Understanding Algorithms: Foundations and Applications
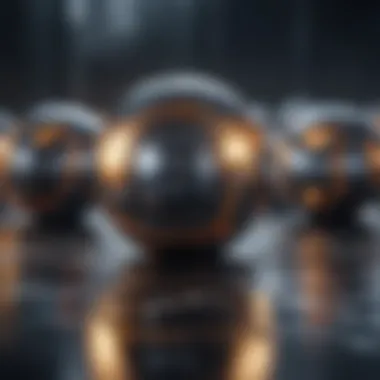
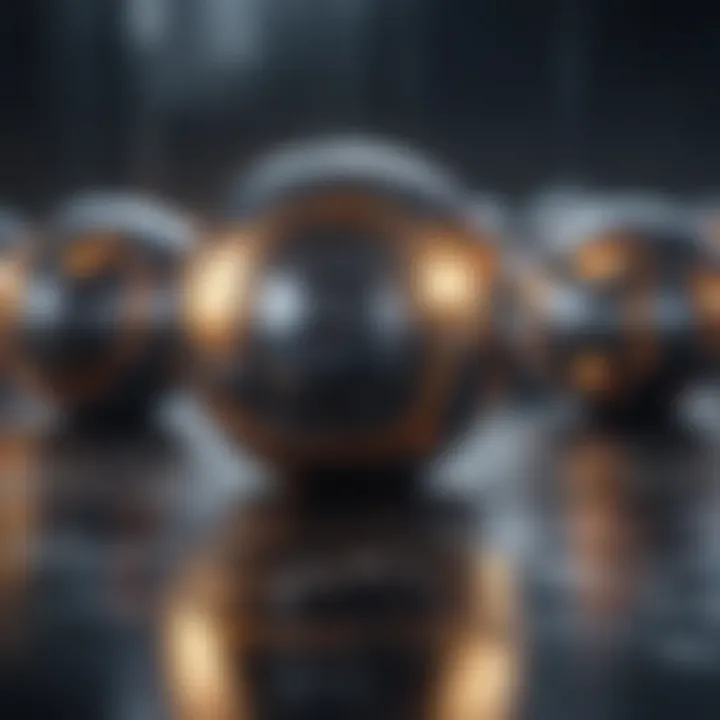
Intro
In the realm of computer science, algorithms serve as the backbone of programming and data processing. They are the systematic procedures to solve problems, manipulating data to achieve desired outcomes. With numerous applications across various domains, understanding algorithms is essential for anyone involved in technology, whether they are students, educators, or seasoned professionals. This section lays the foundation for a deeper exploration into algorithms, addressing key research findings and simplifying complex concepts.
Key Research Findings
Overview of Recent Discoveries
Recent advancements in algorithm research have been pivotal in various fields. They pave the way for improved computational efficiency, data analysis, and artificial intelligence. For instance, breakthroughs in sorting algorithms, like TimSort used in Python, have enhanced performance in real-world applications. Similarly, developments in machine learning algorithms, such as deep learning techniques, have revolutionized data processing and image recognition tasks.
Understanding these trends allows professionals to adapt to evolving technology landscapes.
Significance of Findings in the Field
These findings significantly impact both academic and practical domains:
- Performance Improvement: Enhanced algorithms lead to faster processing times, allowing for more complex problem-solving without compromising efficiency.
- Broader Applications: New algorithms facilitate the analysis of large datasets, important for fields like finance, healthcare, and e-commerce.
- Ethical Considerations: The analysis of algorithms raises questions about bias and fairness, especially in AI and machine learning contexts.
Algorithms must be designed responsibly to ensure ethical integrity in various applications.
"An algorithm is merely an efficient way to solve problems. Its significance lies in its application and impact."
Breakdown of Complex Concepts
Simplification of Advanced Theories
Understanding algorithms often requires breaking down complex principles. Fundamental concepts, such as Big O notation, allow for the analysis of algorithm efficiency. It describes how the runtime or space requirements grow concerning input size.
For example, an algorithm with a time complexity of O(n) implies that its time to execute grows linearly with the size of the input. Simplification of these terms can help researchers grasp their relevance in practical applications.
Visual Aids and Infographics
Visual representation can enhance the understanding of algorithms. Flowcharts, for instance, can diagram the steps of an algorithm, making it easier to follow the logic. Similarly, graphs can demonstrate comparative performance, highlighting the efficiency of different algorithms in a clear visual context.
Utilizing these tools can foster a better grasp of abstract concepts, making the study of algorithms more approachable.
Through this article, we will continue to explore various aspects of algorithms, ensuring a thorough comprehension of their fundamentals and real-world implications.
Prelims to Algorithms
Algorithms form the backbone of computer science, providing structured solutions to computational problems. Their significance cannot be overstated, as they dictate the efficiency and performance of software applications across various domains. By understanding algorithms, one gains insights into how data is processed, how tasks are automated, and ultimately how decisions are made in technology.
The study of algorithms extends beyond mere coding; it delves into the thought processes that underpin problem-solving in a systematic manner. When we dissect algorithms, we reveal methodologies that can be applied for achieving desired outcomes, optimizing resources, and eliminating redundancies.
In this section, we address the core concepts that are foundational to grasping algorithms, starting with a precise definition. Following that, we will explore the historical development of algorithms, acknowledging the key figures and milestones that shaped their evolution. This journey not only illuminates the technical aspects but also provides context to the innovative ideas that have driven advancements in computer science, ensuring that aspiring professionals appreciate the depth behind what might seem just code on a screen.
Definition of Algorithms
An algorithm can be defined as a set of well-defined instructions or rules designed to solve a specific problem or perform a task. These sequences of steps must be clear, unambiguous, and finite, concluding with a solution or output.
For example, consider the algorithm for sorting an array of numbers. It provides systematic steps to arrange the numbers in a specific order, enabling further analysis or operations to be conducted efficiently. To qualify as an algorithm, it should not only be functional but also optimal in terms of resource usage.
In essence, algorithms encapsulate a systematic approach to problem-solving seen in coding routines, mathematical computations, and artificial intelligence applications. They create a bridge between abstract concepts and practical implementation, showcasing their importance in computational logic.
History of Algorithms
The history of algorithms is interwoven with the development of mathematics and computer science, tracing back to ancient civilizations. The term 'algorithm' derives its roots from the name of the Persian mathematician and scholar Al-Khwarizmi, whose works in the 9th century laid the groundwork for algebra.
Historically, algorithms were often expressed in mathematical terms. Notable early examples include Euclid's algorithm for finding the greatest common divisor, which dates back to around 300 BC. As the field evolved, algorithms became crucial for computation, particularly with the advent of programmable machines in the 20th century.
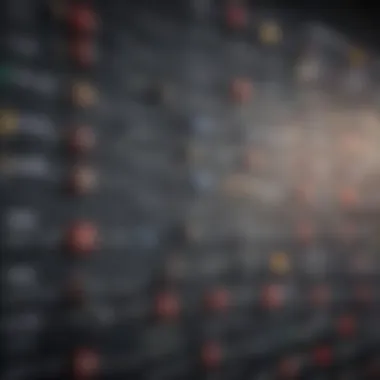
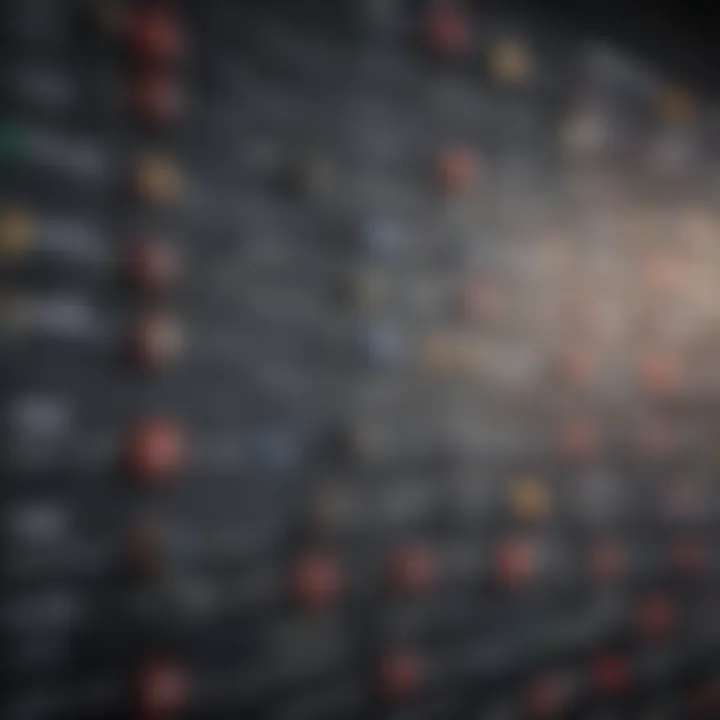
During the mid-1900s, prominent figures such as Alan Turing and John von Neumann made pivotal contributions. Turing introduced the concept of a theoretical machine, now known as the Turing Machine, which formalized the notion of computation. Von Neumannโs architecture set the stage for modern computer design, influencing how algorithms could be implemented in programming languages.
The rise of computer science in the latter half of the 20th century led to a surge in algorithm development, focusing on sorting, searching, and optimization problems, paving the way for a myriad of advanced techniques used today.
"Algorithms are the fuel that powers the software engines of our time, without which modern technology would not function."
Understanding the historical context aids in appreciating the role algorithms play in current technological advances. As we continue exploring their types, design, and applications, the thread uniting them is the ability to solve problems efficiently and reliably.
Types of Algorithms
Algorithms are the backbone of computer science, playing a vital role in not just theoretical study, but practical applications as well. Understanding the various types of algorithms is essential for anyone aiming for proficiency in programming or system design. Different types of algorithms serve unique purposes and come with their own sets of advantages and challenges. This section will explore the primary categories of algorithms, detailing their functions, significance, and potential pitfalls.
Sorting Algorithms
Sorting algorithms are designed to arrange data in a specific order, typically ascending or descending. Their efficiency is essential in various applications, from databases to user interface design. Notable sorting algorithms include Quick Sort, Merge Sort, and Bubble Sort. Each algorithm has a different mechanism and efficiency based on the nature of the data being processed.
- Quick Sort uses a divide-and-conquer approach, consistently outperforming others in average cases.
- Merge Sort is stable and works well with large datasets, but it consumes more space than other methods.
- Bubble Sort is simple but inefficient for large data sets, suitable primarily for educational purposes.
Sorting algorithms have significant applications in programming, particularly for optimizing search operations and managing datasets efficiently.
Search Algorithms
Search algorithms are crucial for locating specific data within a larger dataset. Performance varies widely depending on factors such as the data structure and the algorithm used. Popular search algorithms include Binary Search and Depth-First Search.
- Binary Search operates efficiently on sorted arrays, reducing the search space logarithmically.
- Depth-First Search is used in graph data structures and explores deeply, backtracking when necessary.
The importance of search algorithms lies in their fundamental role in applications like web search engines, database queries, and even artificial intelligence.
Graph Algorithms
Graph algorithms entail a set of procedures for processing graphs, which are structures that represent objects and their relations. Algorithms such as Dijkstra's Algorithm and Kruskal's Algorithm are instrumental in solving problems in networking, routing, and more.
"Graph algorithms are essential for understanding complex relationships in data."
- Dijkstra's Algorithm is used to find the shortest path between nodes in a weighted graph, often applied in mapping services.
- Kruskal's Algorithm helps in finding the minimum spanning tree for a graph, which has practical significance in network design.
Understanding graph algorithms is imperative in fields like social network analysis, logistical planning, and resource management.
Dynamic Programming Algorithms
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It relies on the principle of overlapping subproblems and optimal substructure. Algorithms like Fibonacci Sequence Calculation and Knapsack Problem are classic examples of dynamic programming.
Dynamic programming optimizes performance, significantly reducing time complexity in scenarios that would otherwise require extensive computation. It is a valuable tool for programmers in solving problems that are recursive by nature.
Randomized Algorithms
Randomized algorithms leverage random numbers to influence their execution path. While often used to simplify the process and improve efficiency, their unpredictable nature comes with trade-offs. Examples include Randomized Quick Sort and Monte Carlo Methods.
- Randomized Quick Sort uses random pivot selection to potentially improve average-case speed.
- Monte Carlo Methods apply randomness to understand complex systems and processes, widely used in statistical sampling and numeric computation.
Understanding randomized algorithms is crucial for tackling challenges in areas such as complex simulations and optimization problems.
In summary, each type of algorithm serves unique functions that can enhance computational efficiency under various circumstances. Their significance extends across different fields, making it essential for computer science practitioners to comprehend their applications and implications.
Algorithm Design and Analysis
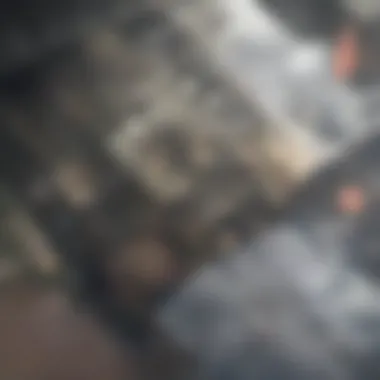
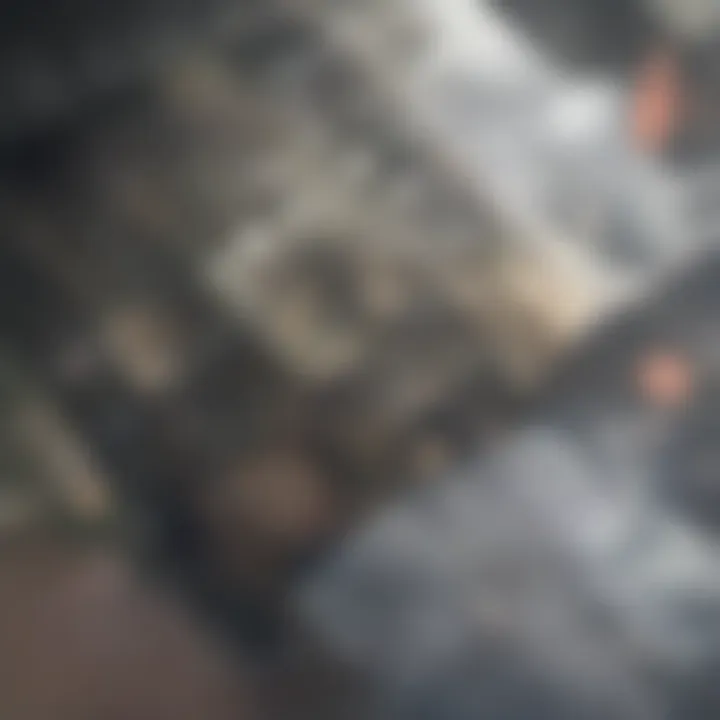
Algorithm design and analysis is a crucial aspect of computer science. This component focuses on developing efficient algorithms while evaluating their performance both in time and space. The relevance of this topic lies in its direct impact on computational efficiency, ultimately affecting how software operates in real-world applications. Good algorithm design leads to robust applications, while poor design can lead to inefficiencies.
Design Techniques
Design techniques provide various strategies to develop algorithms, each with unique characteristics.
Divide and Conquer
Divide and conquer is a prominent technique that focuses on breaking a problem down into smaller, manageable subproblems. Each of these subproblems is solved independently, and their solutions are combined to form the final solution. The key characteristic of this approach is its recursive nature, which allows for efficient resolution of large problems.
The beneficial aspect of this technique is its complexity reduction. By tackled smaller parts of a problem rather than the entire issue at once, it often yields more efficient algorithms. Common examples include quicksort and mergesort.
However, one disadvantage can be the overhead of recursive calls, which may lead to high memory consumption in some cases. Despite this, divide and conquer remains a popular choice due to its effectiveness in optimizing algorithm performance.
Greedy Algorithms
Greedy algorithms are another important design choice that aims to make the most immediate optimal choice at each step. This approach often leads to a solution for problems in a quicker manner than other techniques. The primary characteristic of greedy algorithms is their focus on local optimums in the hope that they lead to a global optimum.
Its popularity comes from the simplicity in implementation and often improved performance. Classic examples include Prim's and Kruskal's algorithms for finding minimum spanning trees.
However, greedy algorithms do not always produce the best overall solution. A notable disadvantage is that they might miss out on better solutions that require more extended consideration. Thus, understanding when to apply this technique is important for an effective algorithm design.
Backtracking
Backtracking is a design method that explores all possible solutions to a problem and evaluates them to find the most optimal one. This technique is based on the idea of incremental building of candidates, and abandoning those that fail to fulfill the criteria of the problem. The key characteristic of backtracking is its systematic exploration of the solution space.
This technique is beneficial when problems require exhaustive searching, such as constraint satisfaction problems like Sudoku. However, backtracking can be inefficient in cases with large solution spaces, as it might navigate through numerous possibilities without yielding results quickly. Its strength lies in its comprehensiveness, which makes it valuable for certain problem types.
Complexity Analysis
Complexity analysis is concerned with evaluating the performance of algorithms in terms of their required time and space. This analysis becomes pivotal when comparing the efficiency of different algorithms addressing the same problem.
Time Complexity
Time complexity refers to the computational time an algorithm takes as a function of the input size. It helps understand the scalability of algorithms, which is essential for assessing how they will perform under increasing data loads. The key characteristic here is the growth rate of an algorithm's run time relative to input size, providing insight into efficiency.
Time complexity is a beneficial measure as it allows for comparisons between different algorithms. Common notations used in time complexity include Big O notation, which describes the upper limit of time requirements. However, the main limitation is that it doesn't account for constant factors that can affect real execution time.
Space Complexity
Space complexity measures the total space required by an algorithm as a function of the input size. This includes the space for inputs, outputs, and auxiliary storage. Its primary characteristic is the memory usage, crucial for environments with limited resources.
Understanding space complexity is beneficial for designing algorithms that will run on devices with constrained memory. Similar to time complexity, space complexity is often expressed in Big O notation. Yet, it can sometimes overlook practical constraints like cache size or the differences in the physical address space, making evaluations less straightforward.
"Both time and space complexity analyses are vital for evaluating the efficiency and scalability of algorithms, guiding the selection of the most appropriate algorithm for specific tasks."
Practical Applications of Algorithms
Algorithms play a vital role in various domains of modern computing. Their practical applications help streamline processes, enhance decision-making, and solve complex problems efficiently. By understanding the different areas where algorithms are employed, one can appreciate their fundamental importance in driving technological progress. Key aspects include efficiency, accuracy, and scalability. These characteristics enable algorithms to operate within various parameters, making them applicable to real-world scenarios.
A significant advantage of utilizing algorithms lies in their ability to analyze data faster than traditional methods. This speed is crucial in fields where data processing is enormous, such as in artificial intelligence, data science, and software development. Therefore, recognizing how algorithms function within these sectors not only showcases their versatility but also highlights the potential impacts they can have on society at large.
"Algorithms are the backbone of technological advancements. They shape how we interact with machines and process information effectively."
Algorithms in Artificial Intelligence
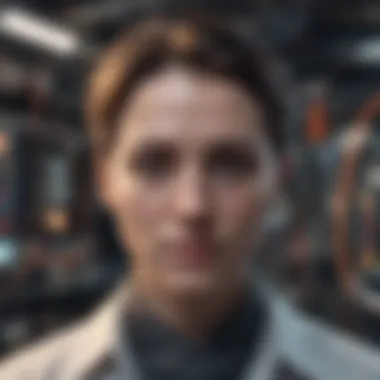
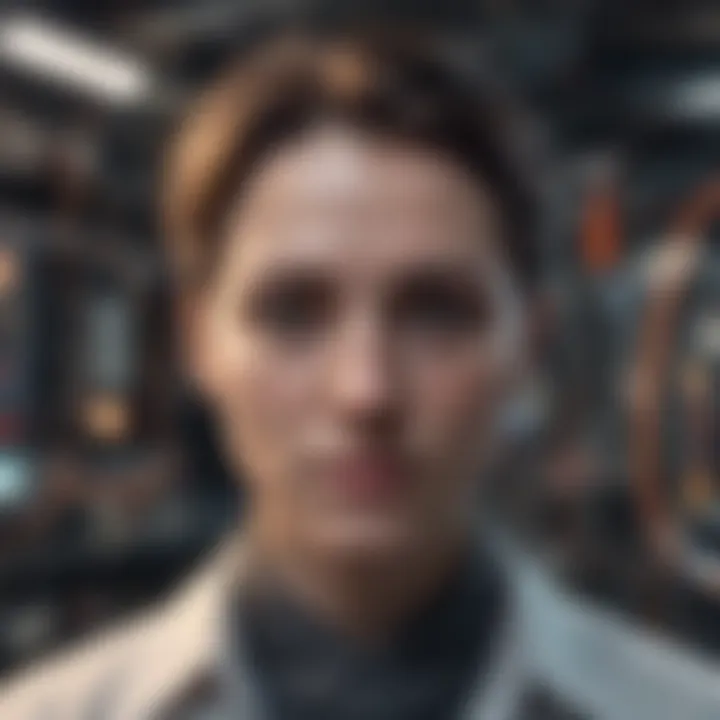
Artificial intelligence relies heavily on algorithms to perform tasks typically associated with human intelligence. The implementation of algorithms in AI has transformed industries. From natural language processing, which enables machines to understand human language, to computer vision, which allows machines to interpret visual data, algorithms enhance performance and enable innovation.
Moreover, machine learning, a subfield of AI, utilizes algorithms to learn from and make predictions based on past data. By analyzing patterns, these algorithms enable systems to improve over time, yielding more accurate results. For instance, the neural networks powering image recognition or recommendation systems are algorithm-driven. The depth of algorithms in AI underscores their significance in developing smarter technologies.
Algorithms in Data Science
Data science leverages algorithms to extract meaningful insights from raw data. By implementing statistical and computational techniques, data scientists can uncover patterns and inform decision-making processes. Common algorithms in data science include regression for predicting outcomes, clustering for grouping similar data points, and classification for identifying data categories.
The significance of these algorithms lies in their ability to handle vast datasets, revealing trends that might not be visible otherwise. For example, using data mining algorithms, businesses can analyze consumer behavior, leading to improved marketing strategies. Thus, algorithms serve as indispensable tools for data analysts aiming to translate complex information into actionable insights.
Algorithms in Software Development
In software development, algorithms are crucial for creating efficient and functional applications. Developers implement algorithms to solve problems ranging from sorting data to optimizing user experiences. For example, algorithms determine the best routes in navigation applications like Google Maps, managing to provide users with quick and accurate directions.
Additionally, coding practices often include algorithmic thinking. This approach enhances code effectiveness and maintainability. Common algorithmic concepts such as recursion, dynamic programming, and searching mechanisms are fundamental to building efficient software. Knowledge of algorithms empowers developers to design better solutions, significantly impacting the overall software quality.
Ethical Considerations of Algorithms
The role of algorithms in modern society extends far beyond mere computational functions. Algorithms not only enhance productivity but also shape decision-making processes across various sectors. This section will explore the ethical considerations associated with algorithm implementation and development. Understanding these issues is critical to fostering responsible innovation that acknowledges the potential repercussions on individuals and communities.
One core element of ethical considerations is the need to address bias in algorithms. Algorithms are designed based on data sets, and if these data sets reflect historical prejudices or societal inequalities, the resulting algorithms may perpetuate those biases. Bias can manifest in various ways, such as algorithmic discrimination against certain demographics in hiring practices or unfair lending decisions in finance. Tackling this challenge involves rigorous scrutiny of data sources, promoting diversity in the development teams, and ensuring the algorithms are tested on a broad range of scenarios to prevent biased outcomes.
Moreover, there should be a proactive effort to educate developers on the ethical implications of their creations. This education might include principles of fairness, accountability, and the social impact of their algorithms. Bias in algorithms is not an isolated issue; it has real-world consequences. As algorithms make more decisions that influence daily life, addressing bias is essential in creating more equitable systems.
Bias in Algorithms
Bias in algorithms is a significant concern as it can lead to systematic disadvantages for certain groups. There are three primary sources of bias:
- Data Bias: If training data contains inherent biases, these biases are likely to be reflected in the algorithm's decisions. For example, an algorithm trained on primarily male user data may struggle to accurately predict female behavior.
- Algorithm Design Bias: Developers' choices in choosing the model or deciding on the parameters can introduce biases. The lack of diverse perspectives in the design teams can further exacerbate these issues.
- Feedback Loop Bias: When algorithms continuously learn from biased outcomes, they reinforce pre-existing biases. For instance, if a hiring algorithm favors certain skill sets, the job candidates it recommends will only match those criteria, perpetuating a cycle of favoritism.
Addressing these biases requires a comprehensive approach. Institutions should foster interdisciplinary collaborations that include ethicists, sociologists, and technical experts to create holistic solutions that consider social implications. Algorithmic audits and scrutinizing algorithms throughout their lifecycle are also paramount to ensure fairness and inclusivity.
Transparency and Accountability
In conjunction with bias, transparency and accountability play a vital role in the ethical design and implementation of algorithms. Stakeholders must understand how algorithms make decisions and hold creators accountable for their results.
Transparency involves revealing to users how algorithms operate, their underlying assumptions, and the data driving them. Increased transparency helps users comprehend potential biases and refine their expectations. In many cases, understanding the algorithm can empower users to appeal decisions or question outcomes perceived as unjust. This awareness will also instigate dialogue around best practices and standards in algorithm development.
Accountability, on the other hand, requires that developers and organizations take responsibility for the impact their algorithms have on society. By establishing clear guidelines and regulations on algorithmic ethics and requiring regular audits, organizations can promote ethical practices. Moreover, frameworks like the EU's General Data Protection Regulation (GDPR) set precedents that guide companies toward responsible algorithm usage.
The Future of Algorithms
The future of algorithms holds significant importance in the field of computer science. As technology continues to evolve rapidly, the design and utilization of algorithms will adapt to meet new challenges and opportunities. Algorithms will become increasingly crucial in processing vast amounts of data, optimizing processes, and enhancing decision-making capabilities. Understanding their evolution helps anticipate changes in various fields such as artificial intelligence, data science, and beyond.
Trends in Algorithm Development
Several notable trends are emerging in algorithm development. These trends reflect the current needs in technology and predict future directions. A few key areas include:
- Machine Learning Algorithms: The rise of machine learning continues to shape algorithm design. Algorithms that can learn from data improve their accuracy over time and provide better predictions or classifications.
- Explainable AI: As artificial intelligence becomes more pervasive, the demand for transparency increases. Developers are focusing on algorithms that not only deliver results but also explain their decision-making processes. This will help in establishing trust in AI systems.
- Optimization Algorithms: Efficiency is vital. Optimization algorithms that focus on resource management are critical as industries aim to reduce waste and improve performance. Examples include algorithms used in logistics and supply chain management.
These trends indicate a shift towards algorithms that are adaptive, transparent, and efficient, addressing both computational needs and ethical considerations.
Impact of Quantum Computing
Quantum computing is poised to redefine algorithm efficiency. Traditional algorithms may struggle to keep pace with the processing power of quantum machines. The potential benefits include:
- Speed: Quantum algorithms, underpinned by the principles of quantum mechanics, have the capability to solve problems significantly faster than classical algorithms.
- Complex Problem Solving: Quantum computing excels at solving problems that involve complex data structures and massive datasets, such as those found in cryptography and materials science.
- New Algorithm Paradigms: Quantum computing will prompt the creation of new algorithms specifically designed to exploit quantum properties, leading to advancements in various fields.
"As we look towards a future that includes quantum computing, it's essential to reconsider existing algorithms and how they might evolve to leverage this emerging technology."
The impact of quantum computing on algorithms heralds an era of new possibilities. It presents a pivotal opportunity to enhance computational capability far beyond the limitations of classical computing.