Essential Mathematics for Computer Science Success
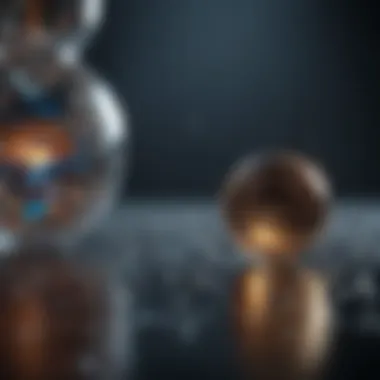
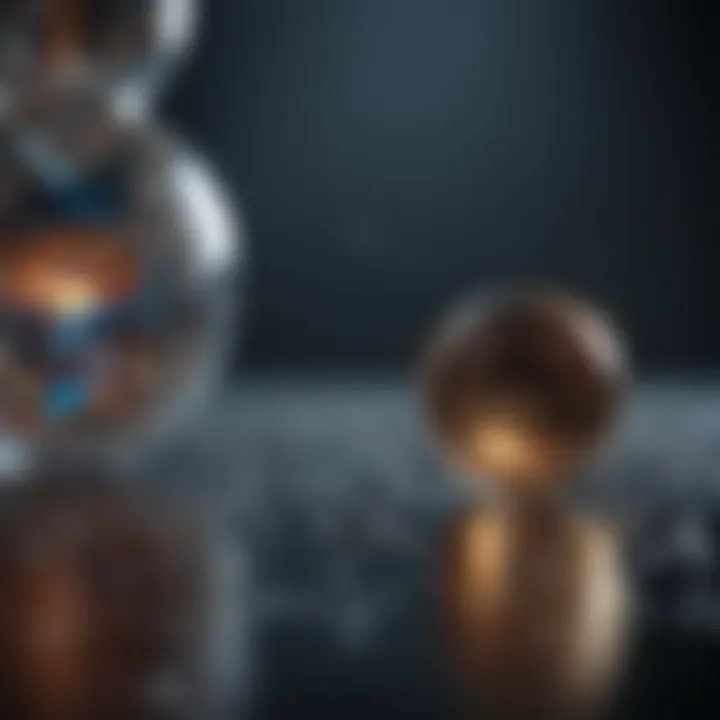
Intro
Mathematics serves as the foundational bedrock for computer science. Many people may not recognize the deep ties between mathematical concepts and computing tasks, but understanding these connections is crucial. As computer science evolves, so does the necessity for a solid grip on core mathematical ideas.
Within this article, we will discuss the essential branches of mathematics that influence programming, algorithm design, and data structures. By grasping these concepts, students, researchers, and professionals can hone their skills and approach problems with a well-equipped mindset. Letβs navigate through these necessary topics, making the theoretical underpinnings clear and practical applications apparent.
Prelude to Mathematics in Computer Science
Mathematics serves as the backbone of computer science. Without a solid foundation in mathematical concepts, understanding algorithms, data structure, and coding can be quite challenging. This section emphasizes the importance of mathematics and provides an overview of key disciplines that are crucial for success in computer science.
Importance of Mathematics in Computer Science
Mathematics is not merely an abstract concept, it has practical applications in computer science. It fosters problem-solving skills and logical thinking. Here are several ways in which mathematics holds importance in this field:
- Framework for Algorithms: Algorithms rely on mathematical principles for their formulation. Understanding the math behind algorithms ensures they are efficient and optimize performance.
- Data Structures: Basic data structures such as arrays, lists, and trees often have mathematical properties that aid in their implementation and application.
- Machine Learning: Machine learning, a significant area in AI, requires advanced mathematical concepts such as calculus, statistics, and linear algebra for model construction and optimization.
- Cryptography: Secure systems rely heavily on mathematical principles. Understanding number theory and probability can enhance one's ability to develop secure algorithms.
In short, mathematics cultivates a sharper analytical mind, making it invaluable in computer science.
Overview of Key Mathematical Disciplines
Various mathematical disciplines form the core of computer science. Familiarity with the following is essential:
- Discrete Mathematics: It covers topics like set theory, logic, combinatorics, and graph theory. Each topic provides unique tools to understand algorithms and programming.
- Linear Algebra: Involves studying vectors and matrices, crucial for transformations in graphics and machine learning algorithms.
- Calculus: Focuses on rates of change, which is fundamental for understanding optimization problems in algorithms.
- Probability and Statistics: These disciplines help in data analysis and modeling uncertainties in algorithms, especially useful in AI and machine learning.
- Numerical Methods: They offer techniques for solving mathematical problems that arise in statistics, engineering, and algorithm design, including approximation methods.
Understanding these mathematical areas enriches oneβs capability in computer science. By merging theory with practical application, students and professionals can better navigate the complexities of this field.
Discrete Mathematics
Discrete mathematics forms a critical foundation in computer science. It emphasizes structures that are fundamentally countable or otherwise distinct and not continuous. Unlike calculus, which deals with continuous change, discrete mathematics focuses on objects such as integers, graphs, and logical statements. Its relevance spans various aspects of computer science, particularly in algorithms, software development, and data structures.
Set Theory and Logic
Set theory serves as the backbone of discrete mathematics. It involves the study of sets, which are collections of objects. In computer science, every data structure can be represented as a set, making this concept essential for understanding databases, data manipulation, and more.
Logic complements set theory by providing tools for reasoning and proof. Propositional and predicate logic offer a framework to derive conclusions based on given statements. Hence, programming languages often embed logical expressions, forming the basis for conditions and loops that control program flow.
Combinatorics
Combinatorics is concerned with counting, arranging, and grouping. Its significance lies in determining the number of ways in which a set can be arranged or grouped. This plays a vital role in algorithm optimization. For instance, the efficiency of search algorithms can often be improved by understanding combinatorial principles. In network theory, combinatorial techniques help analyze and design effective structures.
Graph Theory
Graph theory studies graphs, which are comprised of vertices and edges. It is crucial in representing relationships and networks. In computer science, applications of graph theory are vast. These include network analysis, social media connections, and even in artificial intelligence algorithms like those used in searching or pathfinding. Solving problems related to connectivity and routes demonstrates clear advantages in practical scenarios like optimizing data routing paths in networks.
Algorithms and Complexity
Understanding algorithms is central to computer science. Discrete mathematics helps define algorithms in a rigorous manner. Algorithms are step-by-step procedures to solve problems, and their efficiency is critical. The complexity of these algorithms is expressed using concepts from discrete mathematics, particularly in big O notation, which categorizes algorithms based on their performance characteristics. This analysis aids programmers in selecting or designing efficient algorithms for various tasks.
Linear Algebra
Linear algebra plays a vital role in computer science, providing a framework to understand complex data structures and the transformations applied to them. Its relevance is especially prevalent in areas like computer graphics, machine learning, and data analysis. Matrices and vectors serve as foundational tools for representing and manipulating data.
Vectors and Matrices
Vectors and matrices are two fundamental concepts in linear algebra. A vector represents a quantity with both direction and magnitude. In computer science, vectors can be used to represent various data forms such as points in space, user ratings in recommendation systems, or even features for machine learning models. Matrices, on the other hand, are essentially collections of vectors. They can represent datasets, transformation functions, or systems of linear equations. Understanding how to operate on these entities is crucial for developing efficient algorithms and data structures.
Transformations and Eigenvalues
Transformations are operations that can be performed on vectors and matrices. They can be linear transformations or more complex mappings. Eigenvalues, associated with eigenvectors, indicate how much a corresponding vector is stretched or compressed during a transformation. This concept is significant in areas such as principal component analysis (PCA), which reduces the dimensionality of large datasets and enhances computational efficiency in many applications. Understanding these calculations is key for tasks in graphics rendering, where transformations must be efficiently applied to create visual representations.
Applications in Computer Graphics
Linear algebra is essential in computer graphics. It facilitates the representation and manipulation of images, 3D modeling, and animations. Through transformations, one can scale, rotate, and translate objects within a scene. Matrices are often used to transform the coordinates of shapes, allowing developers to create dynamic and responsive graphics. Techniques like perspective projection, which allows a scene to appear three-dimensional on a two-dimensional screen, rely heavily on matrix calculations. Mastery of linear algebra thus empowers developers to leverage mathematics in creating visually compelling and interactive applications.
"Linear algebra serves as the backbone of computer graphics and machine learning, providing tools for data manipulation and representation."
Understanding the principles of linear algebra is pivotal for anyone working in computer science. The concepts of vectors, matrices, and transformations are not just theoretical but have direct, practical applications that enhance the functionality and efficiency of various computing tasks.
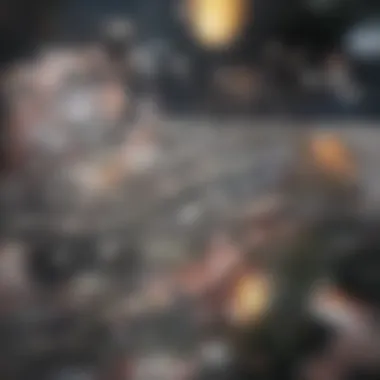
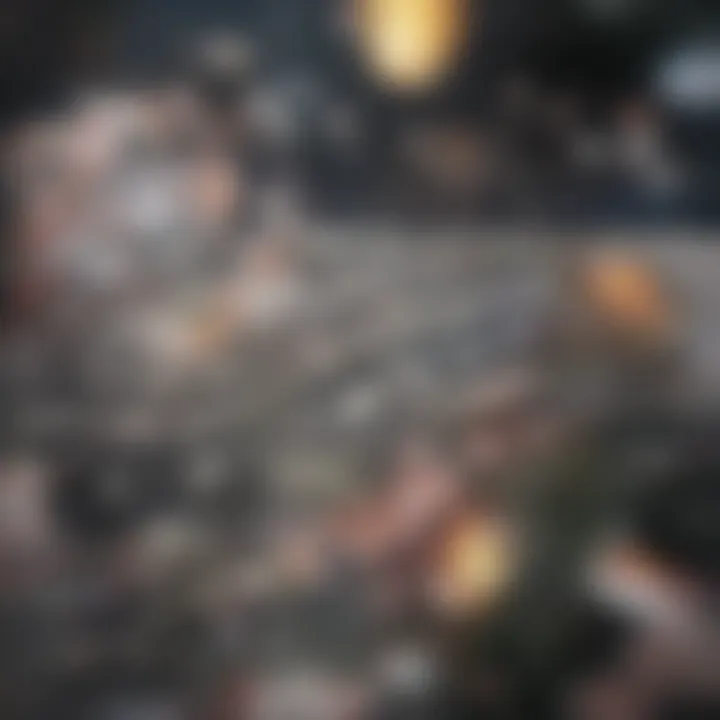
Calculus
Calculus is a branch of mathematics that plays a crucial role in many areas of computer science. Its concepts are essential for understanding changes and motion. Specifically, calculus provides tools to model and analyze dynamic systems, enabling programmers and researchers to understand phenomena like optimization and motion predictions. The methods of calculus, namely differentiation and integration, help in solving problems that involve rates of change and areas under curves. This makes it highly relevant in various applications, including algorithms, data analysis, and simulations.
Differentiation and Integration
Differentiation and integration are the two fundamental operations of calculus. Differentiation focuses on determining the rate at which a quantity changes. For computer scientists, this has applications in performance analysis and algorithm optimization. For instance, if one is analyzing the efficiency of an algorithm, differentiation can help find points of maximum or minimum performance and understand how changes in input size affect execution time.
Integration, on the other hand, computes the accumulated sum of quantities, which helps in understanding total quantities that change over time. For example, when dealing with continuous data streams in machine learning, integration can aid in determining overall trends within the data. Together, these concepts empower computer scientists to develop more efficient and responsive systems.
Understanding Continuous Functions
Continuous functions are a key aspect of calculus. A function is continuous if small changes in the input lead to small changes in the output. Understanding these functions is vital for modeling real-world phenomena in computer science. Continuous functions are often used in algorithms that involve approximation, such as interpolation techniques, which provide estimates of function values within certain ranges.
In programming fields, visualizing algorithms often involves continuous functions. For example, curve fitting and data smoothing require a firm grasp of how continuous functions behave. They help in representing complex relationships in a simpler form for analysis and computation. This foundational knowledge allows for building better predictive models and simulations.
Role in Machine Learning Algorithms
Calculus is fundamental to machine learning. Most algorithms used for training models heavily rely on calculus concepts, particularly differentiation. The process of optimizing machine learning models involves calculating gradients to adjust parameters, ensuring the model learns effectively from data.
Gradient descent, a popular optimization technique, directly employs differentiation to minimize error functions. Understanding how to manipulate functions through calculus helps data scientists and software developers to fine-tune their models. This relationship between calculus and machine learning not only enhances model performance but also deepens the understanding of model behavior under different scenarios.
"Calculus is the essence of mathematical modeling and optimization in computer science, particularly in machine learning."
In summary, calculus is a powerful tool that enhances computer science through its mathematical framework. Its principles of differentiation and integration allow for more effective algorithms and models in various fields, including data analysis, performance optimization, and machine learning.
Probability and Statistics
Probability and statistics are fundamental to understanding uncertainty and data analysis in computer science. These two areas provide the tools for making estimations and decisions based on data, which is crucial in algorithms that depend on varied datasets. In a world increasingly driven by data, grasping these concepts empowers students and professionals to derive actionable insights from information, influence decisions, and optimize computational models.
Fundamentals of Probability Theory
Probability theory serves as the backbone for statistical analysis. It allows for modeling uncertainty and making inferences about random phenomena. Essential concepts include:
- Random variables: Entities that take on values determined by chance.
- Probability distributions: Functions that specify the likelihood of different outcomes.
- Events and sample spaces: Basic constructs that form the foundation of probability calculations.
Understanding these concepts enables one to tackle real-world problems, such as noise reduction in signal processing or risk assessment in machine learning. For example, the application of Bayes' theorem, which combines prior knowledge with new evidence, illustrates how probability theory unfolds in practical scenarios.
Descriptive and Inferential Statistics
Descriptive statistics summarize data sets through measures such as mean, median, mode, and standard deviation. They provide a clear view of data, enabling quick assessments of trends and distributions. These statistics are vital for initial data exploration.
In contrast, inferential statistics allow for making predictions or generalizations about a population based on a sample. This branch includes hypothesis testing and confidence intervals. For instance, when launching a new product, testing a small market segment with inferential statistics can reliably indicate broader market responses.
- Common tools used:
- Histograms to understand data distribution.
- T-tests to assess differences between groups.
- ANOVA for examining multiple group averages.
Thus, both descriptive and inferential statistics play different yet complementary roles in computer science applications, from data analysis to machine learning performance evaluations.
Bayesian Statistics and Its Applications
Bayesian statistics merges probability with inference, allowing for continual learning through data. Unlike traditional statistics, it incorporates prior knowledge into the analysis, refining conclusions as more data becomes available. This adaptability is advantageous in numerous fields, including:
- Machine learning: Algorithms adapt as they encounter new data, improving accuracy over time.
- Recommendation systems: These systems leverage Bayesian methods to update user preferences dynamically.
- A/B testing: Bayesian inference helps analyze variants of web pages to determine which performs better.
"Bayesian approaches improve decision-making by providing a probabilistic interpretation of evidence."
Bayesian statistics provides powerful tools for addressing uncertainty, guiding decisions in situations where information continuously evolves.
Numerical Methods
Numerical methods are a fundamental component in the landscape of computer science, particularly in areas that require quantitative analysis and problem-solving techniques. These methods provide the mathematical framework needed to tackle complex problems that canβt be solved through analytical means. Understanding numerical methods allows computer scientists to develop algorithms that approximate solutions to various mathematical equations.
Importance of Numerical Methods
Numerical methods are vital in many applications, from engineering simulations to financial modeling. They allow for the approximation of solutions to problems where traditional methods fail or are impractical. This includes solving differential equations, optimization problems, and performing numerical integration.
The benefits of numerical methods include their versatility and robustness. They can handle various types of problems and often improve the performance of algorithms by providing faster computations. However, it is critical to recognize that the effectiveness of these techniques relies heavily on the specific algorithms used and the nature of the problem being addressed.
Approximation Techniques

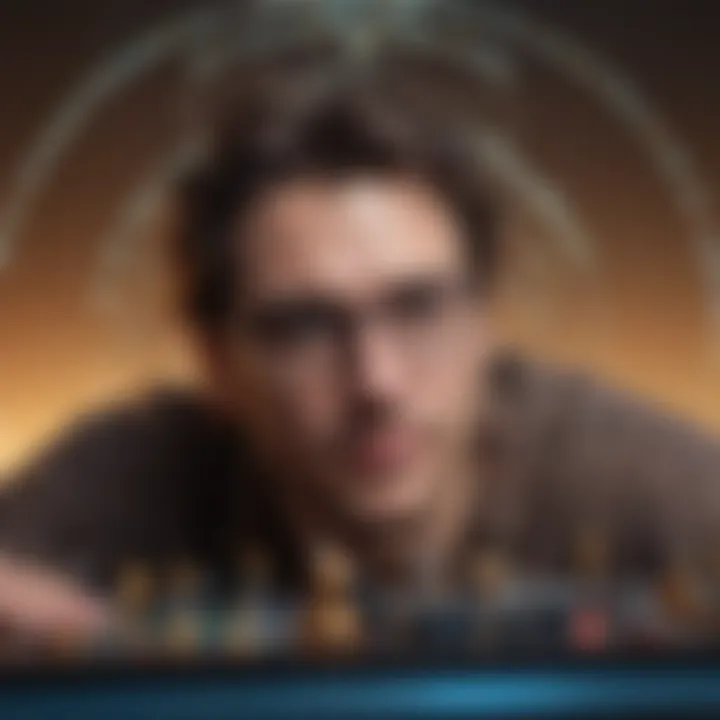
Approximation techniques form the underpinning of many numerical methods. These techniques are essential when an exact solution is not achievable via analytical methods. One common example is polynomial interpolation, where a complex function is approximated by a simpler polynomial function
In practice, approximation allows for the estimation of values of functions at a certain point based on known values at other points. The Taylor series expansion is another useful method for approximating functions near a specific point.
Key points about approximation techniques include:
- They provide significant simplification of complex problems.
- They allow for the analysis of continuous data sets in discrete systems.
- Error analysis in approximation is crucial to ensure the reliability of results.
Root Finding Algorithms
Root finding is a specific area within numerical methods that focuses on locating the roots of a functionβpoints where the function equals zero. This is particularly important in computer science for developing algorithms that require identifying thresholds or solutions to equations.
There are several well-known root finding algorithms, each with its own strengths and limitations. The bisection method is straightforward and guarantees convergence, making it suitable for simple applications. On the other hand, the Newton-Raphson method provides quicker convergence but may fail in certain situations, like when the initial guess isn't close enough to the actual root.
Common root finding algorithms include:
- Bisection Method
- Newton-Raphson Method
- Secant Method
- Fixed Point Iteration
Understanding these algorithms is crucial for software that involves computational mathematics, physics simulations, and engineering applications. The choice of the algorithm greatly impacts efficiency and accuracy in real-world computing scenarios.
"In numerical analysis, the quest for precision is a balance between computational feasibility and the inherent limitations of the methods employed."
Mathematics in Programming
Mathematics plays a critical role in programming. Understanding the mathematical concepts can lead to improved problem-solving skills, better algorithm design, and overall more efficient coding practices. The integration of math in programming is not just theoretical; it affects how software behaves in real-world applications. By grasping fundamental mathematical principles, developers can make informed decisions that enhance software performance and reliability.
Programming is often about devising solutions to complex problems. Mathematics provides tools for logical reasoning and abstraction necessary for programming challenges. From basic calculations to advanced algorithms, the influence of math is undeniable. Topics like graph theory and logic enable programmers to approach artificial intelligence, networking, and computational problems systematically.
In addition, mathematical rigor promotes a disciplined approach to coding. It encourages developers to think critically about their approach and optimize their code. This can lead to significant gains in execution speed and memory usage, which are crucial in professional environments where performance is paramount.
Mathematical Foundations in Software Development
Mathematical foundations serve as the bedrock for software development. Essential principles, including logic, set theory, and combinatorics, underpin the structure of programming languages and algorithms. For instance, boolean algebra forms the basis for logical operations in programming languages. Developers use these logical constructs in decision-making within their code.
Moreover, understanding mathematical reasoning enhances debugging. When you can manipulate and reason through algorithms mathematically, identifying flaws becomes easier. This is important in software development environments where time constraints frequently dominate.
"Mathematics is not about numbers, equations, or algorithms: it is about understanding."
Data Structure Efficiency Analysis
Efficiency in programming heavily relies on the choice of data structures. Here, mathematics comes into play. Different data structures offer varying speeds of operations, including insertion, deletion, and search. For example, a linked list allows for efficient insertions and deletions, but searching through it is slower compared to arrays.
Mathematics enables programmers to analyze these efficiencies using Big O notation. This mathematical concept provides a high-level understanding of how an algorithm performs relative to input size, which is vital for optimizing algorithms. Through this analysis, programmers can make informed decisions about which data structure to use in a specific context.
Additionally, analyzing algorithms mathematically highlights their time and space complexities. This aids in making trade-offs between memory usage and processing time. In scenarios involving large datasets, such efficiencies prove invaluable for ensuring quick and responsive applications.
Mathematical Logic in Computer Science
Mathematical logic is a fundamental aspect of computer science that provides a formal foundation for reasoning about algorithms, programming languages, and computational processes. It helps us understand how to structure arguments, analyze proofs, and verify the correctness of software. The techniques and principles from mathematical logic empower students and professionals to grasp complex concepts in computer science with a greater clarity.
The significance of mathematical logic can be observed through its contributions to crucial areas such as automated theorem proving, which allows computers to prove mathematical theorems automatically. With logic, one can represent facts and rules about the world in a manner that machines can process. This not only enhances the reliability of software systems but also supports advanced applications such as artificial intelligence.
Formal Proof Techniques
Formal proof techniques are essential tools used within mathematical logic. They provide a structured way to demonstrate the validity of propositions based on defined axioms and inference rules. Key techniques include:
- Natural Deduction: This method involves deriving conclusions from premises through a series of justified steps, ensuring that each inference made follows logically from the previous one.
- Proof by Contradiction: This technique assumes the opposite of what one intends to prove, leading to a contradiction, thereby confirming the original proposition.
- Induction: Often used in proofs about natural numbers and sequences, mathematical induction is a method that involves proving a base case followed by an inductive step that confirms the statement holds for all natural numbers.
These methods are crucial in ensuring program correctness. By providing a rigorous framework for reasoning, they highlight the importance of logical conclusions in the development and validation of algorithms.
Applications in Programming Languages
Mathematical logic also plays a vital role in the design and implementation of programming languages. Logic is embedded in the syntax and semantics of these languages. For example, logical assertions in languages like Prolog utilize formal logic to represent knowledge bases and perform queries.
Furthermore, many programming languages offer features that allow for strong type checking, ensuring certain logical properties hold before code execution. This is particularly significant in languages such as Haskell, where types are derived from logical propositions. The following points illustrate the relevance of mathematical logic in programming languages:
- Type Safety: Ensures that operations are performed on compatible data types.
- Formal Specifications: Aid in defining the behavior of software systems, allowing for formal verification methods to justify that a program meets its specification.
- Functional Programming: Languages like Lisp or Scala utilize mathematical routines that stem from logical principles, allowing developers to implement complex algorithms efficiently.
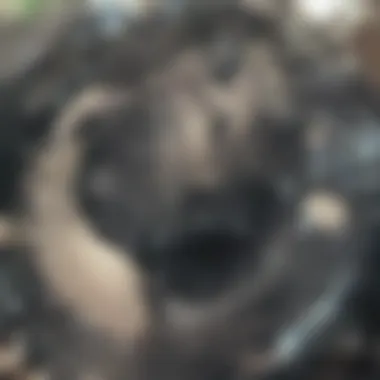
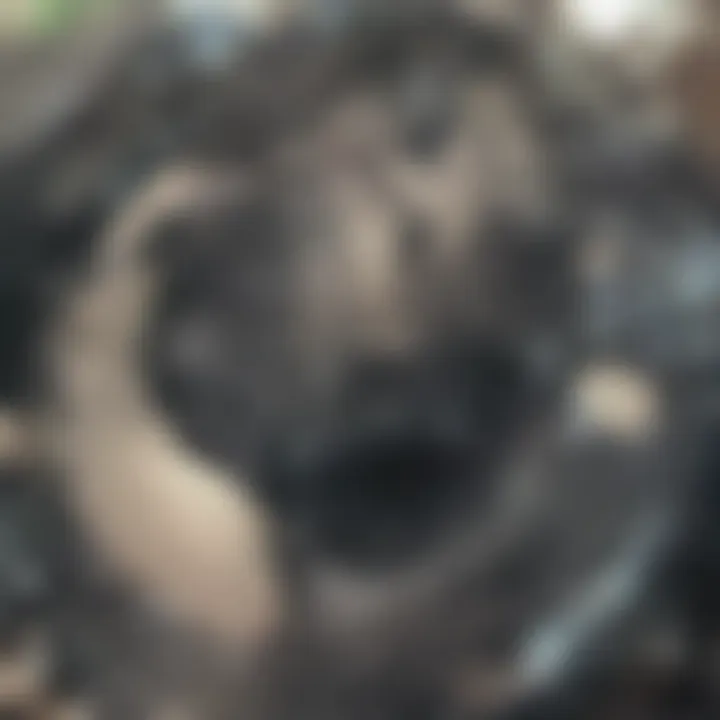
"Mathematical logic is not only about proving the truth of a statement but also about the framework that allows us to express computational theories clearly."
For more information on mathematical logic, you may visit Wikipedia.
Theoretical Computer Science
Theoretical computer science contains crucial concepts that serve as the foundation for understanding computing itself. This domain explores abstract models, computational processes, and their limitations, allowing us to grasp what can be computed, efficiently or not. It interconnects various fields such as logic, mathematics, and algorithm design. By understanding theoretical computer science, students and practitioners can appreciate why some problems are inherently difficult to solve and how to approach these challenges.
Turing Machines and Computability
Turing machines act as a fundamental model for computation, introduced by Alan Turing in the 1930s. This concept helps describe what it means for a function to be computable. Turing machines consist of a tape, which acts as infinite memory, a head that reads and writes symbols, and a state register to store the current condition of the machine. Thus, it captures the essence of algorithmic processes in a theoretical manner.
The relevance of Turing machines in computer science lies in their simplicity and power. They establish the limits of what can be achieved through computation, leading to the development of the Church-Turing thesis. This thesis posits that any computation performed by a Turing machine can be replicated by other computational models, which includes modern-day computers. Understanding Turing machines thus provides insight into the capabilities and limitations of both theoretical and practical computing.
The study of Turing machines is not just an academic exercise; it lays the groundwork for discussing undecidable problems, which have profound implications in algorithms and programming languages.
Complexity Classes and Reductions
Complexity classes categorize problems based on how difficult they are to solve, primarily in terms of time and space resources required. Notable classes include P (problems solvable in polynomial time) and NP (nondeterministic polynomial time). Understanding these classes aids in classifying algorithms according to their efficiency and performance.
Reductions are a crucial concept in complexity theory. They involve transforming one problem into another, allowing researchers to assess the difficulty of problems by comparing them. If a problem can be reduced to another known problem, it inherits the complexity characteristics of that target problem.
- Key points about complexity classes:
- They define boundaries for problems based on computational resources.
- They help in proving the hardness of specific problems.
- They guide the development and choice of algorithms in various applications like cryptography and optimization.
Applications of Mathematics in Real-World Computing
In computer science, mathematics plays a critical role in understanding and solving complex problems. The applications of mathematics are vast and include optimizing systems, validating results, and securing data. This section will examine specific areas where mathematics significantly impacts computing.
Mathematics in Cryptography
Cryptography relies heavily on mathematical principles. It provides the foundation for securing information online. Encryption methods, such as RSA and AES, use number theory and algebra extensively to protect data during transmission. The strength of these methods often depends on the difficulty of certain mathematical problems, like factoring large prime numbers.
The secure communication protocols we use today, such as HTTPS, utilize cryptographic algorithms to protect sensitive information. For example, when you enter personal data on a website, cryptography ensures that this data is transformed into an unreadable format. The key ideas are based on:
- Symmetric Encryption: Uses the same key for encryption and decryption. Examples include AES.
- Asymmetric Encryption: Involves a pair of keys, a public key for encryption and a private key for decryption. RSA is a well-known example.
Understanding these concepts requires knowledge in discrete mathematics, number theory, and algebra. Such knowledge helps computer scientists develop new encryption methods and improve existing ones, maintaining security in an increasingly interconnected world.
Optimizing Algorithms with Mathematics
Mathematics is essential in designing and refining algorithms. Through mathematical techniques, developers can analyze the efficiency and effectiveness of algorithms. This involves understanding key elements such as:
- Time Complexity: Determines how the runtime of an algorithm grows with the size of input. Big O notation is commonly used for this analysis.
- Space Complexity: Looks at how the algorithm uses memory resources.
- Algorithm Design Techniques: Includes dynamic programming, greedy algorithms, and divide-and-conquer.
Optimizing algorithms is crucial for performance. For instance, searching algorithms, such as binary search, use mathematical logic to reduce the time necessary to find an element in a sorted list, compared to linear search methods.
Mathematics provides tools to understand these complexities and make informed decisions about algorithm design. By applying mathematical approaches, computer scientists can develop faster and more efficient algorithms, resulting in better software performance.
Ending
The conclusion serves as a critical point in summarizing the extensive discussions that have preceded it. It reinforces the significance of mathematical concepts within the realm of computer science. This article has systematically navigated through various mathematical domains that create the foundation for robust software development, algorithm optimization, and data analysis.
In reflecting on the key insights discussed, it becomes clear that mathematics is not merely an academic requirement but a practical tool that enhances programming efficacy. For instance, discrete mathematics informs the structure of data systems, while calculus offers methods for performing complex analytical tasks. Embracing these mathematical tools can empower students and professionals to tackle real-world computing challenges with greater precision and confidence.
Some benefits of mastering these concepts include:
- Improved problem-solving abilities
- Enhanced algorithm development skills
- A stronger grasp of data structure efficiency
- Better insights into system design and optimization
Furthermore, an understanding of these principles prepares individuals for future innovations in technology. As industries increasingly rely on sophisticated algorithms and data-driven decision-making, the relevance of mathematics continues to expand. The article concludes that fostering a solid grasp of mathematical foundations is essential, not only for personal growth in computer science but also for contributing meaningfully to advancements in the field.
"Mathematics is the language in which God has written the universe" β Galileo Galilei.
Recap of Key Mathematical Concepts
Throughout this article, we have explored several vital mathematical disciplines essential for computer science:
- Discrete Mathematics: Focused on set theory, logic, combinatorics, and graph theory, all of which are essential for understanding algorithms and data structures.
- Linear Algebra: Important for transformations and understanding matrices, which are widely used in graphics and machine learning.
- Calculus: Necessary for understanding changes and applying integration and differentiation in coding algorithms, especially in continuous systems.
- Probability and Statistics: Crucial for working with data, performing inferential statistics, and comprehending predictive models, especially in machine learning.
- Numerical Methods: Key to solving numerical problems through various approximation techniques and root-finding algorithms, directly applicable in complex computing tasks.
- Mathematics in Programming: Covers foundational concepts that enhance software development, enabling better efficiency and effectiveness in creating algorithms.
Future Trends in Mathematics and Computer Science
Looking forward, several trends indicate the growing importance of mathematics in computer science. The integration of artificial intelligence and machine learning continues to evolve, relying heavily on mathematical concepts. New areas like quantum computing emerge, demanding an even deeper understanding of linear algebra and probability.
Personalized data analytics is growing in prominence, underscoring the need for strong statistical foundations. Furthermore, with the rise of big data, knowledge of calculus and numerical methods becomes increasingly advantageous.