Exploring Shortest Path Algorithms in Graphs
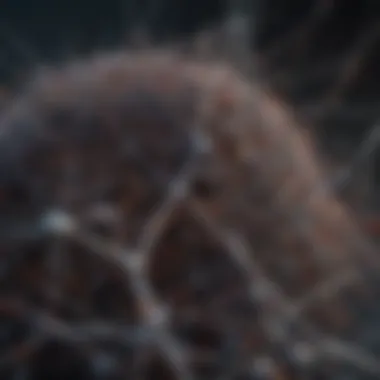
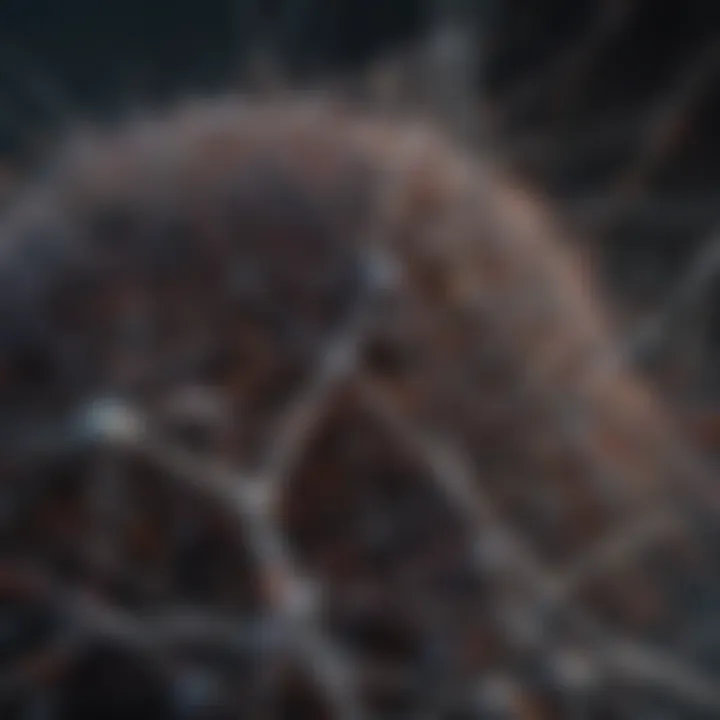
Intro
Graph structures play a vital role in the way we understand and analyze connections within various systems. They appear in diverse fields such as computer science, transportation, and social network analysis. The challenge of finding the shortest path in these graphs is significant because it can directly affect resource efficiency, speed of operations, and overall outcomes in projects ranging from delivery logistics to algorithm design.
Graph theory has grown to be a fundamental aspect of many industries. This article aims to dissect the methodologies behind finding the shortest path in graph structures, emphasizing algorithms that are instrumental in solving real-world problems.
Through a detailed exploration of the key algorithms, their complexities, applications, and practical examples, this article provides a bridge between theoretical concepts and their real-life implications. The reader will gain an understanding of how these algorithms operate and can be utilized in various domains, enhancing their ability to navigate the complexities of graph structures effectively.
Prelims to Graph Theory
Graph theory serves as the backbone for understanding relationships and pathways in various systems. It lays the foundation for analyzing connections, simplifying complex structures into manageable models, and allows for efficient problem-solving. This section dives into the essentials of graph theory, highlighting its significance in various fields from computer science to logistics. Understanding graphs is crucial, as they reveal the dynamics of interconnected data.
Definition of a Graph
A graph is a mathematical concept comprised of vertices and edges. Vertices represent the individual points, while edges depict the connections between these points. By studying graphs, one can deduce valuable insights about connectivity and accessibility within a network. This definition is fundamental as it encapsulates the basic elements that will be built upon in subsequent discussions regarding pathfinding algorithms.
Components of a Graph
Graph structures are simple yet profound, consisting of several key components that play critical roles in their functioning.
Vertices
Vertices, or nodes, are the fundamental units of a graph. Each vertex symbolizes an entity, whether it be a city in a transportation map or a user in a social network. The beauty of vertices lies in their versatility; they can represent a variety of objects or information depending on the context. Their key characteristic is the ability to connect, which allows for seamless navigation through the graph. Vertices are beneficial due to their simplicity and adaptability, enabling models that can represent diverse systems. However, they may also lead to challenges in distinguishing between similar entities where additional identifiers may be necessary.
Edges
Edges are the connectors that establish relationships between vertices. They define how vertices interact and the nature of their connection. Without edges, vertices would remain isolated points without context. A key characteristic of edges is their directional nature; they can be directed or undirected, indicating whether the connection is one-way or mutual. The presence of edges facilitates pathfinding within the graph. However, relying heavily on edges can complicate the visualization of more complex graphs, as dense connections can obscure important information.
Weighted and Unweighted Graphs
Graphs can be classified as either weighted or unweighted, based on whether edges have assigned values. Weighted graphs assign a cost to the edges, allowing for more nuanced calculations when determining the shortest path. This characteristic is essential for practical applications such as logistics and network routing. Conversely, unweighted graphs treat all connections equally, simplifying calculations but potentially overlooking valuable details about the paths. Understanding this distinction is vital, as it influences the choice of algorithms used for finding the shortest path.
"Graph theory provides a framework for understanding complex relationships and is fundamental in developing solutions across various domains."
Through this exploration of basic graph theory, readers can gain insight into how these elements interact. This foundational knowledge prepares readers for more detailed discussions on shortest path algorithms and their real-world applications.
Understanding the Shortest Path Problem
The shortest path problem is a fundamental challenge in graph theory. Its understanding is crucial as it has significant implications across various fields, including computer science, logistics, telecommunications, and navigation systems. By identifying the most efficient route or path between two points in a graph, we enhance operational efficiency and decision-making.
What is the Shortest Path Problem?
The shortest path problem involves finding the minimum distance or cost to travel from a starting point to a destination within a graph. Each edge in the graph may have a weight that represents distance, cost, or time, and the goal is to calculate the path with the least total weight.
The problem can be represented mathematically, and lays the groundwork for several algorithms that facilitate solutions. This concept is not limited just to physical distances. It extends to various scenarios in data networks, urban planning, and even social networks. Understanding this problem opens pathways to problem-solving in more complex situations encountered in real-world applications.
Applications of Shortest Path Solutions
Shortest path solutions find extensive applications in various domains. A brief overview of some significant sectors includes:
Transportation Networks
Transportation networks present a tangible application of the shortest path problem. This can involve roadways, railways, and air routes. The main contribution here is optimizing travel times and reducing operational costs in logistics and public transport.
The key characteristic of transportation networks is their dynamic nature. Routes can change due to traffic conditions, weather, and other real-time factors. This characteristic makes it a popular choice for analysis in this article. One unique feature is the use of identifiable nodes like intersections and junctions.
While these networks offer efficiency in route planning, they also have disadvantages, such as the potential for congestion and delays that may not be easily encapsulated within static graph structures.
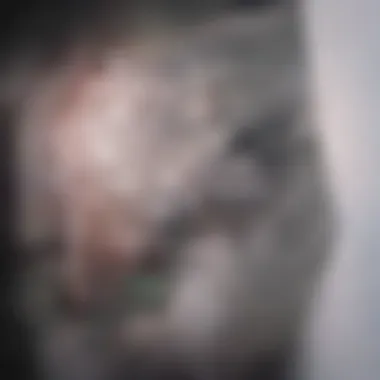
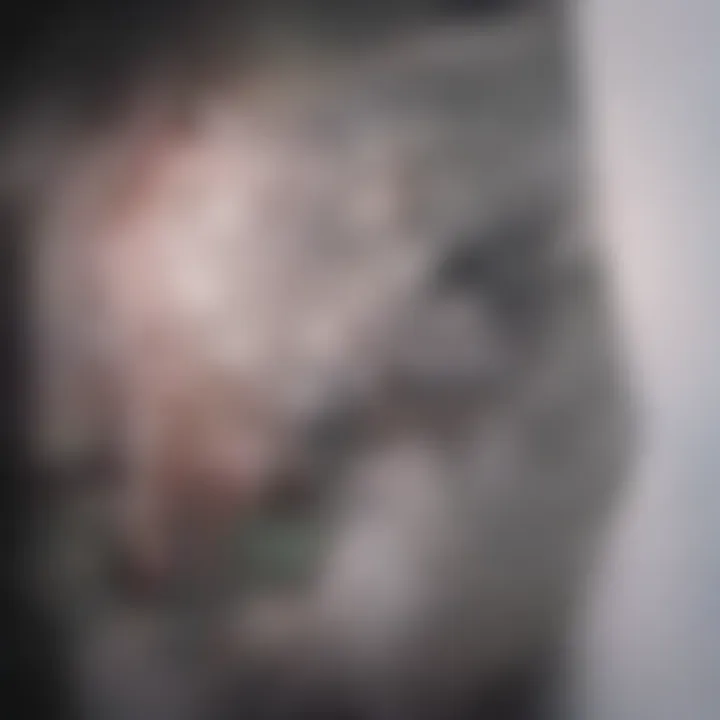
Telecommunication Systems
In telecommunication systems, the shortest path problem aids in optimizing data transmission. Data must navigate through a network of servers and switches, which can be represented as a graph. The contribution here is significant, as it enhances the speed and reliability of communication services.
The essential characteristic of telecommunication systems is their vast interconnected networks, where minimizing delays and maximizing throughput are critical. This aspect makes them a relevant component for exploring shortest path solutions. One unique feature of these systems is their ability to adapt in real-time to changes in network usage.
However, these systems can face challenges like network congestion and signal loss, which can complicate the shortest path calculations.
Navigation Systems
Navigation systems, such as GPS, directly address the shortest path problem to provide users with the most efficient routes for travel. This is an area of technology that benefits everyday life significantly. The main contribution of navigation systems is to simplify travel, delivering clear directions and eliminating confusion.
A notable characteristic of navigation systems is their reliance on real-time data. This means that routes can be recalibrated dynamically based on traffic, construction, or other obstacles. This makes navigation systems very effective for users. However, while they provide convenience, they can also lead to dependency on technology, causing potential issues if systems fail or data is inaccurate.
In summary, understanding the shortest path problem and its applications in transportation networks, telecommunications, and navigation systems illuminates its practical relevance. By exploring these applications, we gain insights into the broader impact of shortest path algorithms on society.
Common Algorithms for Finding Shortest Paths
Finding the shortest path in graph structures is a fundamental problem in various fields such as computer science, logistics, and network design. The algorithms designed to tackle this issue play a crucial role in optimizing processes, reducing costs, and improving efficiency. Each algorithm has its own strengths and weaknesses, making it important to understand which one best fits a given situation. This section will explore three prominent algorithms: Dijkstra's, Bellman-Ford, and A* search, detailing their approaches and applications.
Dijkstra's Algorithm
Algorithm Overview
Dijkstra's algorithm is one of the most widely used methods for finding the shortest path in weighted graphs. It operates by initiating from a starting node and expanding out, at each step choosing the node that is nearest based on current known distances. The algorithm's greedy approach allows it to efficiently find the minimum cost path. Its major characteristic is that it only works with non-negative weights, which simplifies computations but limits its versatility. The popularity of Dijkstraβs algorithm stems from its clear mathematical foundation and effectiveness in most scenarios, though it faces challenges in dynamic graphs where weights might change over time.
Step-by-Step Implementation
The implementation of Dijkstra's algorithm involves various steps: setting the initial node distance to zero while assigning infinity to all others, selecting the node with the smallest distance, and updating the path costs for its neighbors. By repeating these steps until all nodes are processed, the algorithm efficiently identifies the shortest path. This systematic approach ensures that every node is evaluated based on the most current distance information, creating clarity in the traversal process. However, the algorithm becomes inefficient on sparse graphs where a linear search for the minimum distance can slow performance.
Time Complexity Analysis
The time complexity of Dijkstra's algorithm is often stated as O(V^2), where V is the number of vertices. However, using a priority queue can reduce it to O(E log V), making it much more feasible for dense graphs. This time efficiency is a significant characteristic that highlights why Dijkstraβs is preferred for many applications. Despite its strength, the fixed time complexity can pose issues in extensive graphs or real-time systems that require dynamic updates.
Bellman-Ford Algorithm
Algorithm Overview
The Bellman-Ford algorithm stands out due to its ability to handle graphs with negative weight edges. It can effectively detect negative cycles, which can be a crucial aspect in many applications, such as financial models where costs can fluctuate. This flexibility is its key feature, providing a broader application range compared to Dijkstra's algorithm. However, it operates with a higher time complexity, which can limit its usefulness in scenarios that demand quick responses.
Use Cases for Negative Weights
A notable application of the Bellman-Ford algorithm is in the detection of arbitrage opportunities in currency exchange systems. The algorithm identifies potential profit paths despite the presence of negative weights. This unique feature makes it beneficial for financial institutions. Additionally, its ability to handle negative weights provides a valuable perspective in scenarios where costs are uncertain or can vary significantly.
Time Complexity Insights
In terms of time complexity, the Bellman-Ford algorithm operates at O(VE), which can be seen as a disadvantage in very large graphs. This performance, while adequate for many uses, raises concerns when scalability becomes a necessity. The time complexity requires careful consideration when choosing this algorithm, especially in applications that demand efficiency.
A Search Algorithm
Algorithm Principles
A* search algorithm integrates heuristic evaluation with distance costs to find the shortest path. This heuristic approach allows for more efficient traversal, as it directs the search toward the target node, reducing unnecessary evaluations. A key characteristic of A* is its adaptability to various heuristics, which can be fine-tuned according to specific needs in different contexts. This customization ability contributes to its popularity in practical applications, although it requires careful selection of the heuristic to ensure accurate results.
Comparison with Dijkstraβs Algorithm
When comparing A* with Dijkstraβs algorithm, one can see that while Dijkstra's focuses solely on the shortest distance, A* uses both distance and heuristic values. This dual consideration can make A* faster and more efficient in certain scenarios, especially in large graphs with clearly defined goals. Nevertheless, the complexity of A* can make its implementation more challenging, depending on the effectiveness of the heuristic employed.
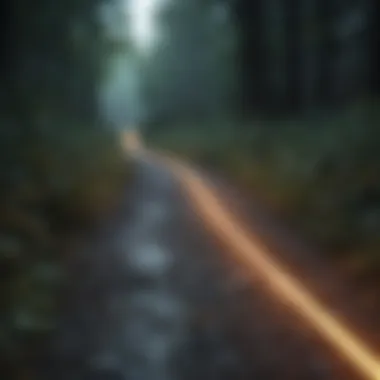
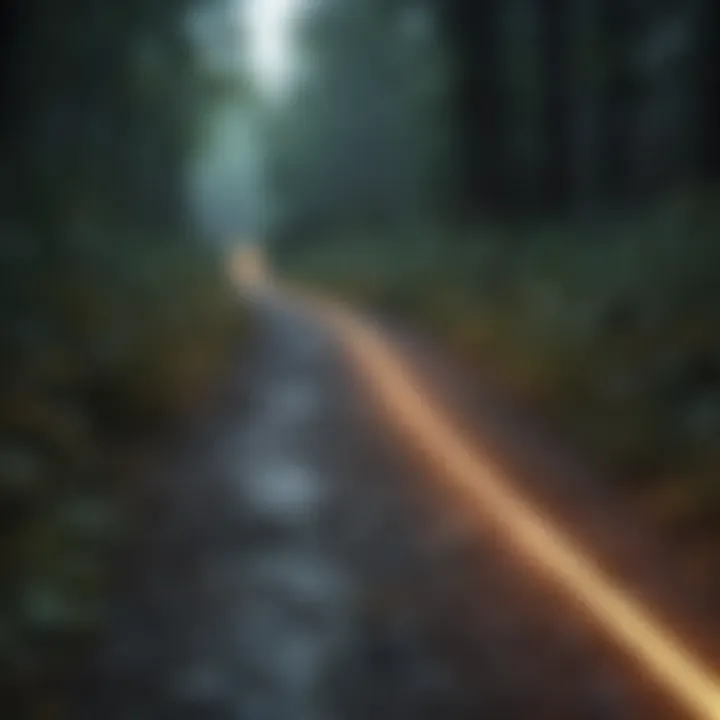
Practical Applications
A* is widely employed in pathfinding for video games and AI, where efficiency is paramount. Its ability to navigate complex environments while prioritizing pathways that lead toward goals makes it especially useful in real-time applications. The flexibility of A* allows developers to implement various strategies, adapting the algorithm to suit distinct requirements in diverse fields like robotics and computer graphics.
Graph Representation Techniques
Graph representation techniques are critical in the realm of graph theory and pathfinding algorithms. They serve to translate abstract graph structures into formats that computer systems can process efficiently. Understanding how to represent graphs accurately allows for effective realization of algorithms such as Dijkstra's or A*. The choice of representation affects both the performance of these algorithms and the ease of accessing graph components. This section will explore two primary graph representation techniques: adjacency matrix and adjacency list. Each has its strengths and weaknesses, suitable for different scenarios in the context of shortest path problems.
Adjacency Matrix Representation
An adjacency matrix is a two-dimensional array where each entry indicates whether a pair of vertices is connected by an edge. This representation is straightforward and has its own set of advantages.
Advantages
The adjacency matrix is beneficial for dense graphs. It allows for quick access to check if there is a connection between two vertices. The key characteristic here is the simplicity of lookups; accessing the relationship between vertices can be done in constant time, O(1). This efficiency enables algorithms that rely heavily on vertex connectivity to perform optimally. A unique feature is that all edges are represented, so modifications such as adding or removing edges are also simple operations. However, they consume more memory compared to other representations, which can be considered a downside in sparse graphs.
Disadvantages
The adjacency matrix has its limitations, particularly when used for sparse graphs. Due to its square nature, memory requirements grow significantly with the number of vertices. It is not efficient in terms of space for graphs with a low number of edges relative to the number of vertices. The unique aspect of this representation that poses a disadvantage is that even when many vertex pairs are disconnected, the matrix will still utilize space to indicate non-existent connections. As a result, in such cases, adjacency matrices may not be the most practical choice.
Adjacency List Representation
An adjacency list, on the other hand, stores each vertex and lists all adjacent vertices. This method generally consumes less space and caters well to sparse graphs.
Advantages
The adjacency list is particularly effective for representations of sparse graphs. Its main advantage lies in its space efficiency. The key characteristic here is that it utilizes only as much memory as is necessary to store the edges. This makes it a popular choice for many applications where graphs are large but have relatively few edges. Each vertex can be quickly traversed, which benefits shortest path algorithms. The concise structure allows for faster operations in various implementations, such as depth-first or breadth-first search.
Disadvantages
Despite its benefits, the adjacency list has its shortcomings. Accessing whether two vertices are connected may take longer, about O(n) in the worst-case scenario, since it requires checking through the list of adjacent vertices. This inefficiency can become a bottleneck in dense graphs where numerous connections exist. The unique drawback is that additional complexity is involved in graph modification procedures. Adding or removing edges may require iterating through lists, which adds overhead.
Understanding both advantages and disadvantages of these two techniques forms the groundwork for choosing the most suitable representation for a given application. Ultimately, selection depends on the specific characteristics of the graph involved, such as density and required operations.
Real-World Case Studies
Exploring real-world case studies is essential in understanding how theoretical concepts translate into practical applications. Case studies demonstrate the effectiveness and relevance of shortest path algorithms in everyday scenarios. For professionals, students, and researchers, seeing these algorithms in action clarifies their potential and operational value across different sectors.
Shortest Path in GPS Navigation
GPS technology heavily relies on efficient shortest path algorithms. When you input a destination, the system must quickly calculate multiple potential routes to find the optimal path. This is where algorithms like Dijkstraβs or A* become crucial. They evaluate various points on the map, considering factors such as distance, travel time, and even traffic conditions.
Key Considerations in GPS Navigation:
- Real-time Data Evaluation: Algorithms must process real-time information about traffic to offer the best routes.
- Dynamic Route Adjustment: Users often need immediate rerouting. Algorithms need to accommodate changing conditions seamlessly.
- User Preferences: Different users may prioritize different criteria, such as shortest distance or least time.
The combination of these elements illustrates the complexity of shortest path calculations in GPS systems, highlighting their critical role in modern navigation.
Route Optimization in Logistics
Logistics is another field where shortest path algorithms are indispensable. In supply chain management, companies must optimize delivery routes to minimize costs and improve efficiency. Algorithms help determine the best way to transport goods from one location to another, significantly impacting operational performance.
Efficiencies Gained Through Route Optimization:
- Cost Reduction: By finding the shortest and most efficient routes, companies save on fuel and labor costs.
- Time Management: Routes can be tailored to ensure timely deliveries, essential for maintaining customer satisfaction.
- Resource Allocation: Understanding optimal paths allows for better planning and use of transportation resources.
In logistics, applying shortest path algorithms can lead to profound improvement in overall performance. As industries evolve, their reliance on these computational methodologies will only grow.

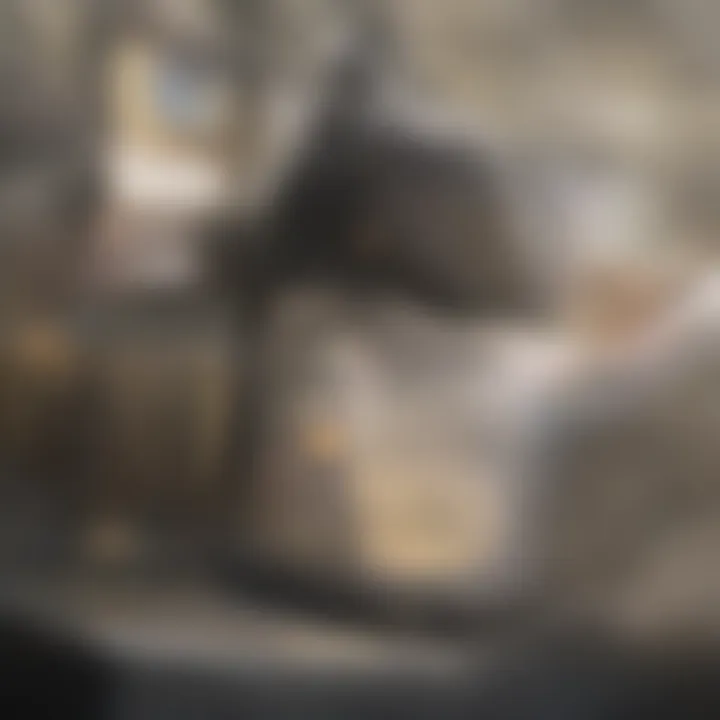
Challenges in Shortest Path Algorithms
The topic of challenges in shortest path algorithms is crucial to understand as it informs researchers and practitioners of potential pitfalls and considerations in real-world applications. As graph structures evolve, traditional shortest path algorithms may face limitations, requiring adaptation and innovation. Key factors revolve around graph dynamism and scalability, both of which play pivotal roles in the effectiveness of routing solutions. Addressing these challenges is essential in harnessing the full potential of shortest path algorithms in various fields, including transportation, telecommunications, and network management.
Handling Dynamic Graphs
Dynamic graphs represent situations where the structure of the graph changes over time. Edge weights can fluctuate, vertices may be added or removed, and entire pathways might become obsolete. This variability introduces significant challenges when attempting to find the most efficient route. Traditional algorithms, such as Dijkstra's, typically operate on static graphs, making them less suitable for scenarios where conditions continuously change.
A dynamic graph requires the use of specialized algorithms or adaptive strategies that can accommodate these fluctuations. An example of this is the use of incremental algorithms, which build upon previous results to quickly adjust to graph changes without recomputing from scratch. By doing this, they can significantly reduce computational overhead and improve response times. This process is vital in applications like traffic systems or real-time logistics, where quick decision-making is essential.
Scalability Issues
Scalability is another prominent challenge in shortest path algorithms. As the size of datasets increases, both in terms of the number of vertices and edges, many algorithms struggle to maintain efficiency. For large-scale graphs, such as those encountered in social networks or geographic information systems, an algorithm that works well for smaller datasets may become infeasible and time-consuming.
To address scalability, approaches such as partitioning graphs into subgraphs have been proposed. This method allows for simpler calculations within manageable segments while maintaining an understanding of the entire graphβs architecture. Furthermore, heuristic algorithms like the A* search can be deployed to make educated guesses about the most promising paths, reducing the search space effectively.
Future Directions in Graph Pathfinding
The exploration of future directions in graph pathfinding is crucial for several reasons. As technology and methodologies evolve, the demand for more efficient and adaptable algorithms in various fields increases. Both machine learning and quantum computing are at the forefront of this development, promising significant advances in how we navigate graphs and find solutions to shortest path problems. \n
Incorporating innovative techniques can enhance the efficiency of existing algorithms and create opportunities for solving previously intractable problems. This not only fuels academic research but also has tangible implications for industries relying on these technologies, such as logistics, telecommunications, and transportation. Embracing these future directions will allow for more robust solutions tailored to complex challenges that arise in real-time scenarios. \n
Impacts of Machine Learning
Machine learning stands as a transformative component in graphs and pathfinding methodologies. By integrating adaptive learning algorithms, researchers can develop systems that not only analyze patterns but also predict and adapt to changing conditions within a graph. This capability is particularly advantageous when dealing with dynamic graphs, where nodes and edges can change over time. \n
Machine learning can facilitate real-time optimization. For instance, algorithms can learn from historical data to make informed decisions about pathfinding more efficiently. Some key benefits include:
- Improved decision-making based on predictive analysis.
- Enhanced accuracy through continuous learning from data.
- Increased speed in processing large datasets, which is critical in large-scale applications. \n
Although promising, the implementation of machine learning techniques in graph theory also requires careful consideration. Challenges like data availability, the complexity of model training, and the interpretability of results must be addressed to realize full potential. \n
Quantum Computing Implications
Quantum computing introduces a paradigm shift in computational capabilities. When applied to graph pathfinding, it has the power to revolutionize how we approach problems that require significant computational resources. Problems that once took hours or days to solve could see dramatic reductions in time. Quantum algorithms leverage superposition and entanglement, which can vastly improve the efficiency of searches. \n
One of the more notable quantum algorithms that could affect shortest path problems is Grover's algorithm, designed for unstructured search problems, thus optimizing the pathfinding process. The implications include:
- The ability to process complex graphs at unprecedented speeds.
- Opportunities for real-time decision-making in critical applications, such as autonomous driving or network optimization.
- A potential reduction in computational cost for organizations, beneficial for start-ups and research institutions alike. \n
However, much of the required infrastructure and understanding around quantum computing is still developing. Continued research is necessary to overcome these barriers and make quantum solutions practical. \n
"The next decade will be critical for advancing graph algorithms through innovative computational techniques, influencing various sectors significantly."
Finale
The conclusion of this article encapsulates the various elements of finding the shortest path in graph structures. Understanding these concepts is not merely an academic exercise. It holds significant implications across a range of practical applications. The methodologies discussed empower developers, researchers, and industries to optimize their processes, leading to better decision-making and resource allocation.
Summary of Key Insights
This article has highlighted several critical themes:
- Graph Structures: The foundational elements of graphs, including vertices and edges, are crucial for understanding the dynamics of various systems.
- Shortest Path Algorithms: We explored key algorithms such as Dijkstra's, Bellman-Ford, and A*, illustrating their specific contexts and performance characteristics.
- Real-World Applications: From navigational systems to logistics optimization, the relevance of these algorithms in real-world scenarios is evident.
- Challenges and Future Directions: Addressing the limitations of current algorithms offers a pathway for innovation, especially when considering dynamic environments and the potential of emerging technologies like machine learning and quantum computing.
This summary provides a cohesive overview, emphasizing that the quest to find the shortest path is not just a technical challenge but a necessary endeavor across diverse fields.
The Importance of Continued Research
Continued research in the field of graph theory and shortest path algorithms is essential for several reasons. First, as the complexity of networks increases, new methods and improved algorithms are required to handle larger datasets efficiently. The advent of big data creates a pressing need for algorithms that can adapt to changing conditions in real-time.
Moreover, exploring intersections between graph theory and other domains, like artificial intelligence and machine learning, can yield innovative solutions. This interdisciplinary approach can enhance algorithmic performance and expand their functionality.
Ultimately, investing resources into the ongoing study of shortest path problems can lead to breakthrough advancements, enhancing not just theoretical knowledge, but also practical applications that benefit businesses, urban planning, and technology development.