Mathematical Foundations Essential for Programmers
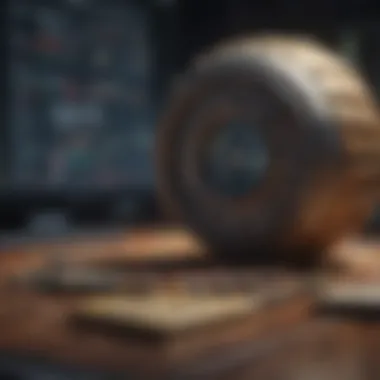
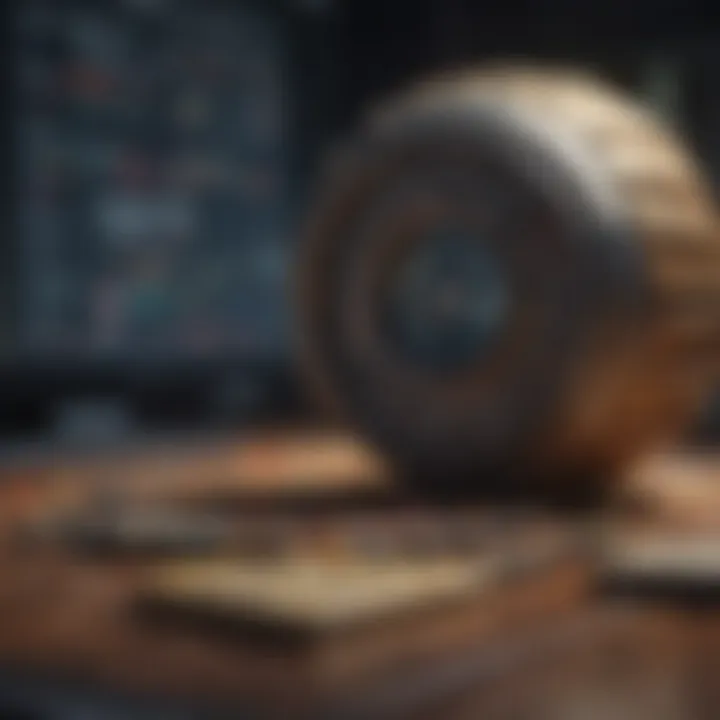
Intro
In the world of programming, mathematical foundations play a pivotal role. Understanding the essential concepts of mathematics allows programmers to design efficient algorithms, structure code effectively, and solve complex problems. Without a solid grasp of mathematics, a programmer may struggle to understand how algorithms work or how to optimize their code effectively.
This article delves into the necessary mathematical principles that programmers ought to be familiar with, starting from basic arithmetic and extending into advanced areas like algebra, calculus, and discrete mathematics. Each section will discuss how these principles are applicable in programming practices, making a clear connection between math and coding.
Key Research Findings
Overview of Recent Discoveries
Mathematics has seen numerous developments that directly impact programming. For example, research in algorithm complexity reveals insights into how certain mathematical techniques can dramatically enhance the efficiency of data handling. In addition, advancements in numerical analysis improve the precision of computations, which is critical for applications in scientific programming and simulations.
Significance of Findings in the Field
Understanding these findings is crucial for programmers who aim to create optimized software. The importance of diving into core mathematical principles cannot be understated. Knowledge of statistics provides insight into data interpretation, while linear algebra is essential for graphics programming and machine learning algorithms. These foundations shape not only the efficiency and effectiveness of programs but also the programmer's ability to innovate.
"Mathematics is the language with which God has written the universe." β Galileo Galilei
Breakdown of Complex Concepts
Simplification of Advanced Theories
To make complex mathematical theories more accessible, it is beneficial to break them down into simpler components. For instance, calculus can be intimidating at first glance, but it fundamentally relies on concepts of limits and rates of change, which are intuitively comprehensible. By thoroughly understanding these basic ideas, programmers can more readily apply calculus concepts in their work, especially in fields like optimization and predictive modeling.
Visual Aids and Infographics
Using visual aids can significantly enhance the understanding of mathematical concepts in programming. Graphs and charts can depict relationships, show data distribution, and simplify the comprehension of algebraic equations. Infographics summarizing complex theories or algorithms can provide a quick reference for busy programmers, aiding in retention and application.
Incorporating both visual elements and simplified explanations can bridge the gap between theory and practice, empowering programmers to leverage mathematics effectively.
As we progress through the following sections, the intention is to build upon this foundation, leading to a comprehensive understanding of how mathematics interacts with programming at every stage.
Preface to Mathematics in Programming
Mathematics serves as the backbone of programming and computer science. An understanding of mathematical principles is essential for effective programming. This introduction highlights key benefits and unique considerations.
When programmers grasp foundational mathematics, they enhance their problem-solving skills. Mathematical concepts allow for clearer thinking and better analytical abilities. For instance, knowledge of algorithms often relies on understanding mathematical logic and structures. By applying mathematical notions, programmers can optimize code and improve system efficiency.
The Role of Mathematics in Computer Science
Mathematics plays a pivotal role in computer science. Algorithms are built using mathematical formulas and proofs. These algorithms can solve complex problems or analyze large datasets. In programming, understanding complexity theory helps optimize solutions. It teaches how to manage resources efficiently, impacting overall system performance.
Furthermore, mathematics is crucial in creating software models. Developers use statistics and probability to predict behavior patterns. This is particularly true in fields like machine learning. A solid grasp of math allows developers to write effective models and interpret data correctly.
"Mathematics is not just a tool for computation; it is the framework for understanding complex systems."
Mathematics: Not Just for Mathematicians
Programming is often viewed as a practical field, yet it draws heavily from mathematics. Many programmers may feel hesitant about their mathematical skills. However, the reality is that math is integral to various branches of programming. Areas like data science, artificial intelligence, and game development rely significantly on mathematical concepts.
Understanding basic concepts of algebra can simplify programming logic. For example, variables in programming correspond directly to algebraic variables. Comprehending functions in math parallels understanding programming functions. This alignment makes learning more intuitive.
In summary, mathematics is a universal language that enhances programmers' understanding. It empowers them to tackle challenges head-on. By shifting the perception that math is only for mathematicians, programmers can embrace its relevance and utility in their craft.
Basic Arithmetic Operations
Basic arithmetic operations form the foundation of most mathematical and programming concepts. These operations are not only fundamental to mathematics but also essential in programming, where calculations and logical reasoning are prevalent. Understanding how to efficiently perform arithmetic tasks is crucial since they are often the first step in more complex problem-solving scenarios. All computer programs rely on these operations to manipulate data, and thus, programmers must be familiar with them to write effective code.
The core operations include addition, subtraction, multiplication, and division. These operations serve as building blocks that enable programmers to address a variety of tasks, from simple calculations to complex algorithms.
Addition and Subtraction
Addition and subtraction are the primary arithmetic operations that form the basis of mathematical logic. Addition combines values to yield a sum. For instance, if a program tracks user scores in a game, it may use addition to update the scores whenever a player achieves a milestone.
On the other hand, subtraction calculates the difference between two values. In programming, it can be used to manage inventories, calculate changes in state, or track the remaining resources. For example, if a user purchases an item, subtraction reduces the available stock in the system.
From a programming perspective, these operations can be implemented in various programming languages. For example, in Python:
In this snippet, is calculated by adding and . Meanwhile, is updated by subtracting the sold quantity from available stock. Clearly, understanding addition and subtraction is invaluable for anyone developing software that relies on numerical manipulation.
Multiplication and Division
Multiplication extends the concept of addition by adding a number to itself a specified number of times. This operation is particularly useful in programming when scaling values. For example, when calculating the total price of an item multiplied by its quantity, multiplication efficiently computes this in a fraction of the time it would take using repeated addition.
Similarly, division breaks a value into equal parts. It is essential for scenarios where proportionality is key. For instance, in data analysis, finding the average requires summing a set of numbers and then dividing by the count of those numbers. This process can easily be implemented in code.
In programming languages like Java:
Here, represents the cost of multiple items, while calculates an average value by dividing by . Overall, these operations are critical not only for their mathematical beauty but also for their practical applications in everyday coding tasks.
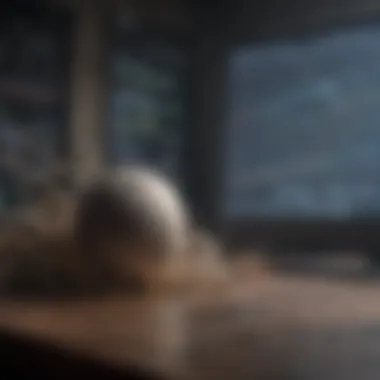
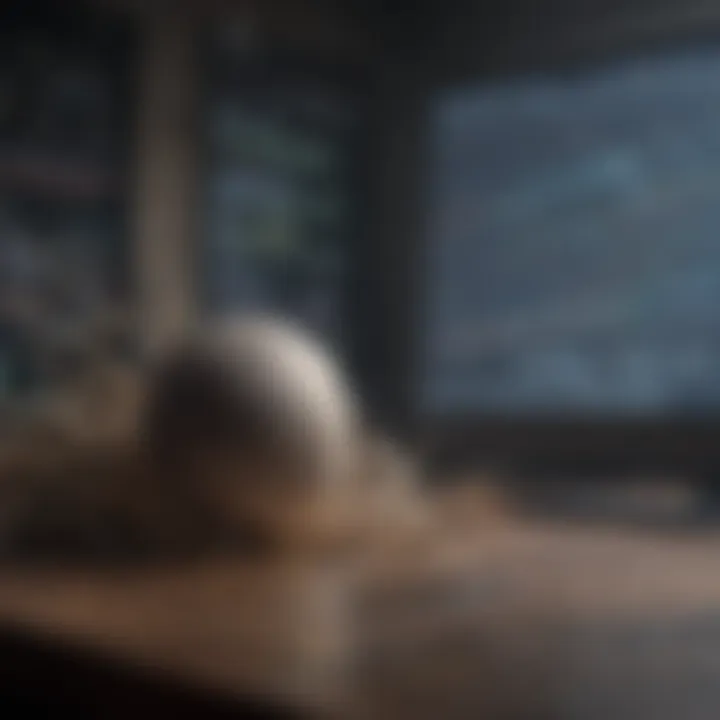
Algebraic Concepts in Programming
Algebra forms the backbone of many programming techniques. Understanding algebraic concepts is critical for programmers to effectively manipulate data and formulate solutions. This section will explore the components that underlie programming logic. We will discuss the significance of variables, constants, equations, and inequalities. Each aspect is fundamental to creating effective algorithms and understanding how to handle different data types.
Variables and Constants Explained
Variables and constants are essential in programming, serving distinct but interconnected roles. A variable is a symbolic name associated with a value that can change during program execution. For instance, in a simple code snippet:
Here, is a variable that contains the value . It can change if we later assign it a different value.
In contrast, a constant is a fixed value that does not change. Using constants in a program can enhance clarity and reduce errors. For example:
In this example, is a constant representing a fixed value. It is important for readability and maintaining a codebase.
The following points illustrate the benefits of using variables and constants:
- Variables enable dynamic programming: This allows the program to adapt to user inputs and other changing conditions, which is crucial in many applications.
- Constants enhance code reliability: By using constants, programmers can avoid unnecessary changes in the code, which helps prevent bugs.
- Readability: Using descriptive variable and constant names makes the code easier to understand, which is essential for both the original programmer and others who may work on the code later.
Understanding how to effectively use variables and constants will lead programmers to construct more efficient and robust programs.
Equations and Inequalities
Equations and inequalities are also crucial in programming, allowing for complex decision-making processes. An equation asserts that two expressions are equal. In contrast, an inequality expresses a relationship where one expression is greater than or less than another.
An example of an equation might be:
Here, is checked for equality with . If this condition holds true, a specific code block will execute, demonstrating conditional logic based on equations.
Inequalities, on the other hand, often govern control structures:
In this example, if is greater than , the program will respond accordingly. This highlights how inequalities enable programmers to manage various scenarios within their applications.
Understanding equations and inequalities allows programmers to:
- Model complex systems: By applying mathematical principles, programmers can represent real-life scenarios realistically within their code.
- Implement logic in decision-making: Control flow in programming relies heavily on the ability to compare variables and make choices based on their relationships.
- Solve problems effectively: Many algorithms require solving equations or evaluating inequalities to achieve their goals. Thus, a solid understanding of these concepts is indispensable.
In programming, the application of algebraic concepts like variables, constants, equations, and inequalities transcends mere computation. They provide the structure needed to build efficient solutions and navigate complex problem constraints effectively.
Functions and Their Applications
Functions play a vital role in both mathematics and programming. They serve as the building blocks for creating complex systems. Understanding functions equips programmers with a framework for solving problems efficiently. Functions allow the encapsulation of code into reusable components. This aids in simplifying the complexity of software development. Their applications range from basic calculations to complex algorithms.
Understanding Functions in Mathematics
In mathematics, a function is a relationship between a set of inputs and a set of outputs. The input is often referred to as the independent variable, while the output is the dependent variable. For example, in the function ( f(x) = x^2 ), the value of ( f ) depends on ( x ). Functions are essential in describing mathematical relationships.
Functions have several important properties:
- Domain: The set of all possible input values.
- Range: The set of all possible output values.
- Operations: Functions can be combined through operations such as addition, subtraction, multiplication, and division.
Understanding these aspects enables programmers to use functions effectively in code. The principles of functions allow developers to predict behavior and manipulate data systematically.
Coding Functions in Programming Languages
Translating mathematical functions into programming languages is crucial for practical applications. Each programming language handles functions uniquely, but the core principles remain the same. A function in code typically includes a name, parameters, and a body that defines what the function does.
For example, here is a simple function written in Python:
In this piece of code:
- The function is named .
- It takes one parameter, .
- The function returns the square of .
Using functions like this promotes code reuse and clarity. They help in organizing code logically and make debugging easier. Functions can also be made more complex by including multiple parameters, return types, and exception handling.
The integration of functions enhances maintainability and scalability in projects. As projects grow, having defined functions allows teams to work on different components without interference. The knowledge of functions, thus, not only applies in theoretical mathematics but serves practical purposes in software development.
"Functions are essential for effective programming; they simplify complexity and enhance code reusability."
In summary, a deep understanding of functions equips programmers with the tools needed for efficient problem-solving and effective code management.
Discrete Mathematics for Computer Science
In the realm of programming, the understanding of discrete mathematics plays a vital role. It serves as a foundation for various computer science fields, including algorithms, data structures, and optimization. A programmer's ability to think abstractly and solve problems systematically is highly influenced by concepts drawn from discrete mathematics.
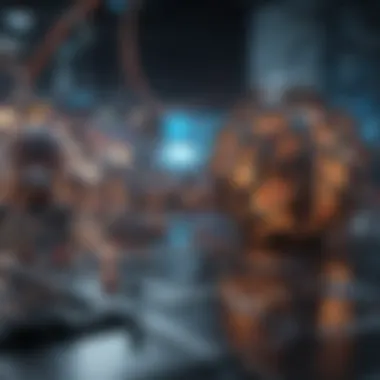
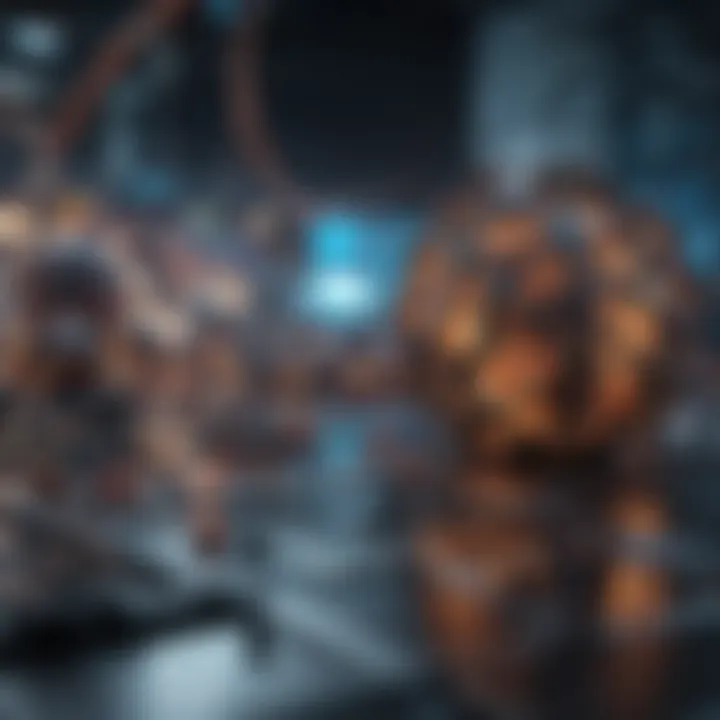
Discrete mathematics focuses on structures that are fundamentally discrete rather than continuous. Examples include integers, graphs, and logical statements. This area of study lays the groundwork for essential programming techniques, such as recursion and state machines. Consequently, mastering discrete mathematics not only enhances problem-solving skills but also provides tools vital for efficient programming.
The main benefits of studying discrete mathematics include its applications in data organization, algorithm efficiency, and logic formation. As programmers tackle complex problems, the principles of discrete mathematics guide them in developing structured and efficient solutions.
Furthermore, understanding how to apply these concepts can vastly improve a programmer's knowledge in areas such as database management and cryptography.
Set Theory and Its Relevance
Set theory provides the basic language of mathematics through the study of sets, collections of objects that can be treated as a single entity. In programming, sets enable better data organization and manipulation. For example, operations on sets include union, intersection, and difference. These operations directly relate to database queries or data analytics tasks.
Programmers utilize set theory not only to process data effectively, but also to optimize data storage. A clear understanding of how to group, filter, and manage sets helps in designing efficient algorithms. Importantly, the principles of set theory help with error detection and data verification, which are crucial in ensuring integrity in systems.
Logic and Boolean Algebra
Logic is the foundation of programming languages. Boolean algebra introduces the concepts of true and false, enabling decision-making processes that are at the heart of programming. In any program, conditional statements rely heavily on logical expressions that result in Boolean values.
Being proficient in logical reasoning allows programmers to create robust algorithms. Frequently, the efficiency of a program is dictated by the logic structures employed. For instance, understanding how different logical operators workβsuch as AND, OR, and NOTβcan significantly advance a programmer's capability to design sophisticated control flows.
Moreover, logical reasoning maneuvers can aid in debugging, as they establish guidelines for verifying conditions and outcomes. By applying Boolean algebra, programmers can manipulate and derive conditions that drive program execution, ensuring that every possible scenario is addressed.
"Discrete mathematics empowers programmers to systematically encode complex logic into functional code."
Finale
In summary, discrete mathematics is fundamental to computer science, shaping the way programmers approach problem-solving. By grasping the concepts within set theory, logic, and Boolean algebra, programmers create efficient, effective, and reliable software. In a world increasingly driven by data, the utilization of discrete mathematics will only grow in importance, guiding the future of programming and technology.
Combinatorics and Graph Theory
Combinatorics and graph theory are essential disciplines within the field of mathematics, particularly relevant to programming. Both areas provide tools and concepts that foster a better understanding of structure, organization, and complexity in programming tasks.
The applications range from optimizing algorithms to analyzing data structures. This section will discuss the fundamental aspects of combinatorics and graph theory, their intersections, and their practical implications for developers and researchers in computer science.
Fundamentals of Combinatorics
Combinatorics is the study of counting, arrangement, and combination of objects. This field becomes crucial when dealing with algorithms that require repetitive counting or selection processes. Combinatorial thinking allows programmers to evaluate possible configurations of data and develop efficient solutions to complex problems.
Key points in combinatorics include:
- Permutations: The arrangement of objects in a specific order. For example, when developing algorithms such as sorting, understanding permutations helps in predicting outcomes based on input variations.
- Combinations: Selections of objects without regard to order. This is essential in scenarios like selecting items for calculations where the order does not affect the result.
- Binomial Coefficients: They play a vital role in calculating combinations. The understanding of binomial coefficients can be important in optimization problems.
Grasping these concepts enhances the programmer's ability to devise algorithms that are not only functional but also efficient, reducing unnecessary computations in tasks.
Understanding Graphs and Algorithms
Graph theory focuses on studying graphs, which are mathematical structures made of vertices and edges. In programming, graphs represent relationships and structures that can model numerous real-world problems.
Some applications of graph theory in programming include:
- Social Networks: Represent the connections between individuals as graphs. Understanding these structures enables the development of algorithms that analyze relationships, trends, and user interactions.
- Pathfinding Algorithms: Used in navigation and routing applications, such as Google Maps. These algorithms rely on graph structures to determine the best route from point A to point B.
- Network Analysis: Analyzing flows in networks can help improve the performance of various systems, including telecommunications or transport networks.
Furthermore, algorithms associated with graphs, such as Dijkstra's and A* algorithms, draw on combinatorial principles to establish connections and minimize distances effectively.
In summary, a solid grasp of combinatorics and graph theory equips programmers with the necessary tools to analyze complex problems and develop solutions that are both innovative and efficient. Understanding these mathematical foundations enables better algorithm design and optimizes overall program performance.
Calculus and Its Programming Implications
Calculus plays a significant role in programming, especially in fields that involve continuous change, such as computer graphics, game development, and machine learning. Its principles enable programmers to model real-world phenomena and optimize processes in their code. Understanding calculus helps in developing algorithms that require precision in calculations. The implications of calculus in programming can lead to more efficient code and better problem-solving strategies, particularly when dealing with complex systems.
Basics of Differential Calculus
Differential calculus focuses on the concept of a derivative, which represents the rate of change of a function. This is especially useful in programming when analyzing how changes in input affect output. For programmers, derivatives can inform decisions about optimizations. For example, if a programmer knows how the performance of an algorithm behaves as the input size increases, they can adjust the algorithm for optimal performance. In practice, areas such as real-time systems and physics simulations rely heavily on the principles of differential calculus.
- Rate of Change: Understanding how variables change helps in controlling animations and movements in programs.
- Tangent Lines: Tangents are commonly used to approximate functions in various algorithms, such as finding limits.
- Optimization: Calculating local maxima and minima enables programmers to find the most efficient solutions to problems.
Integral Calculus in Data Analysis
Integral calculus is concerned with the accumulation of quantities, such as areas under curves. This concept is crucial when programmers analyze data sets, especially in statistics and data mining. Integrals allow for the determination of probabilities, averages, and total quantities, which are essential in making informed decisions based on data.
- Area Calculation: Integral calculus can be used for computing the area under a curve, which can represent various data metrics.
- Accumulation Functions: Programmers can model the cumulative distribution functions of datasets using integrals.
- Statistical Applications: Integrals are used to solve problems related to continuous probability distributions.
In summary, calculus is an indispensable tool for programmers who seek to deepen their analytical capabilities and enhance the performance of their applications. Understanding both differential and integral calculus opens doors to advanced mathematical modeling and data management techniques that can dramatically improve the quality of code and overall software engineering practices.
Probability and Statistics for Programmers
Probability and statistics form a critical segment of the mathematical foundations necessary for effective programming. The ability to understand and manipulate data is paramount in software development, particularly in fields such as machine learning, data science, and artificial intelligence. This chapter delves into the significance of probability theory, which helps to evaluate the likelihood of certain events and outcomes, and statistical analysis, which offers tools for interpreting and drawing conclusions from data.
Understanding these concepts not only enhances a programmerβs ability to create robust algorithms but also positions them to tackle complex problems more effectively. A solid grounding in probability and statistics allows developers to make informed decisions based on data rather than mere guesswork, fostering a more accurate representation of the tasks at hand.
Understanding Probability Theory
Probability theory is the branch of mathematics that deals with uncertainty and the analysis of random events. In programming, it is vital for several reasons:
- Risk Assessment: Programmers often need to assess risks. For example, when developing financial applications, understanding the chance of default on loans becomes essential.
- Decision Making: Algorithms that require a decision-making process need to leverage probability to choose the best course of action. This is evident in recommendation systems used by platforms like Netflix or Spotify.
- Predictive Analytics: Many programming projects, especially in data science, involve making predictions based on historical data. Probability theory helps quantify these predictions, allowing programmers to build models that forecast future trends.
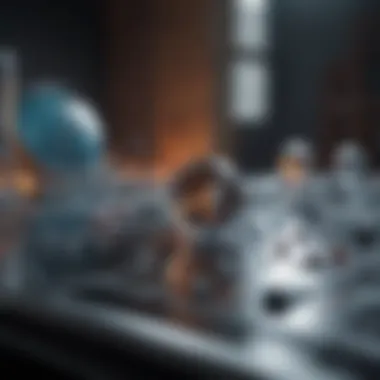
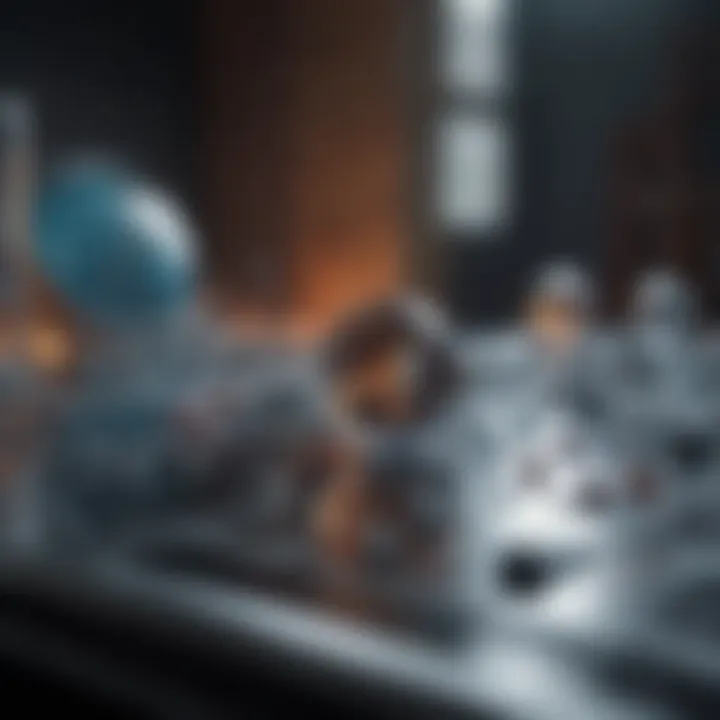
A fundamental concept in probability theory is the idea of random variables, which are variable quantities whose values are determined by inherent randomness. Another important aspect is probability distributions, such as the normal distribution, which describes how the values of a variable are distributed.
When implementing these concepts in programming, one might examine libraries such as NumPy in Python, which provides functions for generating random numbers and performing statistical operations.
"Understanding the basics of probability is more than just academicβit's key for effective software development across multiple domains."
Statistical Analysis in Programming
Statistical analysis equips programmers with techniques to summarize, visualize, and draw conclusions from datasets. Here are essential elements involved in this aspect of programming:
- Descriptive Statistics: This includes measures like mean, median, and standard deviation. These statistics provide a snapshot of the data, allowing programmers to glean insights quickly.
- Inferential Statistics: This involves making generalizations from a sample to a population. Techniques include hypothesis testing and confidence intervals, which enable programmers to draw conclusions about large datasets from smaller samples.
- Data Visualization: Understanding statistics can also enhance how programmers visualize data. Libraries like Matplotlib in Python allow developers to create informative graphs and charts to present data findings effectively.
Applications of Mathematics in Programming
Mathematics serves as a cornerstone in programming, guiding the development of algorithms and structures that form the foundation of software solutions. The application of mathematical concepts plays a critical role in making programming more efficient and effective. In this section, we delve into how mathematics informs various aspects of programming, particularly in algorithm development and data structures. Understanding these principles is fundamental for students, researchers, educators, and professionals alike. By leveraging mathematical foundations, programmers can enhance their problem-solving capabilities and create robust systems.
Algorithm Development
Algorithms form the crux of programming. They are finite sequences of instructions that, when executed, perform a specific task or solve a problem. Mathematics is integral in forming these algorithms.
Mathematical concepts such as logic, probability, and statistics help in designing algorithms that are not only correct but also efficient. For instance, understanding Big O notation allows programmers to evaluate the performance and efficiency of algorithms.
Several key factors come into play when considering algorithms:
- Complexity: Analyzing time and space complexity assures that the algorithm performs optimally as the size of input grows.
- Correctness: Mathematical proofs ensure that an algorithm will function as intended under specified conditions.
- Optimization: Mathematics aids in refining algorithms to use fewer resources and execute faster. Techniques such as dynamic programming utilize mathematical strategies to reduce the redundancy in calculations.
The intersection of mathematics and programming enhances not only the functionality of algorithms but also their reliability. Understanding these elements empowers programmers to tackle larger problems efficiently.
Data Structures and Their Mathematical Basis
Data structures organize and store data for efficient access and modification. The choice of data structure profoundly affects the performance of algorithms and overall program execution. Mathematics, particularly discrete mathematics, provides the framework for understanding various data structures.
For instance, concepts drawn from set theory, combinatorics, and graph theory are essential in analyzing and implementing data structures such as:
- Arrays: Essential for storing elements in contiguous memory locations, benefiting from the mathematical foundation of sequences.
- Trees and Graphs: Structured representations depicting relationships. Mathematics aids in understanding traversal, searching, and optimization techniques in such structures.
- Hash Tables: Using hash functions formulates a method for data retrieval through mathematical transformations, optimizing search time.
- Linked Lists: These structures rely on mathematical representation of links to efficiently organize memory.
Understanding the mathematical basis for these structures enhances a programmer's ability to choose the right data storage method, improving software performance.
Mathematics in Artificial Intelligence
Mathematics plays a crucial role in the domain of Artificial Intelligence. It provides the formal framework and tools that underpin machine learning algorithms, statistical models, and computer vision tasks among others. Understanding the mathematical foundations enables better insights into how various AI systems function. Moreover, it equips programmers to innovate and troubleshoot effectively. In this section, we will delve into essential aspects of mathematics in AI, focusing on machine learning essentials and optimization techniques.
Machine Learning Essentials
Machine learning involves a myriad of mathematical concepts. Statistics is at its core, guiding the understanding of data distributions, hypothesis testing, and model validation. The importance of linear algebra cannot be understated as well, with concepts like vectors and matrices being fundamental in representing data and transformations within algorithms.
- Key topics in machine learning include:
- Data Representation: How data is structured and processed.
- Algorithms: Various types, including supervised and unsupervised learning.
- Evaluation Metrics: Understanding performance through metrics such as accuracy, precision, and recall.
Many algorithms, such as neural networks, rely on calculus for optimization. The process of backpropagation used in training these models involves taking derivatives to minimize loss functions. A solid grasp of these mathematical principles allows programmers to design better models and improve their performance.
Optimization Techniques in AI
Optimization is essential in AI to enhance the performance of algorithms. It involves finding the best solution from a set of possible solutions, often framed as minimizing or maximizing functions. Understanding mathematical optimization techniques helps in refining machine learning models significantly.
Some common optimization techniques include:
- Gradient Descent: An iterative method for finding minimum values of functions. It adjusts model parameters to minimize loss.
- Newton's Method: A second-order optimization technique that uses second derivatives to find stationary points more efficiently.
- Stochastic Optimization: Involves randomness and helps in reducing overfitting by updating model parameters more frequently.
The capacity to optimize algorithms contributes significantly to the efficiency and accuracy of AI applications.
Finale: The Indispensable Role of Mathematics in Programming
The importance of mathematics in programming cannot be overstated. It serves as the backbone for developing efficient algorithms and robust software. In this conclusion, we will highlight several specific elements that illustrate why mathematics is indispensable for programmers.
First, understanding mathematical principles aids in creating optimized algorithms. Algorithms rely on mathematical models to solve problems, analyze data, and execute tasks. For example, sorting algorithms like QuickSort utilize concepts of complexity that are deeply rooted in mathematics. Knowledge of Big O notation helps programmers comprehend how their solutions scale as input sizes change.
Second, mathematics enhances problem-solving skills. By framing programming challenges within mathematical contexts, programmers can devise effective and innovative solutions. The process of translating real-world problems into mathematical language is often the first step in finding a computational solution.
Lastly, mathematical concepts are pivotal in emerging fields such as artificial intelligence and machine learning. These domains leverage advanced mathematical techniques, such as linear algebra and calculus, to enable computers to learn from data. Without a solid foundation in these areas, it is challenging to develop effective models or understand their limitations.
"Mathematics is not just a tool for coding; it is the very language of logic that guides us through complex programming challenges."
In summary, the intersection of mathematics and programming provides a rich tapestry of tools and frameworks necessary for effective software development. An understanding of mathematical foundations is not simply beneficial; it is essential for navigating the complexities of todayβs programming landscape.
Recap of Key Mathematical Concepts
Let's briefly recap the key mathematical concepts discussed throughout the article:
- Arithmetic Operations: Fundamental operations that are the building blocks of more complex calculations.
- Algebraic Concepts: Equations, variables, and constants form the basis for writing code that manipulates data.
- Functions: Both in mathematics and programming, functions represent specific relationships between inputs and outputs.
- Discrete Mathematics: The study of structures that are fundamentally discrete rather than continuous, which is essential for computer science.
- Calculus: Used primarily in optimization problems and understanding changes over time in algorithms.
- Probability and Statistics: Crucial for making decisions based on data and improving algorithms through data analysis.
- Graph Theory: Important for understanding networks, resource allocation, and efficient pathfinding algorithms.
This knowledge provides a comprehensive framework for effective programming and helps in tackling various challenges in software development.
Future Directions for Learning
As the landscape of programming evolves, so too must the mathematical skills of programmers. Here are some suggested directions for further learning:
- Deepen Understanding of Advanced Topics: Topics such as linear programming and game theory become increasingly relevant in data science and AI.
- Explore New Mathematical Fields: Areas like cryptography rely heavily on number theory and abstract algebra. Familiarizing oneself with these concepts can open doors to careers in cybersecurity.
- Apply Mathematics to Real-World Problems: Engage in projects that use mathematical models to solve practical problems. Participate in hackathons or coding challenges that emphasize algorithmic efficiency and mathematical reasoning.
- Stay Updated with Educational Resources: Online platforms like Coursera and Khan Academy provide various courses on math-oriented programming topics. Engaging with forums on Reddit or open discussions on platforms like Facebook can also enhance learning through community insights.
By pursuing these directions, programmers can ensure their skill set remains relevant and robust, contributing to their success in a competitive job market. The importance of mathematics in programming extends far beyond the classroom; it is a lifelong journey of learning and growth.