications and Limitations
The applicability of the Bellman-Ford algorithm stretches across several domains:
- Network Routing: In data networks, it assists in determining optimal routing paths even when costs can fluctuate.
- Finance: When modeling financial transactions and the associated risks, it provides essential insights for cost-efficiency.
- Game Development: In pathfinding applications within games, it helps to navigate complex terrains where path costs can vary due to game mechanics.
However, the algorithm does face limitations:
- Time Complexity: With a time complexity of O(VE), it can become inefficient for very large graphs, where V is vertices and E is edges. Thus, for dense graphs, its performance can lag compared to others like Dijkstraโs algorithm.
- Lack of Heuristic Approach: Unlike A* search, it does not utilize heuristic methods to guide the search process, making it less optimal for certain applications.
Understanding both applications and limitations of the Bellman-Ford algorithm is essential for making informed decisions about its use in real-world scenarios.
The Bellman-Ford algorithm serves a crucial function in computing shortest paths in graphs containing negative weights, showcasing its essential nature in network analysis and optimization.
A Search Algorithm
The A* search algorithm stands out as a prominent method in the realm of pathfinding, particularly due to its efficiency and effectiveness in diverse applications. A* integrates concepts from both Dijkstra's algorithm and greedy algorithms, balancing exploration and exploitation. The key feature of A* is its ability to utilize heuristic functions, which significantly enhances its performance in finding shortest paths.
Optimizing pathfinding in various domains, A* has gained popularity in fields such as logistics, video games, and robotics. It allows for intelligent searching through graphs and maps, making it essential for applications requiring real-time navigation and decision-making. The implications of A* extend far beyond mere computational efficiency; its performance can dictate the usability and responsiveness of systems reliant on pathfinding.
Heuristic Functions
Heuristic functions are integral to the functionality of the A* algorithm. These functions evaluate potential paths and guide the search towards the most promising routes. Simply put, a heuristic function estimates the cost from a given node to the goal, providing insight that prevents unnecessary exploration of less favorable paths.
Choosing an appropriate heuristic is critical. It must be admissible, meaning it should never overestimate the actual cost to reach the target. Common heuristic functions include the Euclidean distance and the Manhattan distance, each applicable under different circumstances. Evaluating these functions boosts A*'s effectiveness by allowing it to explore routes with the lowest expected total cost sooner than others.
"The quality of the heuristic function directly impacts the performance of the A* algorithm, influencing both speed and accuracy in finding the shortest path."
Pathfinding Applications
A* finds practical application across various sectors. In transportation networks, it assists in route optimization, reducing travel time and costs. The ability to handle dynamic inputs, such as real-time traffic conditions, further enhances its utility.
In video game development, A* serves as a core mechanism for non-player character movement. It allows characters to navigate complex environments while adapting to barriers and obstacles, thus providing realistic interactions.
Robotics is another arena benefiting from A*. Autonomous robots use it for navigating environments, avoiding hazards, and optimizing routes for tasks such as delivery and exploration.
In summary, the A* search algorithm is a vital tool in pathfinding. Its reliance on heuristic functions elevates its performance, making it applicable in numerous fields. By understanding A*, one can appreciate how the algorithm functions and the significant impact it has on enhancing computational efficiency and user experience.
Floyd-Warshall Algorithm
The Floyd-Warshall algorithm is a fundamental technique for solving the all-pairs shortest path problem in a weighted graph. Its significance lies in its ability to compute the shortest paths between every pair of vertices, making it particularly useful in scenarios where information is needed about multiple connections simultaneously. This algorithm exemplifies a powerful dynamic programming approach, allowing for efficient computation despite the potential for complex graph structures.
All-Pairs Shortest Paths
In many real-world applications, knowing the shortest path between all pairs of nodes is essential. The Floyd-Warshall algorithm achieves this mathematically by iteratively refining estimates of the shortest path lengths. Unlike single-source algorithms, Floyd-Warshall considers all vertices as intermediate points, optimizing paths between every combination of start and destination. This makes it particularly advantageous in dense graphs where multiple connections exist. A key feature of this algorithm is its clear formulation:
- Initialize a distance matrix, where each entry is either the edge weight or infinity if no direct edge exists.
- Update the distance matrix iteratively, using each vertex as a possible intermediate node.
- Continue until all possibilities are exhausted.

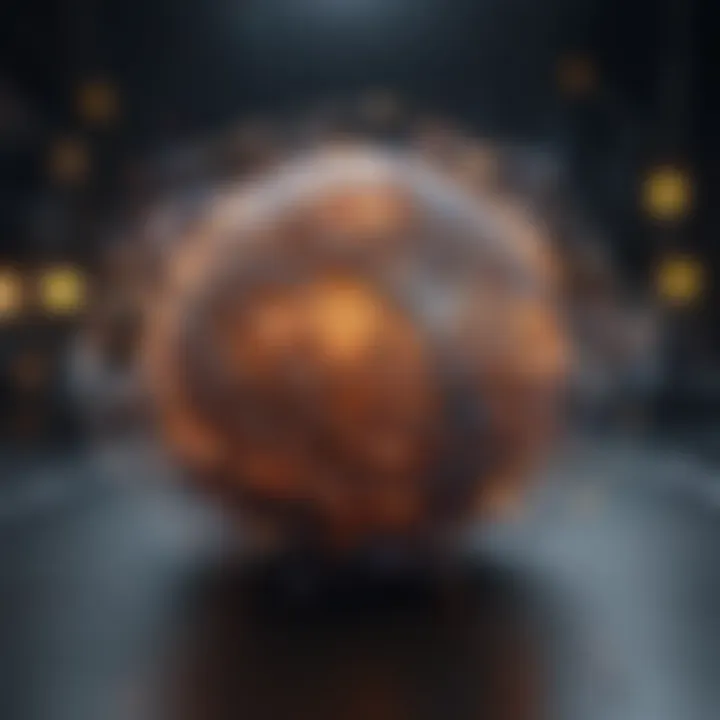
The result is a comprehensive matrix that reflects the shortest paths between all possible vertex pairs. This matrix can then be utilized in various applications, such as transportation networks or communication systems, where understanding all pairs is paramount.
Limitations of the Algorithm
Despite its strengths, the Floyd-Warshall algorithm has limitations worth noting. Its time complexity is O(V^3), where V is the number of vertices in the graph. This cubic time can make it impractical for very large graphs, as the computation increases dramatically with added vertices. Furthermore, the algorithm struggles with memory efficiency since it requires the storage of a complete distance matrix, which can be substantial for large datasets.
An additional consideration is the handling of negative weight cycles. While the algorithm can detect such cycles, paths influenced by them do not yield meaningful results, as they can reduce the path cost indefinitely. As such, any application involving graphs with negative weight edges needs a careful assessment of potential cycle formations before employing the Floyd-Warshall algorithm.
"The flexibility of the Floyd-Warshall algorithm makes it a useful tool for theoretical and practical applications, but it must be applied thoughtfully, considering its limitations such as time complexity and memory usage."
Applications of Shortest Path Algorithms
Shortest path algorithms are pivotal in various real-world applications. Their ability to determine the most efficient route between points is highly sought after. This section will explore how these algorithms function in different contexts, emphasizing transportation networks, network routing, and game development.
Transportation Networks
In the realm of transportation, shortest path algorithms optimize routes for vehicles. The importance of these algorithms cannot be overstated. They help in analyzing traffic flow, determining bus routes, or even assisting in ride-sharing applications such as Uber. For instance, Google Maps utilizes Dijkstra's algorithm to present users with the quickest routes available.
Key benefits include:
- Time Efficiency: Reduced travel time due to optimal routing.
- Cost Savings: Lower fuel consumption when taking the shortest paths.
- Environmental Impact: Minimized emissions through shorter journeys.
Considerations also arise, particularly in complex urban environments. Traffic conditions can change rapidly. Algorithms must adapt to real-time data to remain reliable. The integration of live data feeds into routing systems exemplifies a forward-thinking approach to this challenge.
Network Routing
In computer networks, shortest path algorithms are critical for data packets traveling between nodes. They ensure efficient data transfer in networks, such as the internet. Common algorithms like Bellman-Ford and Dijkstra's help routers determine optimal paths for data. This impacts user experience significantly, affecting load times and streaming capabilities.
Essential elements include:
- Load Balancing: Distributing data across multiple paths to avoid congestion.
- Fault Tolerance: Rerouting during link failures, maintaining connectivity.
- Performance Monitoring: Adjusting paths based on traffic conditions for better efficiency.
As networks expand, challenges like scale and dynamic changes must be addressed. Implementing real-time monitoring tools can enhance routing efficiency, demonstrating the interplay between algorithms and technology.
Game Development
Shortest path algorithms play a vital role in game development, particularly for artificial intelligence in gaming. They enable non-player characters (NPCs) to navigate environments effectively. Whether it's a racing game or an open-world adventure, these algorithms guide NPC movements, making gameplay more realistic.
Incorporating algorithms like A* helps:
- Path Planning: NPCs can find their way around obstacles, enhancing strategic gameplay.
- Dynamic Interactions: The game can adapt paths based on player actions, creating a more immersive experience.
- Resource Management: Optimizing player routes ensures efficient use of in-game resources.
Moreover, the complexity of game worlds presents unique challenges. The algorithms must balance performance and accuracy to ensure smooth gameplay.
In summary, understanding the applications of shortest path algorithms not only highlights their significance but also demonstrates their versatility across different industries. The intricate balance of maintaining efficiency while adapting to real-time changes defines the evolution of these algorithms.
Limitations and Edge Cases
Understanding the limitations and edge cases of shortest path algorithms is crucial for their effective application. An algorithm may perform well under certain conditions but falter in others. Recognizing these boundaries allows researchers and practitioners to make informed decisions when selecting or implementing an algorithm.
Negative Weight Edges
Negative weight edges introduce complexities in shortest path calculations. These edges can lead to situations where the overall shortest path becomes counterintuitive. For example, if one edge has a negative weight and connects two nodes, it might create opportunities to lower the total path cost if visited multiple times. Such characteristics render some algorithms, like Dijkstra's, ineffective, as they do not account for negative weights. Only algorithms such as Bellman-Ford can handle such cases adequately.
The main challenges with negative weight edges include:
- Infinite loops: When cycles involve negative weights, it can lead to an endless process in pathfinding.
- Ambiguity in results: The presence of negative weights can result in multiple shortest paths, complicating the analysis.
- Increased computational complexity: The algorithm may require additional checks to manage the intricacies introduced by these edges.
Applying shortest path algorithms in graphs with negative weight edges necessitates caution and a thorough understanding of the expected outcomes and potential pitfalls.
Large Scale Graphs
Another significant limitation arises with large scale graphs. As the number of nodes and edges increases, the computational resources required to execute shortest path algorithms can grow exponentially. Algorithms that are efficient with smaller datasets may become prohibitive in terms of time and space when scaled up.
Key considerations for dealing with large scale graphs include:
- Memory constraints: Storing graph representations like adjacency matrices can consume vast amounts of memory in large graphs, leading to inefficient use of resources.
- Computational load: Each algorithm has its time complexity, which can be exacerbated by the sheer volume of data, resulting in high processing times.
- Distribution of data: In massive networks, data might be spread across regions, necessitating distributed systems to handle computations effectively.
Researchers are continually developing strategies to mitigate these challenges. Techniques such as graph partitioning or approximation algorithms help reduce the burden when working with large scale graphs. Overall, understanding these limitations is essential for optimizing graph shortest path algorithms in real-world applications.
Future Trends in Shortest Path Research
The domain of shortest path algorithms is evolving rapidly, guided by innovations in technology and shifts in data handling practices. Understanding these trends is essential for practitioners and researchers alike. This section will outline significant developments in the integration of machine learning and real-time data utilization.
Machine Learning Integration
The convergence of machine learning and shortest path algorithms presents a groundbreaking approach to traditional methods. Machine learning can enhance the efficiency of pathfinding algorithms by recognizing patterns in large datasets. This integration allows for more adaptive algorithms that can learn from previous computations, improving their accuracy over time.
Incorporating machine learning into these algorithms often involves neural networks or reinforcement learning. These methods can analyze vast amounts of graph data, identifying optimal paths more effectively than classical approaches. The implications are noteworthy:
- Reduction of Computational Cost: Machine learning models can predict paths faster than many traditional algorithms, particularly in dynamic environments where graphs continually change.
- Enhanced Accuracy: Models trained on historical data can mitigate errors associated with incomplete datasets.
- Flexibility in Application: Machine learning provides the capacity to adapt algorithms for specific use cases, such as urban traffic systems or logistics networks.
Research continues to explore the potential of these integrations, making this area fertile ground for future advancements.
Real-Time Data Utilization
The capacity to process real-time data is revolutionizing shortest path algorithms. In applications where conditions change rapidly, such as traffic management or emergency routing, real-time data can significantly enhance decision-making.
The key benefits of utilizing real-time data include:
- Dynamic Updates: Algorithms can adjust as new information comes in, allowing paths to be recalculated promptly in response to detours or delays.
- Improved User Experience: For applications like GPS navigation systems, users benefit from the accurate forecasting of arrival times, adjusting routes based on current traffic conditions.
- Integration with IoT: The Internet of Things provides a constant flow of data from various sensors, enhancing the granularity of data available for pathfinding algorithms.
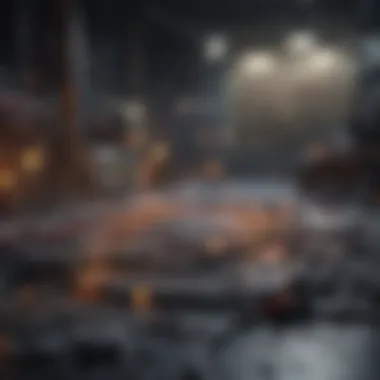
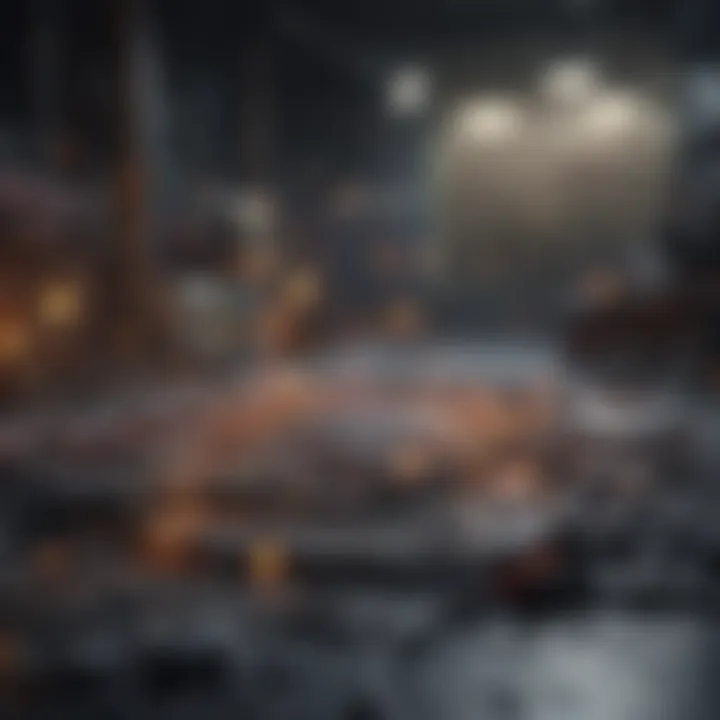
Researchers are actively exploring how to best leverage real-time data with shortest path algorithms, optimizing performance while tackling challenges such as data integration and latency. As the computational landscape shifts, these capabilities will likely define the next generation of shortest path research.
"The fusion of machine learning and real-time data is not merely a trend; it's a transformation of how we approach and implement shortest path algorithms."
The ongoing evolution in these areas emphasizes agility and precision, promising to reshape the efficiency and functionality of algorithmic solutions across sectors. Institutions and industries should pay attention to these trends as they promise substantial enhancements.
Case Studies
The exploration of case studies in graph shortest path algorithms serves as a pivotal component within this article. It reveals real-world applications that highlight the significance and adaptability of these algorithms in various sectors. Case studies allow one to observe the practical impacts and limitations of theoretical models and conclusions. Furthermore, they offer insights into optimizations and enhancements derived from actual challenges faced during implementation.
Urban Traffic Management
Urban traffic management stands as one of the most pressing applications of graph shortest path algorithms. With increasing populations in urban areas, the need for efficient traffic flow becomes paramount. Using algorithms like Dijkstra's or A*, city planners can model traffic systems as graphs where intersections represent nodes and roads signify edges. By calculating the shortest paths in real-time, authorities can optimize traffic light patterns, suggest alternative routes to drivers, and reduce congestion effectively.
Implementing these algorithms provides several benefits:
- Real-Time Adjustments: Ability to adapt quickly to changing conditions, such as accidents or road constructions.
- Data Integration: Utilization of data from sensors and GPS systems enhances the accuracy of route predictions.
- User-Friendly Navigation: Traffic apps like Google Maps leverage these algorithms, assisting users in finding optimal paths.
Nevertheless, challenges arise. Factors such as varying traffic speeds, fluctuating road conditions, and the presence of pedestrians complicate accurate pathfinding, necessitating continual refinement of algorithms.
Logistics and Supply Chain Optimization
In the context of logistics and supply chain management, graph shortest path algorithms are crucial for optimizing routes and improving efficiency. Companies strive to minimize costs while maximizing delivery speed. By using algorithms, businesses can map distribution networks, allowing them to identify the most efficient paths for transportation.
These algorithms facilitate several considerations that are important:
- Cost Efficiency: They help in reducing operational costs through optimized routing.
- Delivery Times: Shortest path algorithms enable companies to promise faster delivery to customers, improving service quality.
- Resource Allocation: Businesses can make informed decisions regarding vehicle deployment and fleet management based on analyzed data.
In summary, case studies on urban traffic management and logistics showcase the real-world implications of graph shortest path algorithms. They exemplify how these algorithms provide significant improvements in efficiency and utility across various domains.
Comparison of Shortest Path Algorithms
The comparison of shortest path algorithms is critical for effectively understanding their strengths and weaknesses across various applications. Each algorithm has its unique approach, performance metrics, and use cases. By evaluating these factors, it becomes easier to determine the ideal algorithm for specific situations. This section aims to identify the key parameters that warrant comparison, such as efficiency, robustness, adaptability, and suitability for different types of graphs.
When addressing the efficiency of algorithms, we often consider their time and space complexity. An efficient algorithm can process large datasets while minimizing resource use. For instance, Dijkstra's algorithm is known for its applicability to positive weight graphs but struggles with graphs containing negative weights. On the other hand, the Bellman-Ford algorithm tackles negative weights effectively but at the expense of additional computational time.
Furthermore, it is essential to assess how algorithms perform in dynamic environments where graphs regularly change. Algorithms like A* demonstrate adaptability by incorporating heuristics, making them efficient in specific contexts such as gaming and navigation systems. Meanwhile, Floyd-Warshall provides a thorough solution for all pairs of shortest paths, which can be overkill for sparse graphs but is useful in dense networks.
In summary, a comprehensive comparison not only reveals the optimal conditions for each algorithm but also guides users in selecting or developing hybrid solutions that leverage the best features of multiple approaches.
Efficiency Evaluation
Efficiency evaluation involves measuring the performance of various algorithms in terms of their time and space complexities. This assessment is crucial for anyone aiming to apply these algorithms in practical scenarios. For example, when implementing Dijkstra's algorithm, the primary considerations include:
- Time Complexity: The algorithm has a complexity of O((V + E) log V) when using a priority queue, where V is the number of vertices and E denotes the number of edges.
- Space Complexity: The space complexity is O(V) owing to the storage of distances and previous nodes.
In contrast, the Bellman-Ford algorithm has a time complexity of O(VE) and is primarily used when negative edge weights are present. However, it does come with a space complexity of O(V) similar to Dijkstra.
The A* algorithm utilizes heuristics, which can appear misleading when assessing its efficiency. Its time complexity can vary significantly based on the chosen heuristic, sometimes resulting in exponential performance related to node expansion. Therefore, a well-designed heuristic can substantially improve efficiency.
Use Case Scenarios
When discussing use case scenarios, one can categorize algorithms based on their strength and application fit. Here are some notable scenarios:
- Dijkstra's Algorithm: Optimal for routing in network communication and logistics where all edge weights are positive. It suits real-time applications like GPS navigation but isnโt viable for graphs with negative weights.
- Bellman-Ford Algorithm: Often utilized in finance and networking where negative cycles might occur, such as arbitrage detection in currency markets.
- A Search Algorithm:* Famous for its use in video games for character navigation, this algorithm benefits from heuristic evaluations, prioritizing paths based on estimated costs.
- Floyd-Warshall Algorithm: Ideal for scenarios requiring all-pair shortest paths, often employed in large scale network analysis and road planning where comprehensive calculations are necessary.
These examples illustrate the varying strengths of each algorithm. By matching the tasks at hand with corresponding algorithmic capabilities, practitioners can enhance overall effectiveness in their implementations.
Practical Implementation
The successful implementation of graph shortest path algorithms is pivotal in transforming theoretical concepts into applicable solutions in real-world scenarios. Practical implementation involves not just the algorithmic execution but also how one integrates these algorithms into existing systems or workflows. This section explores key components, benefits, and considerations when implementing shortest-path algorithms, ensuring users can make informed decisions in various contexts.
Choosing the Right Algorithm
When it comes to selecting the most suitable shortest path algorithm, several factors should be assessed. Different algorithms boast unique strengths and weaknesses, making the selection process essential for optimal performance.
- Graph Characteristics: Determine whether the graph is directed or undirected, weighted or unweighted. For instance, Dijkstraโs algorithm is efficient for graphs without negative weights, while the Bellman-Ford algorithm handles graphs with negative weights.
- Performance Requirements: Assess the speed and resource consumption required for your specific application. A* search is fantastic for pathfinding where heuristics can greatly improve efficiency.
- Use Case Scenarios: Consider the actual application, such as routing in telecommunication networks versus pathfinding in video games. Each scenario can strongly influence which algorithm will yield the best results.
Each project is unique. Therefore, careful evaluation of these factors will help align your choice with your projectโs specific needs.
Code Examples
To better understand the practical application of these algorithms, here are simple code snippets for Dijkstra's algorithm in Python. It utilizes an adjacency list representation of the graph.
This code outlines the algorithm's core functionality, allowing users to understand how to compute shortest paths effectively. By adapting these concepts within larger projects, significant improvements in operation can be achieved.
The emphasis on practical implementation will not only enhance algorithm comprehension but also facilitate real-world applications.
Finale
Graph shortest path algorithms have become a cornerstone in the realm of data science and computer science. They offer efficient solutions for determining the quickest route between nodes in a graph. This article has surveyed critical techniques, advantages, and limitations of various algorithms, including Dijkstra's Algorithm, Bellman-Ford, A* Search, and Floyd-Warshall. The insights provided emphasize the importance of selecting the appropriate algorithm based on specific needs.
The significance of this conclusion lies in synthesizing the information presented throughout the article. Each algorithm serves distinct purposes supported by unique strengths. Therefore, understanding these differences can aid in optimizing pathways in real-world applications such as transportation, logistics, and network routing. Moreover, as technology evolves, further research and innovation in this area continue to emerge, expanding their applications beyond traditional uses.
Utilizing these algorithms lays a foundation for tackling complex problems, thereby improving decision-making processes in various fields. Choosing the right algorithm can save substantial time and resources, benefiting industries where efficiency is crucial. This article aims to equip readers with a comprehensive understanding to approach graph-related challenges logically and effectively.
Key Takeaways
- Shortest path algorithms are essential tools for various applications in data-driven fields.
- Each algorithm possesses specific characteristics that cater to particular problems and scenarios.
- The appropriate selection of an algorithm based on context can enhance efficiency and outcomes in practical applications.
- Ongoing developments in algorithmic approaches integrate modern technology, signaling a vibrant future for the field.
Looking Ahead
As we move forward, the integration of machine learning and artificial intelligence in graph shortest path algorithms shows promising potential. Researchers are exploring adaptable algorithms that can learn from data patterns, improving accuracy over time. Moreover, real-time data utilization will transform how decisions are made in dynamic environments like transportation systems and online mapping services. These advancements will continue to shape the future landscape of shortest path analysis, offering richer and more nuanced insights for professionals and researchers alike.
In summary, the realm of shortest path algorithms presents valuable opportunities for enhancing various applications. Embracing these techniques can yield significant benefits while paving the way for innovative research and development.