Essential Mathematical Foundations for Computer Science
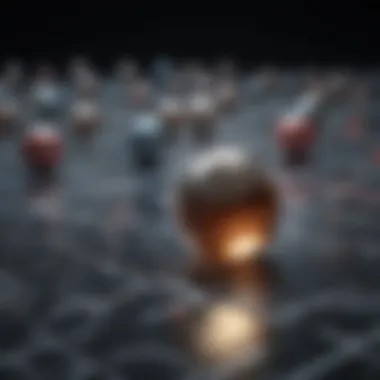
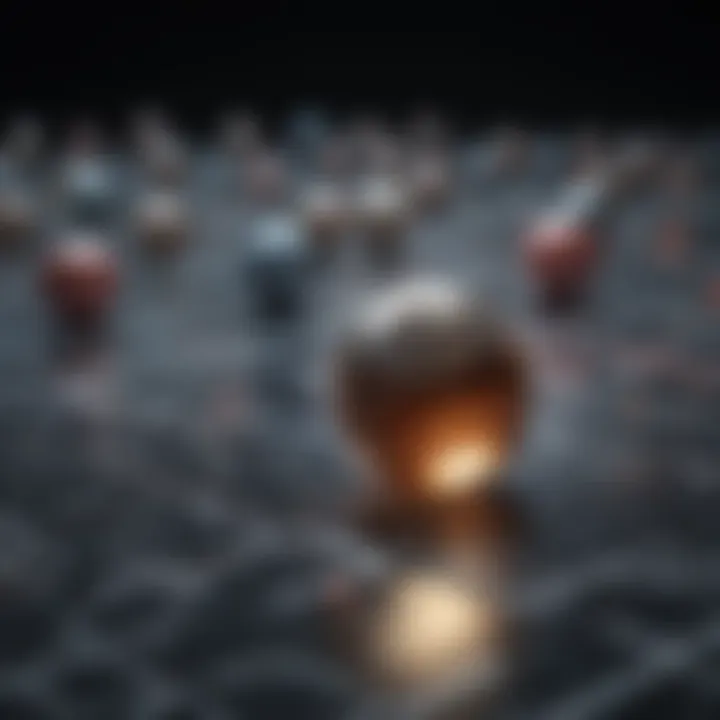
Intro
Mathematics is a cornerstone of computer science. Understanding various mathematical concepts enhances programming skills and logical reasoning. This article will guide readers through essential topics such as discrete mathematics, logic, algebra, and statistics. Each section aims to provide clarity and practical significance as applied to real-world computer science challenges.
Key Research Findings
Overview of Recent Discoveries
Research shows that mathematical theories directly impact computational efficiency. Advances in algorithms often stem from improved mathematical techniques. For instance, graph theory plays an essential role in networks and data structures, influencing data retrieval and storage methods. Recent studies in numerical methods optimize calculations, reducing processing time significantly, which is paramount in large data analyses.
Significance of Findings in the Field
The ongoing discoveries in the realm of mathematics are crucial for several reasons:
- Algorithm development: Mathematical foundations enable developers to design efficient algorithms.
- Data structures: Understanding mathematical principles aids in creating robust data structures, essential for effective programming.
- Problem-solving: Advanced mathematical logic fosters better problem-solving approaches, allowing programmers to tackle complex issues more effectively.
"Mathematics is the language with which God has written the universe." - Galileo Galilei
Breakdown of Complex Concepts
Simplification of Advanced Theories
Many often view certain mathematical concepts as daunting. However, breaking them down into simpler terms can help grasp their real utility. For example, discrete mathematics encompasses various areas like set theory, combinatorics, and graph theory. Mastering these subjects empowers students to develop algorithms that manage data more effectively.
Visual Aids and Infographics
Supplementing text with visual aids can enhance understanding. Flowcharts and diagrams depicting algorithms or data structures can clarify complex ideas. For example, illustrating a binary tree structure can demonstrate how data is organized efficiently. Utilizing online resources such as Wikipedia and Britannica can provide additional context and visualization to reinforce learning.
End
Building a strong foundation in mathematics is essential for anyone pursuing a career in computer science. The concepts explored, such as discrete mathematics, logic, algebra, and statistics, are crucial for effective problem-solving and computational thinking. Future sections will delve deeper into each topic, providing tools and knowledge necessary for success in the field.
Foreword to Mathematics in Computer Science
Mathematics serves as the backbone of computer science. This is especially true in areas such as algorithms, programming, and computational theory. In this section, we will dissect how mathematical principles are interwoven with the core elements of computer science. By gaining a solid understanding of these mathematical foundations, students and professionals can develop enhanced problem-solving skills that are critical in the field.
Mathematical concepts not only enable the understanding of data structures and algorithms but they also illuminate the pathways for optimizing computational tasks. When one considers the complexity of computer systems, it becomes clear why a strong grasp of mathematical principles is necessary. The logical reasoning developed through mathematics translates directly to efficient program design and debugging.
Furthermore, areas such as discrete mathematics, statistics, and algebra are integral in various applications, ranging from data analysis to network optimization. Understanding these concepts allows computer scientists to create robust and reliable software while simultaneously recognizing the limitations imposed by computational constraints.
"Mathematics is the language in which God has written the universe."
โ Galileo Galilei
The Role of Mathematics in Computing
Mathematics guides the core processes of computing. From the formulation of algorithms to the performance analysis of these algorithms, mathematics provides the tools necessary to evaluate and refine computation strategies. Computational problems are often abstract in nature and require mathematical models to arrive at solutions.
For example, mathematical logic underpins programming languages and their syntax. Without a solid understanding of logical constructs, it is easy to encounter pitfalls in developing efficient algorithms. Mathematical models, such as graph theory, give insight into the connections and interactions between different data points, which can be vital in fields like networking and database design.
Additionally, areas such as cryptography rely heavily on number theory and modular arithmetic, essential for securing data. The necessity for mathematical structures in these contexts illustrates the pervasive role of math in driving innovation in computer science.
Importance of Mathematical Foundations
A foundational understanding of mathematics cannot be overstated for anyone pursuing a career in computer science. Concepts like sets, functions, and relations lay the groundwork for data organization and manipulation. When programmers grasp these foundational principles, they can more easily navigate the complex landscapes of software development.
Moreover, competencies in statistics and probability are crucial for roles involving machine learning and artificial intelligence. The ability to analyze and interpret data accurately influences decision-making processesโnot just in software development, but also in business and scientific research fields.
In summary, mathematics is not simply a skillset for computer scientists; it is a fundamental component that enhances logical reasoning, cultivates analytical abilities, and fosters innovative thinking.
By positioning mathematics centrally in computer science education, students can build a skill set that promotes not only academic success but also professional excellence. Familiarity with these concepts prepares them for real-world challenges and positions them as competent problem solvers.
Fundamental Concepts of Logic
Logic serves as the backbone of computer science. Understanding fundamental concepts of logic is essential for developing effective algorithms and programming methods. This section outlines the significant aspects of logic that every computer scientist should grasp. Propositional Logic and Predicate Logic are two vital components that form the basis of logical reasoning in computing. They offer tools for forming valid arguments and reasoning through complex problems.
One benefit of mastering logic is the ability to enhance problem-solving skills. Logic enables clear thinking and structured analysis. This results in greater efficiency when tackling programming challenges. Moreover, logic has practical applications in various areas such as artificial intelligence, database theory, and software engineering.
Propositional Logic
Propositional logic deals with propositions, which are statements that can either be true or false. Each proposition stands alone, leading to a variety of logical operations. The basic propositions are often represented using symbols, such as P and Q. The main benefit of understanding propositional logic is its simplicity. You can quickly evaluate logical statements and their relationships.
Some core elements include:
- Logical Connectives: These are symbols used to connect propositions. Examples include AND, OR, NOT, and IMPLIES.
- Truth Values: Each proposition is assigned a truth value, either true (T) or false (F). This allows for straightforward evaluation of statements.
For example, consider the proposition "P AND Q." This statement is only true when both P and Q are true.
Predicate Logic
Predicate logic extends propositional logic. It introduces quantifiers and predicates, allowing for more complex statements. Predicates have variables that can represent different objects. This ability enables more nuanced expressions of relationships and conditions.
Key features of predicate logic include:
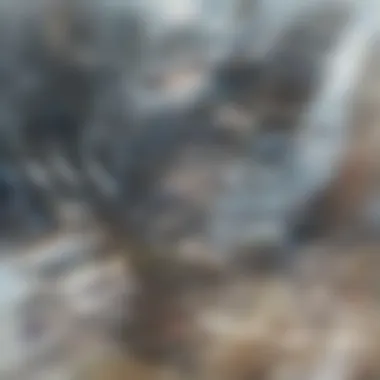
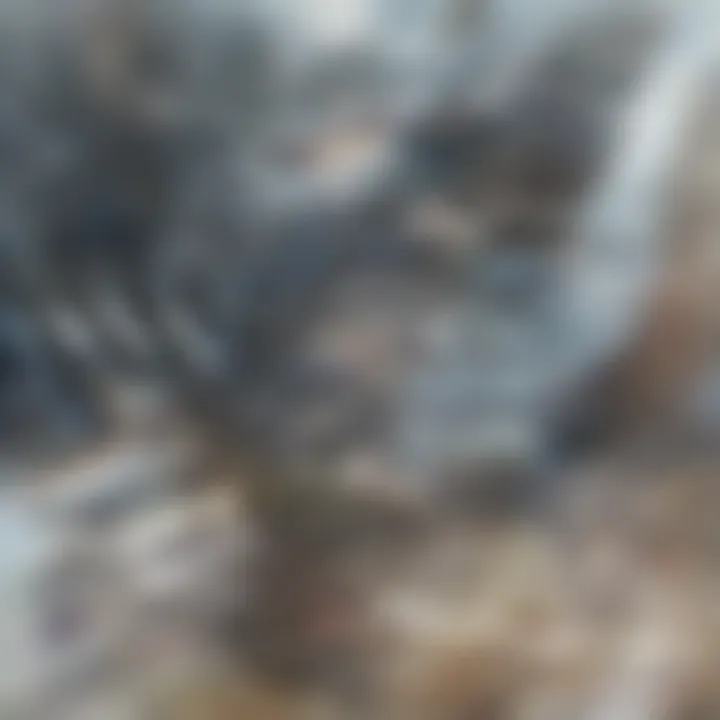
- Universal Quantifier (โ): This symbol indicates that a predicate holds for all instances of a variable.
- Existential Quantifier (โ): This symbol indicates that there exists at least one instance for which the predicate is true.
Understanding predicate logic is vital for database management and artificial intelligence applications. It provides the framework for reasoning about collections of objects and their relationships, essential for logical deductions.
Logical Operators and Truth Tables
Logical operators play an important role in evaluating logical expressions. The three main logical operators include AND, OR, and NOT. Each operator combines propositions in a specific manner, leading to new truth values.
Truth tables are used to illustrate how these operators work. A truth table lists all possible combinations of truth values for given propositions. For example, the truth table for the AND operator looks like this:
| P | Q | P AND Q | | T | T | T | | T | F | F | | F | T | F | | F | F | F |
By using truth tables, one can systematically analyze complex logical expressions. This method is especially useful in verifying the validity of logical arguments.
Understanding fundamental logic concepts enhances critical thinking and problem-solving abilities. It lays the groundwork for advanced topics in computer science.
Discrete Mathematics Overview
Discrete mathematics plays a crucial role in computer science. Unlike continuous mathematics, which deals with real numbers and smooth functions, discrete mathematics involves distinct and separate values. The principles of discrete mathematics underpin many computing concepts, from algorithms to data structures, making it essential for anyone working in the field.
The fundamental elements of discrete mathematics help in understanding complex problems in computing. This includes topics like sets, relations, functions, and combinatorics. These concepts provide a structured way to analyze discrete elements and their interactions, which is vital when designing efficient algorithms and systems.
Sets and Functions
Sets are collections of objects. They can be finite or infinite and are a foundational notion in discrete mathematics. A set can contain any type of elements, such as numbers, letters, or even other sets. Understanding sets helps in organizing data and determining relationships between different elements.
Functions are mappings between sets. They assign each element of one set to exactly one element of another set. This relationship is fundamental to computing, as it allows for the processing of inputs and outputs within algorithms. When examining functions, one often discusses domain and range, encapsulating the inputs and potential outputs.
For example, the function maps real numbers to their squares. Hereโs a brief look at the properties of functions:
- Injective (one-to-one): Every element of the output is unique.
- Surjective (onto): Every element of the target set is covered by the function.
- Bijective: The function is both injective and surjective.
Relations and Their Properties
Relations are another pivotal element in discrete mathematics. They describe a relationship between two sets. A relation can be thought of as a subset of the Cartesian product of those sets. Analyzing relations involves examining their properties such as reflexivity, symmetry, and transitivity.
For instance, if we have sets A and B, a relation R could be defined as a set of ordered pairs where the first element is from A and the second element is from B. Implementing relations correctly in databases can lead to optimized queries and better data integrity.
Important Note: Understanding relations can significantly enhance your ability to manipulate databases and make informed decisions regarding data retrieval.
Combinatorics and Counting Techniques
Combinatorics focuses on counting, arrangement, and combination of objects. It is essential for analyzing algorithms and optimizing solutions in computer science. Techniques from combinatorics allow us to understand the possible configurations of a data set.
Examples of counting techniques include:
- Permutations: Different arrangements of a set of items.
- Combinations: Selection of items where order does not matter.
Combinatorial analysis aids in algorithm design, especially in determining the most efficient paths or solutions in problems such as network design and resource allocation.
In summary, discrete mathematics forms a backbone for understanding many concepts in computer science. Each subtopic, from sets to combinatorics, provides tools and frameworks necessary for effective computational thinking.
Number Theory and Its Applications
Number theory is a vital branch of mathematics that deals with the properties and relationships of numbers, particularly integers. Its significance in the context of computer science is profound. It enables us to approach computational problems with a refined analytical perspective, especially in areas like cryptography and algorithms. By developing a solid understanding of number theory, one can improve problem-solving skills and gain insight into the behavior of algorithms.
Key elements of number theory include prime numbers, factorization, and modular arithmetic. These concepts form the foundation of many algorithms used in computer science, particularly in cryptography.
Prime Numbers and Factorization
Prime numbers are integers greater than 1 that have no divisors other than 1 and themselves. Their fundamental nature makes them pivotal in numerous mathematical applications. In computer science, prime numbers are crucial for cryptographic systems. For instance, the security of public-key algorithms, such as RSA, is predicated on the difficulty of factoring large numbers into their prime components.
The challenge of factorization becomes increasingly complex as the numbers grow larger. Efficient algorithms for prime factorization could undermine the security of cryptographic systems. This forms a key aspect of studying prime numbers: understanding their distribution and the methods used for factoring.
Modular Arithmetic
Modular arithmetic is a system of arithmetic for integers, where numbers wrap around after reaching a certain value, known as the modulus. This arithmetic underpins many algorithms in computer science, especially in the fields of cryptography and algorithm design. It simplifies operations and helps to manage large numbers that arise in calculations.
For example, when encrypting messages, modular arithmetic allows for calculations to keep numbers manageable and provides a way to avoid overflow errors. The properties of modular arithmetic, including congruences and the Chinese Remainder Theorem, are essential tools in algorithm development and analysis.
Cryptography and Security
Cryptography is the method of securing information by transforming it into an unreadable format. Number theory plays a central role here. It forms the base of various encryption techniques vital for secure communication in our digital age. The RSA system, as mentioned earlier, relies heavily on the properties of prime numbers and modular arithmetic.
This interplay between number theory and cryptography illustrates how mathematical principles can secure sensitive data. However, with the growing power of computers, the need for more robust encryption methods is clear. Understanding number theory is therefore crucial for both developing new methods and assessing existing security measures.
"The study of number theory is not just a theological exercise; it has real applications in securing our digital lives."
In summary, number theory is not just an abstract field but a cornerstone of modern computing. Its applications in cryptography, coupled with the importance of understanding prime numbers and modular arithmetic, underline its necessity in the broader scope of computer science.
Algebra in Computer Science
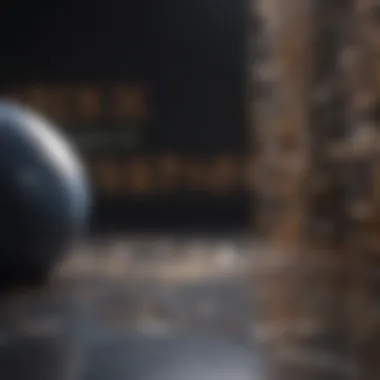
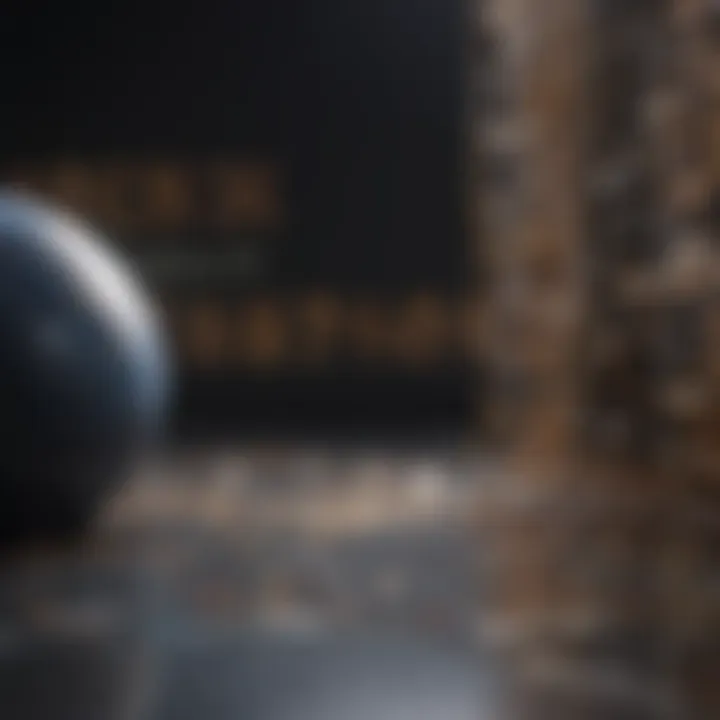
Algebra serves a fundamental role in computer science. It provides tools for problem solving and helps in the formulation of algorithms. Understanding algebraic concepts is crucial for programming and for tasks like data processing and algorithm optimization. Algebra not only form the basis of the mathematical techniques used in computing but it also facilitates clearer thinking when dealing with complex systems.
Linear Algebra Basics
Linear algebra focuses on vector spaces and the linear transformations between them. It allows us to work in multi-dimensional spaces, crucial for computer graphics, machine learning, and data science. Concepts such as vectors, matrices, and eigenvalues come into play here.
Variables and equations can model real-world relationships, making them essential for simulations and modeling. Moreover, systems of linear equations can be solved using algebraic methods, contributing to data analysis.
Matrices and Their Applications
Matrices are arrays of numbers or functions arranged in rows and columns. They are used extensively in computer science for various applications, including graphics transformations and representing graphs. In image processing, for instance, matrices allow for manipulation of pixel data.
The multiplication of matrices facilitates operations like rotating, scaling, and translating images.
- In machine learning, matrices are used to organize datasets.
- Networks can be represented as adjacency matrices to simplify understanding of connections and paths.
In summary, matrices help convert complex calculations into manageable forms.
Vector Spaces and Transformations
Vector spaces offer a framework for analyzing linear combinations and transformations. A vector space consists of vectors which can be scaled and added together satisfying certain axioms.
Transformations within this space can represent operations such as rotation, scaling, or translation of objects in a computational framework. For example,
This function scales a given vector.
Additionally, understanding vector spaces is important for algorithms in data science. This is evident in how machine learning techniques leverage concepts such as Principal Component Analysis, which uses vectors to reduce data dimensionality.
Understanding these algebraic concepts provides a robust foundation for algorithm development. This aids in tackling challenges found in areas like data analysis, artificial intelligence, and network optimization. By gaining proficiency in algebra, one can develop better programming skills that are adaptable to various computational tasks.
Algorithm Analysis and Mathematical Models
In computer science, the efficiency of algorithms directly impacts the performance of applications and systems. Algorithm Analysis and Mathematical Models form a core component of understanding how algorithms operate in terms of time and space complexity. This section elucidates the significance of analyzing algorithms, providing insight into how mathematic principles apply to computational problems.
Understanding algorithm efficiency allows programmers and developers to make informed choices when selecting or designing algorithms. The analysis includes examining the algorithm's resource consumption, which helps address practical considerations, such as processing time and memory usage.
Both Big O notation and complexity classes are central in this analysis. They provide a framework for assessing algorithm performance regardless of the hardware or software environment. By employing these models, one can predict how an algorithm will perform as the size of the input grows. This prediction is essential for scalability in software development.
Furthermore, employing mathematical induction, we can establish the correctness of algorithms and their efficiency. Analysis helps in laying down a strong foundation for designing algorithms that are not only effective but also optimal.
Big O Notation and Complexity Classes
Big O notation is a mathematical representation that describes the upper bound of an algorithm's running time or space requirements. This notation becomes fundamental when determining how the performance of algorithms changes with varying input sizes.
- Constant time (O(1)): The algorithm's performance remains constant, regardless of input size.
- Logarithmic time (O(log n)): The performance grows logarithmically in relation to the input size.
- Linear time (O(n)): The algorithm's time increases linearly with the input size.
- Quadratic time (O(n^2)): Performance is proportional to the square of the input size.
- Exponential time (O(2^n)): The time grows exponentially relative to the input size; impractical for large inputs.
Understanding these categories assists programmers in selecting the right algorithm based on performance needs. For example, merge sort operates in O(n log n) time, making it suitable for large datasets, whereas bubble sort operates in O(n^2) time. The choice of algorithm can thereby be critical based on the expected input size and desired efficiency.
Mathematical Induction
Mathematical induction is a technique used to prove the correctness of algorithms. It involves two steps: the base case and the inductive step.
- Base Case: Verify that the algorithm works for a specific initial case.
- Inductive Step: Assume the algorithm works for a certain case and then show it holds for the next case.
This powerful method establishes validity for all natural numbers or for all elements of a specified set. Algorithm correctness is paramount when developing software, particularly in critical systems such as banking or healthcare, where failures can result in significant errors or losses.
Recursion and Recursive Algorithms
Recursion involves a function calling itself to solve smaller instances of the same problem. Recursive algorithms can often be simpler to implement and understand compared to their iterative counterparts. However, they come with considerations of efficiency and optimization.
Some key points about recursion include:
- Each recursive call adds a new layer to the call stack, consuming memory.
- If not properly managed, recursion can lead to stack overflow errors.
- It is essential to define a base case to terminate the recursive calls to prevent infinite loops.
A common example of a recursive algorithm is the factorial function, defined mathematically as ( n! = n \times (n - 1)! ) with a base case of ( 0! = 1 ).
In summary, understanding Algorithm Analysis and Mathematical Models provides vital tools for computer scientists and educators. This knowledge aids in creating efficient algorithms and verifies their correctness through logical methods, ensuring robust software development.
Statistics and Data Analysis
Statistics and data analysis serve crucial roles in computer science. They provide methods to interpret complex data sets, reveal insightful patterns, and guide decision-making. Students and professionals in the field often employ statistics to validate their algorithms and ensure the reliability of their software. The ability to analyze data statistically helps in extracting meaningful information, which, in turn, assists in addressing problems more effectively.
Understanding statistics equips individuals with tools to summarize data efficiently. Skills in statistics are not just valuable in theoretical contexts, but also in applied settings where real-world data influences software development, system design, and user experience.
Descriptive Statistics Basics
Descriptive statistics refer to statistical methods used to summarize and describe the main features of a data set. This aspect of statistics plays a fundamental role in computer science because it provides a way to easily understand large volumes of data.
Key Components of Descriptive Statistics:
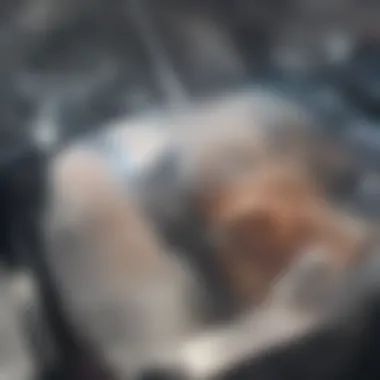
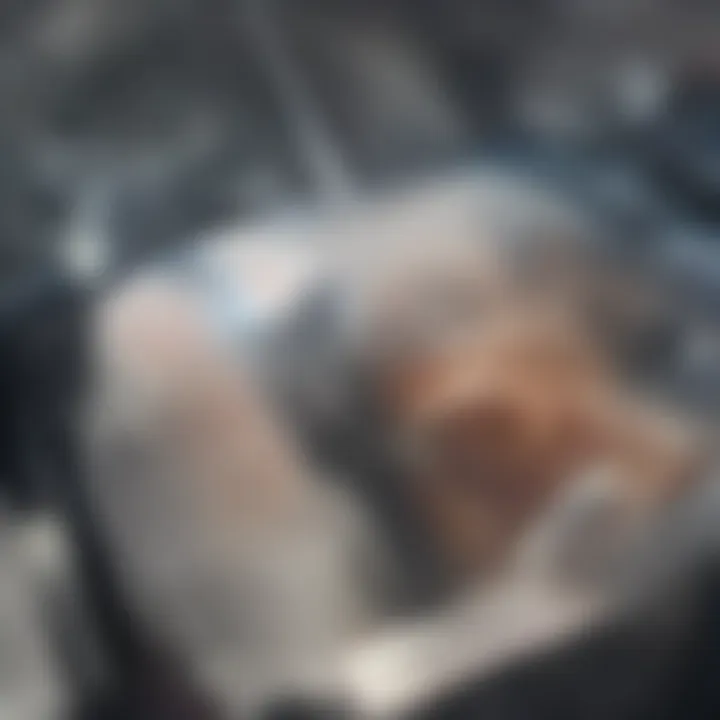
- Measures of Central Tendency: Mean, median, and mode are significant as they give an overview of the data distribution.
- Measures of Variability: Range, variance, and standard deviation inform on how much data values deviate from the average.
- Data Visualization: Tools such as histograms, bar graphs, and pie charts help in effectively communicating data insights.
Utilizing descriptive statistics allows computer scientists to quickly assess data properties and make informed preliminary analyses before delving deeper.
Probability Theory Fundamentals
Probability theory is essential for understanding uncertainty and risk in analytical processes. In computer science, it lays the groundwork for algorithms and models that deal with chance, such as in machine learning.
Core Concepts in Probability:
- Events and Sample Space: An event is a particular outcome, while the sample space refers to all possible outcomes.
- Probability Distributions: This includes normal distribution, binomial distribution, and Poisson distribution, which describe how probabilities are distributed across outcomes.
- Bayes' Theorem: It is a pivotal concept that allows for updating probabilities based on new evidence, which is particularly useful in artificial intelligence.
By comprehending these probability elements, professionals can enhance their predictive modeling techniques and improve the overall robustness of their applications.
Statistical Inference and Applications
Statistical inference involves making predictions or generalizations about a population based on a sample. This is especially vital in computer science when working with data sets where it is impractical or impossible to collect data from an entire population.
Important Aspects of Statistical Inference:
- Confidence Intervals: These provide a range of values that likely contain the true parameter.
- Hypothesis Testing: This process helps in making decisions or inferences based on sample data. Researchers often test assumptions about their algorithms or models.
- Applications in Machine Learning: Inference techniques are widely applied in machine learning to evaluate models and ensure their accuracy against unseen data.
Utilizing statistical inference methods allows for more accurate, reliable, and efficient analyses, which is often the difference between success or failure in processing tasks.
Statistical knowledge is vital for successful data analysis in computer science, allowing every decision to be backed by solid empirical evidence.
Graph Theory in Computer Science
Graph theory offers a rich and significant avenue for exploration within the broader scope of computer science. It provides tools and frameworks to model relationships and interactions between discrete objects. As systems in computing become increasingly connected, understanding the geometrical and algorithmic properties of graphs becomes essential.
This section delves into graph theory essentials, highlighting its relevance not just in theory but also in practical applications. We will review foundational definitions, explore some prevalent algorithms, and examine real-world applications. Ultimately, a grasp of graph theory equips one to solve complex problems effectively, transforming abstract concepts into tangible insights.
Graphs: Definitions and Properties
A graph is a mathematical structure that consists of vertices and edges. Vertices represent objects, while edges capture the relationships between them. The relationships can be weighted or unweighted, directed or undirected, allowing for a diverse range of configurations.
Some key properties include:
- Degree: The degree of a vertex is the number of edges connected to it. It is a fundamental concept when analyzing graph connectivity.
- Path: A path is a sequence of edges that connect a series of vertices. Paths can vary in length and complexity.
- Connectivity: A graph is connected if there exists a path between every pair of vertices. Understanding connectivity is crucial for network analysis.Graphs can be categorized into different types, including:
- Simple Graphs: No loops or multiple edges.
- Complete Graphs: Every vertex is connected to every other vertex.
- Bipartite Graphs: Vertices can be divided into two distinct sets.
Knowing these definitions and properties is critical, as they serve as the groundwork for more advanced discussions on graph algorithms and applications.
Graph Algorithms: An Overview
Graph algorithms form the backbone of solving problems related to graph structures. Various algorithms can manipulate graphs, leading to solutions for numerous computational challenges. Among the most prominent graph algorithms are:
- Dijkstra's Algorithm: A famous algorithm used to find the shortest path between nodes in a weighted graph.
- Depth-First Search (DFS): An algorithm for traversing or searching tree or graph data structures. It explores as far as possible along each branch before backtracking.
- Breadth-First Search (BFS): This technique explores neighbors layer by layer, making it particularly useful for finding the shortest path in unweighted graphs.
Each of these algorithms has its own use-cases and efficiencies based on graph structure and weight distributions. Proper selection of an algorithm is crucial for optimizing performance in real-world applications.
Applications of Graph Theory
Graph theory is not limited to theoretical studies; its implications are visible in various domains. Here are some fields where graph theory shines:
- Social Networks: Users and their connections can be represented as graphs, where the vertices are users and the edges symbolize connections or interactions.
- Transportation and Logistics: Graphs model routes and point-to-point connections, aiding in route optimization and traffic efficiency.
- Computer Networks: The relationships between nodes in a network can be analyzed using graph-based approaches, helping improve network design and troubleshoot issues.
- Artificial Intelligence: Graph-based algorithms are integral in problems involving pathfinding, such as in game AI strategies.
The study of graphs allows computer scientists to tackle a multitude of real-world challenges, making it a pivotal component of computational thinking.
Ending and Future Directions
The benefits of mastering these mathematical concepts are numerous. First, they lay a strong foundation for learning advanced topics. Concepts such as algorithmic complexity, data structures, and machine learning all rely heavily on the principles outlined in this article. Additionally, they equip professionals with the tools necessary to tackle real-world challenges. Whether it's optimizing algorithms or analyzing big data, the application of mathematical principles is evident.
There are considerations to keep in mind for future learning. Emerging technologies, such as artificial intelligence and quantum computing, rely on a deeper understanding of mathematics. Updating our knowledge base is crucial as these fields evolve. Continuous learning and adaptation to advancements in mathematical applications within computer science are vital for anyone looking to make significant contributions in the field.
"Mathematics is not just about numbers, it's about understanding patterns and relationships that apply to the world around us."
In summary, understanding the mathematical foundations of computer science provides a significant advantage. The synergy between mathematics and computing will only strengthen as technology advances, making it imperative for current and future computer scientists to embrace these concepts.
Summary of Key Concepts
In the course of this article, several key mathematical concepts were central to understanding their role in computer science. Here is a recap of some significant topics:
- Propositional and Predicate Logic: These set the stage for reasoning about algorithms and their correctness.
- Discrete Mathematics: Includes sets, functions, and relations that are foundational in designing and analyzing algorithms.
- Number Theory: Application in cryptography emphasizes the importance of secure communication.
- Linear Algebra: Critical for areas such as computer graphics and machine learning.
- Statistics: Essential for data analysis and making informed decisions based on data.
Each of these concepts interlinks, forming a comprehensive mathematic foundation in computer science that enhances both theoretical understanding and practical application.
The Evolving Role of Mathematics in Computing
The role of mathematics in computer science is continually transforming, shaped by rapid advancements in technology. We have moved from basic algorithms to complex machine learning systems that require an in-depth understanding of mathematical principles.
As computer science evolves, some key aspects to consider include:
- Interdisciplinary Integration: Mathematics is becoming increasingly integrated with other fields, including biology, economics, and engineering.
- Advanced Algorithm Development: New algorithms address large-scale problems, necessitating advanced math to analyze efficiency and performance.
- AI and Machine Learning: These fields demand a rigorous comprehension of statistics and linear algebra for effective implementation.
The continuous growth of mathematics within the context of computing signals a need for adaptability in learning. As we transition to more complex concepts, it is essential for professionals and students to cultivate a strong mathematical intuition alongside technical skills. This adaptability not only fosters innovation but also ensures that the field evolves in a meaningful and impactful way.