Essential Mathematics for Computer Science Guide
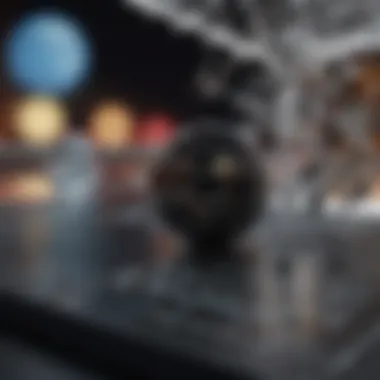
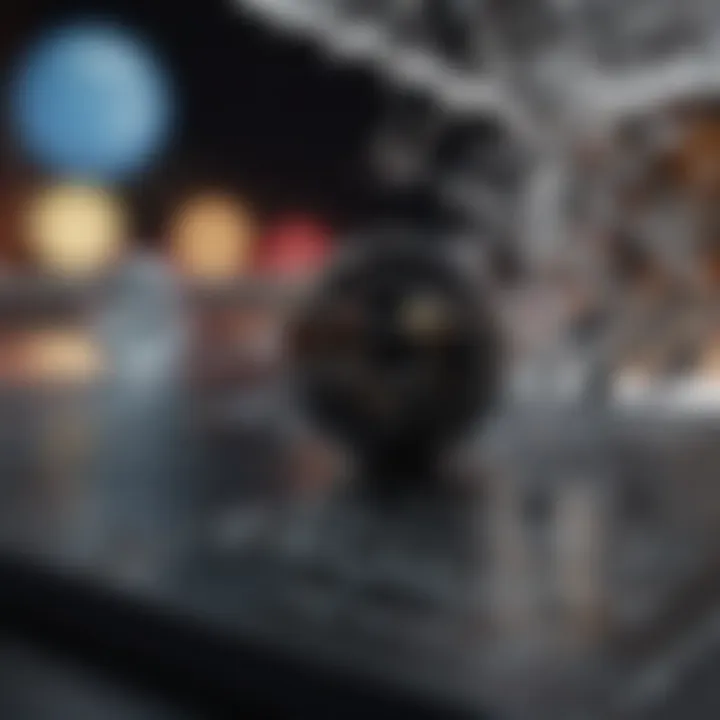
Intro
Mathematics serves as the backbone for many science fields, and computer science is no different. As one embarks on the journey of understanding computer science, grasping the essential mathematical concepts becomes imperative. One might wonder why this is so critically important. After all, isn’t programming about writing code?
The truth is, underneath that stylish code lies a myriad of mathematical principles at play. For instance, think about algorithm efficiency and data structures; they rely heavily on concepts from discrete mathematics. Furthermore, if you're tackling machine learning, that involves statistics and linear algebra. Without a thorough understanding of these areas, one risks navigating the complex waters of computer science without a compass.
In this guide, we’re diving deep into the key mathematical foundations that shape computer science. We will explore these concepts in depth, illustrating their relevance in various computer science domains, from basic programming to complex system designs.
Key Research Findings
In recent years, there has been a surge in understanding the role that mathematics plays in artificial intelligence and big data. Research indicates that mathematical modeling has become indispensable in developing algorithms that underpin these technologies.
Overview of Recent Discoveries
- Machine Learning and Statistics: New studies highlight how statistical learning theory undergirds modern algorithms used in predictive analytics.
- Graph Theory in Networking: Insights have been gained on how graph theory effectively models data connections, making it essential for network routing and optimization.
- Calculus in Graphics and Animation: Discoveries demonstrate that calculus, especially concepts like differentiation and integration, are vital for rendering images and animations in real-time applications.
Significance of Findings in the Field
These findings are not just theoretical; they have practical applications across industries. From designing better algorithms to optimize efficiency in data processing, mathematics integrates seamlessly into every corner of computer science. Thus, understanding these relationships becomes vital for anyone serious about making their mark in the field.
"Mathematics is the script that underwrites the language of algorithms. Without it, we lose clarity in our computational dialogues."
Breakdown of Complex Concepts
Concepts that once seemed daunting can transform into an engaging study with the right perspective and tools.
Simplification of Advanced Theories
- Discrete Mathematics: This area encompasses basic structures like sets, relations, and functions, breaking down complex problems into manageable components. It's essential for understanding data structures like trees and graphs.
- Calculus: It's not magic; it’s a method for tracking change. Whether it’s optimizing a function or understanding motion in computer graphics, calculus helps make sense of it all.
- Statistics: Vital for analyzing data, statistics allows researchers and developers to draw conclusions and make predictions from datasets. Concepts like probability distributions provide insights into data behavior.
Visual Aids and Infographics
To better understand these concepts, visual tools such as graphs and infographics can be immensely useful. For instance, a flowchart demonstrating the relationship between different areas of mathematics and their applications in computer science can create clarity. Infographics comparing statistical methods offer a glance into when to use each method effectively.
Prelude to Mathematics in Computer Science
Mathematics serves as a fundamental pillar upon which the field of computer science is built. The study of algorithms, data structures, and computational theory heavily relies on various branches of mathematics. It’s not merely an academic requirement; it’s a crucial element that shapes how computer scientists tackle complex problems. Understanding mathematical concepts can greatly elevate one’s problem-solving skills, making it easier to design efficient algorithms, understand data flow, and optimize systems.
It’s important to recognize that mathematics promotes logical reasoning and analytical thinking. This is not just about crunching numbers; it reflects a mindset that is critical in tech fields. As technology continues to evolve, so does the need for robust mathematical knowledge and skills.
In order to bridge the gap between abstract mathematical theories and their practical applications in computer science, one must familiarize themselves with the intricate relationships between these disciplines. From the ways that set theory underpins database systems to how probability theory aids in machine learning, the relevance is profound. Mathematical literacy, therefore, is not just an optional skill; it’s essential for anyone aiming to excel in this domain.
The Role of Mathematics in Tech Fields
Mathematics permeates almost every aspect of technology. In software development, algorithms and their efficiency often depend on mathematical constructs. For instance, if a developer knows how to calculate the time complexity using Big O notation, they can predict how their code will perform as data scales.
Moreover, fields such as cybersecurity rely heavily on number theory. Encryption algorithms, critical for secure data transmission, utilize mathematical principles to encode and decode messages. Here are a few ways math shapes essential tech domains:
- Cryptography: Uses advanced algebra and number theory to secure communication.
- Data Science: Employs statistics to analyze and interpret complex datasets.
- Machine Learning: Relies on linear algebra and calculus to optimize model performance.
- Computer Graphics: Applies geometry to manipulate visual representations.
Mathematics is not confined to abstract theories. It is about real-world applications that can solve pressing issues and improve existing technologies.
Why Computer Scientists Need Mathematics
The necessity for mathematics in computer science cannot be overstated. Without a solid grasp of mathematical foundations, a computer scientist may struggle to comprehend complex theories and systems. Here’s why mathematics is indispensable:
- Problem Solving: Mathematics teaches logic and reasoning, which improves problem-solving skills crucial in programming and algorithm development.
- Understanding Algorithms: Many algorithms are based on mathematical principles. For example, searching and sorting algorithms rely on comparisons rooted in statistics.
- Data Analysis: With the explosion of big data, the ability to analyze and interpret data through statistical tools is of paramount importance.
- Enhancing Efficiency: Knowing how to apply mathematical models can lead to optimized solutions that improve performance.
A well-rounded mathematician can contribute to creating innovative solutions by applying theoretical knowledge practically. The impact of mathematics in computer science is clear; it’s a gateway to understanding the more intricate aspects of technology.
Fundamental Concepts in Mathematical Logic
Mathematical logic serves as a cornerstone in the realm of computer science, underpinning various essential concepts that programmers, data scientists, and theorists grapple with daily. In this section, we’ll explore why understanding these fundamental concepts is not just an academic exercise but a critical skill set for navigating the tech landscape. Logic is the bedrock that supports clear reasoning and decision-making, especially when working through algorithms, writing code, or analyzing complex systems.
Propositional Logic
At the heart of mathematical logic lies propositional logic. This area establishes a framework for assessing whether statements are true or false. Statements in this context are known as propositions, and they operate on logical connectors such as "and," "or," and "not." For example, consider two propositions:
- P: It is raining.
- Q: The ground is wet.
In propositional logic, one can deduce that if P is true, Q must also generally hold, assuming typical weather patterns. This kind of reasoning is vital in programming logic, where conditions dictate the flow of execution.
The key takeaway here is that a solid grasp of propositional logic enables computer scientists to formulate logical statements that drive decision-making processes in software applications.
Predicate Logic
Next up is predicate logic, which expands on propositional logic by incorporating quantifiers and predicates. This more sophisticated level of logic allows for the expression of more complex statements about objects and their properties. Unlike propositional logic, which deals only with whole propositions, predicate logic allows assertions about specific variables.
For example, consider the statement:
- "All humans are mortal."
This assertion can be dissected into smaller parts to evaluate its truthfulness, where you might encounter any of the human members in your programming context. It enables developers to represent certain conditions dynamically while interacting with data structures, making it invaluable in database management and artificial intelligence applications.
Proof Techniques
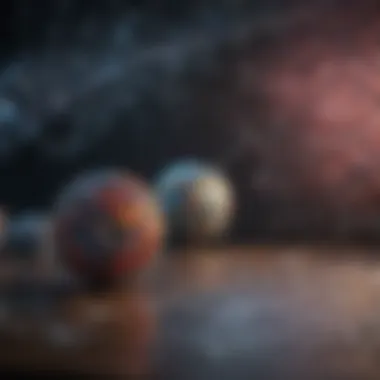
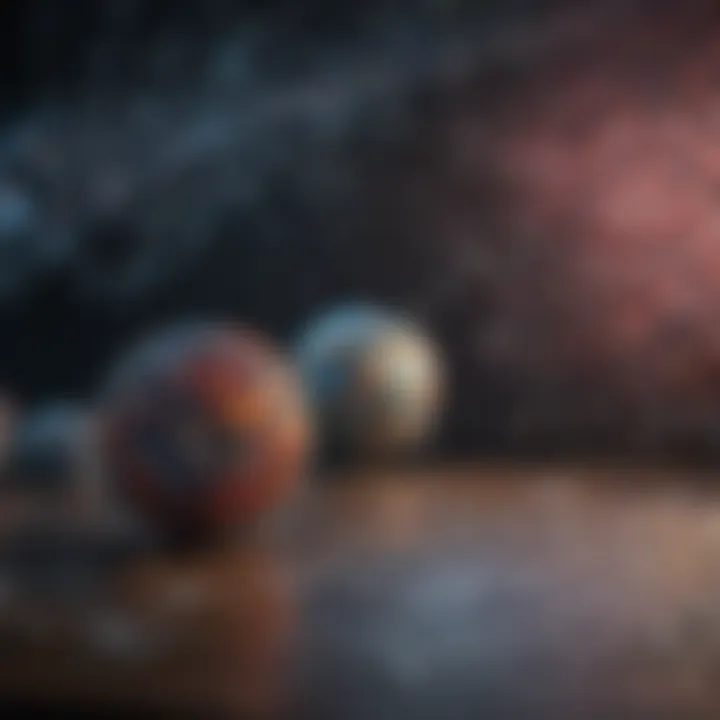
The backbone of reasoning in mathematics is proof techniques, which affirm the validity of propositions using structured arguments. They come in various forms, each with its methodologies and applications. Understanding these techniques is crucial for anyone working in fields that require rigorous validation of concepts.
Direct Proof
In direct proof, one establishes the truth of a statement by straightforwardly following known facts or previously established theorems. It's often seen as the most intuitive proof method because it proceeds in a linear fashion, which can make it easier to understand.
Key characteristic: Direct proofs are generally clear and easy to follow, which makes them a popular choice for computer science reasoning.
One unique feature is their reliance on step-by-step deductions, reinforcing foundational concepts through logical reasoning. However, they might not always apply when dealing with more complex or abstract statements, which can limit their scope.
Indirect Proof
Indirect proofs, or proofs by contradiction, begin by assuming the negation of whatever is being proved. If this assumption leads to a contradiction, then the original proposition must be true. This method can be particularly effective when direct proof appears too cumbersome.
Key characteristic: Indirect proofs shine when direct methods are obscured by complexity. They allow greater flexibility in reasoning across various computer science concepts.
One drawback is that they can sometimes become convoluted, leading to confusion if not adequately structured.
Contradiction
Proof by contradiction systematically shows that the assumption of a proposition's falsehood leads to a conflict with known truths. This method is particularly powerful in formal proofs in computer science, where many principles are refined from established axioms.
Key characteristic: The beauty of contradiction proofs lies in their ability to simplify proof processes by demonstrating unresolvable conflicts arising from initial assumptions.
However, they can often take time to spell out clearly, requiring careful construction to ensure that the contradiction is apparent.
"Understanding these proof techniques not only hones logical reasoning but also enhances problem-solving skills in algorithm design and implementation."
In closing, mastering these fundamental pillars of mathematical logic is indispensable for anyone vested in computer science. They lay the groundwork for further study and application, bridging the gap between abstract logic and practical programming.
Discrete Mathematics: The Backbone of Computing
In the realm of computer science, discrete mathematics serves as an indispensable cornerstone. This field of math focuses on distinct and separate values rather than a continuous range. Its significance in computing cannot be overstated, as many concepts in programming, algorithms, and data structures rely heavily on discrete structures. Understanding these essentials can empower students and professionals alike, enabling them to tackle complex problems effectively.
Through discrete mathematics, one can dissect various topics such as sets, functions, combinatorics, and graph theory—each contributing to robust algorithm design and data processing capabilities.
Sets and Functions
Sets are fundamental constructs in discrete mathematics, defined as collections of distinct objects. Grasping how sets operate lays the foundation for understanding functions, which express relationships between elements of different sets. A function itself provides a clear rule describing how each element from one set corresponds to an element in another.
For example, consider a function connecting students and their assigned grades. If you have a set of students S and a set of grades G, the function maps each student to a unique grade, highlighting the intention and clarity of relationships among data points. Moreover, operations on sets, such as union and intersection, are crucial for managing databases and analyzing information.
Combinatorics
Combinatorics is the study of counting, arrangement, and combination of objects within sets. Its application is vast, impacting algorithmic efficiency in computer science. Understanding permutations and combinations can critically influence decision-making in scenarios involving resource allocation or optimization.
For instance, consider a scenario involving a certain number of channels for data transmission. The ability to calculate how to allocate these channels efficiently based on different combinations can significantly improve data throughput and response time.
Graph Theory
Graph theory extends discrete mathematics into the realm of networks, where relationships between data points are visualized as nodes and edges. Understanding graph theory enhances one's ability to model many real-world systems, such as social networks, transportation logistics, and web structures.
Types of Graphs
There are several specialized types of graphs that each serve unique purposes. These include directed graphs, undirected graphs, weighted graphs, and unweighted graphs. A directed graph has edges that indicate a specific direction, while an undirected graph implies a bidirectional relationship.
For instance, in social networks, a directed graph might illustrate the way one user follows another, whereas an undirected graph can reflect general friendships. The weighted version can indicate the strength or significance of these relationships. Understanding these types is key for tasks like pathfinding or flow optimization, marking their relevance in algorithm development.
Graph Traversal Algorithms
Graph traversal algorithms focus on navigating through these graphs effectively, which is crucial for searching and updating data structures. Depth-first search (DFS) and breadth-first search (BFS) are two primary methods used in these algorithms.
DFS explores as far as possible down one branch before backing up, imitating a thorough investigation. In contrast, BFS explores neighbors first, moving level by level. Both methods provide unique benefits: DFS can be less memory-intensive, while BFS guarantees the shortest path in unweighted graphs, making it a favorable choice in different contexts.
"Understanding the intricacies of graph traversal opens up pathways to advanced problem-solving in algorithm design and practical applications in software development."
Through mastering the concepts of discrete mathematics, from sets and functions to the in-depth exploration of graph theory and its algorithms, individuals ready themselves to better address the challenges posed in the multifaceted world of computer science.
Linear Algebra for Computer Applications
Linear algebra plays a critical role in computer applications, acting as a bridge between mathematical theory and practical computational techniques. It encompasses the study of vectors, matrices, and linear transformations, linking geometrical concepts with their algebraic counterparts. This relationship is vital for numerous domains within computer science, such as graphics, machine learning, and system optimization.
Understanding linear algebra equips computer scientists with a toolkit to formulate and solve problems effectively. Its significance spans various applications, particularly in data manipulation, which is foundational to areas like computer graphics and artificial intelligence.
Vectors and Matrices
In the realm of computer science, vectors and matrices are two important structures that help in representing and processing data. Vectors can be thought of as one-dimensional arrays that hold multiple values, while matrices extend this concept into two dimensions, organizing data into rows and columns.
This organization allows for efficient computation and manipulation, which is important in algorithms such as those used for image processing or numerical simulations. Key operations involving vectors and matrices include addition, scalar multiplication, and matrix multiplication.
Basic Operations:
- Addition: Combining two vectors or matrices of the same size, element by element.
- Scalar Multiplication: Multiplying each element of a vector or matrix by a constant.
- Matrix Multiplication: A more involved operation that combines two matrices to produce a new one, following specific rules regarding their dimensions.
"Linear algebra is the language of many modern computational paradigms. It simplifies complex relationships and makes them analyzable."
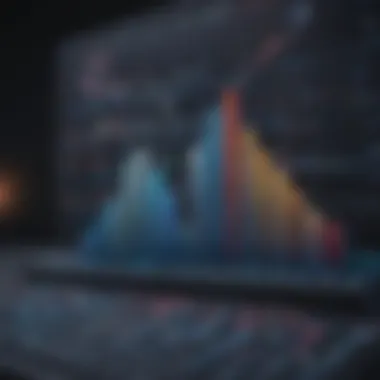
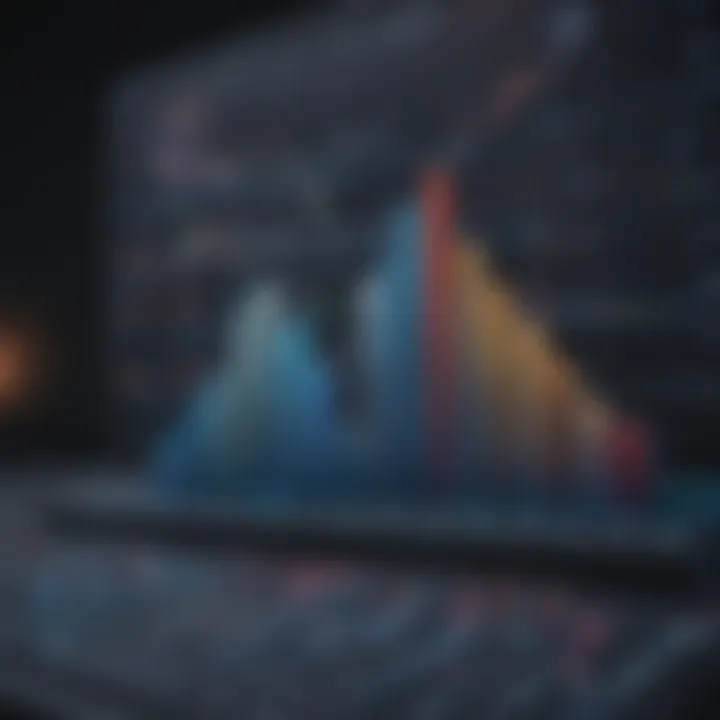
These foundational operations lay the groundwork for more complex mathematical operations encountered in higher-level algorithms.
Eigenvalues and Eigenvectors
Eigenvalues and eigenvectors are pivotal concepts in linear algebra, particularly within the context of dimensionality reduction in data processing. In simpler terms, given a linear transformation defined by a matrix, an eigenvector remains in the same direction after the transformation, while the eigenvalue indicates how much the eigenvector is stretched or compressed.
Understanding eigenvalues and eigenvectors is invaluable, especially in machine learning techniques like Principal Component Analysis (PCA). Here, they help in reducing the number of variables under consideration, thus simplifying complex datasets while retaining their key characteristics. This approach is critical for tasks such as image and speech recognition, where processing power and efficiency are paramount.
Applications in Machine Learning
Machine learning, a significant area in computer science, heavily relies on principles from linear algebra. Concepts like vectors and matrices provide the basis for representing data, while operations such as dot products and matrix factorizations enable effective computations in algorithms.
Some practical applications of linear algebra in machine learning include:
- Feature Extraction: Using techniques like PCA to identify the most critical features within data, enhancing model performance.
- Deep Learning: Neural networks, which function as complex mathematical models, often utilize matrices to represent their parameters and data inputs.
- Recommendation Systems: Matrix factorization aids in identifying hidden patterns in user preferences, helping to suggest products or content users may enjoy.
By anchoring algorithms in linear algebra, machine learning becomes more interpretable and efficient, making it a fundamental component in the toolkit of any computer scientist.
In summary, the study of linear algebra is not just an abstract mathematical pursuit but a crucial part of the fabric that weaves through various computing disciplines. By mastering these concepts, individuals position themselves at the forefront of technological development and applications.
Calculus in Computer Science
Calculus plays a pivotal role in several domains within computer science, especially in areas like algorithms, data analysis, and machine learning. It provides the foundation for understanding how changes occur and assists in making decisions based on those changes. Moreover, calculus enables computer scientists to break down complex problems into manageable parts, allowing for more effective solutions.
Limits and Continuity
Limits are fundamental in calculus as they help in understanding the behavior of functions as they approach specific points. This concept is extremely relevant in programming, particularly in areas such as numerical analysis where evaluating functions at certain points is crucial. For example, when optimizing mathematical functions, determining the limit can guide algorithms to find specific outcomes without needing to compute every possible value.
Continuity, on the other hand, ensures that a function behaves predictably. If a function is continuous, small changes in input will only lead to small changes in output. This predictability is essential in many computer science applications, including rendering in graphics and the stability of algorithms. Without continuity, systems can behave erratically, leading to significant issues in performance and reliability.
Differentiation
Differentiation is about understanding how a function changes; it measures the rate of change. This concept is crucial for optimization problems, allowing us to find maximum or minimum values which are often needed in computer algorithms.
Applications in Optimization
In optimization, derivatives are used to identify the points where functions reach their highest or lowest values. This is particularly beneficial when dealing with algorithms that require efficient resource allocation. For instance, consider a scenario where a company needs to minimize costs while maximizing output. By employing differentiation, one can find the point at which the cost is at its minimum, ensuring the best possible financial decisions are made. The key characteristic here is the ability to gauge not just where the function lies but how it behaves at any given point.
The unique feature of applications in optimization lies in its versatility across different fields—from logistics to artificial intelligence, making it widely applicable. However, challenges can arise, such as when dealing with non-differentiable functions or situations where multiple local minima exist. In these cases, alternative strategies must be employed to ensure reliable outcomes.
Integration
Integration is the opposite of differentiation. It involves aggregating value over an interval, which is crucial in many scientific computations and modeling. In computer science, integration helps in calculating areas under curves, and this can be useful in various applications, including graphics and data analysis.
Numerical Methods
Numerical methods help approximate the value of integrals when it's difficult to calculate them analytically. This is often the case in complex systems or real-world applications where formulas can't be easily derived. The key characteristic of numerical methods is their ability to yield solutions quickly, even when exact answers are impractical.
One unique feature of numerical methods is their adaptability. They can be applied to a plethora of functions, which makes them a favorite in engineering and computer simulations. However, one disadvantage could be the potential for less accuracy depending on the methods used, as well as the trade-offs involved in computational efficiency versus precision.
Applications in Computer Graphics
Applications in computer graphics frequently use integrals to render realistic images through shading and light modeling techniques. In this context, integrals help calculate how light interacts with surfaces, thereby creating a more lifelike representation of a scene. The key here is the seamless blending of mathematical computations with visual outputs, which enhances user experience.
While this integration of calculus in graphics is beneficial, one should also consider the computational cost. Complex scenes can require substantial processing power, which might impact performance on less robust systems. Nevertheless, the reward of improved visual fidelity often outweighs these challenges, making it an area where calculus is genuinely indispensable.
Calculus serves as the bridge connecting mathematical theories with practical applications in computer science, ensuring technologies not only function efficiently but also evolve perpetually.
Statistics and Probability in Computing
In the realm of computer science, statistics and probability serve as the bedrock of decision-making and algorithm design. Every time a program analyzes datasets or makes predictions, it often leans heavily on the tools of statistics and probability. The significance of these subjects can't be overstated; they are essential for understanding data patterns, making informed predictions, and optimizing outcomes in various tech fields.
Without a firm grasp on these concepts, computer scientists would be like sailors navigating without a compass, unsure of where the winds will take them. The following sections break down the vital components of this area, painting a picture of how these principles are utilized in practical applications, enriching one's analytical skills and problem-solving capabilities.
Descriptive Statistics
Descriptive statistics play a pivotal role in summarizing and explaining data. This field involves metrics such as mean, median, mode, and standard deviation. Essentially, it provides a quick glimpse at the data, allowing for an initial understanding of what trends and anomalies may exist. In computer science, these summaries can guide the development of algorithms by providing insights into user behavior, system performance, and much more.
For instance, if a product team wants to understand how users interact with their application, they may analyze session duration or the frequency of feature usage. By applying descriptive statistics, they can find the average session length, identify the most commonly used features, and spot any outliers in user behavior.
Inferential Statistics
While descriptive statistics give us a snapshot of the data, inferential statistics allow us to make predictions about a population based on a sample. This includes hypothesis testing, confidence intervals, and regression analysis. When computer scientists design algorithms, they often rely on inferential stats to validate their models or algorithms by making assumptions and testing hypotheses against datasets.
One common application is in A/B testing. In such scenarios, data scientists compare two versions of a webpage or app feature to see which one performs better. They pull a sample of users and analyze the results to infer which version is more effective, making decisions with higher confidence due to these statistical methods.
Probability Theories and Applications
Probability serves as the backbone for many modern applications in computing. It drives everything from simple algorithms to complex machine learning models. Understanding the fundamental ideas in probability can significantly improve predictions and decision-making processes in technology.
Random Variables
Random variables are a fundamental piece of probability theory. They essentially represent numerical outcomes of random phenomena, allowing computer scientists to assign probabilities to potential outcomes. This characteristic makes them highly useful in modeling scenarios where uncertainty is inherent. For example, when predicting user preferences, random variables can help model a user's action with certain probabilities attached.
A central advantage of random variables is their flexibility. They accommodate all sorts of distributions, letting scientists tailor their approach based on the scenario. However, they require a clear definition of possible outcomes, which may complicate their use in more unpredictable environments.
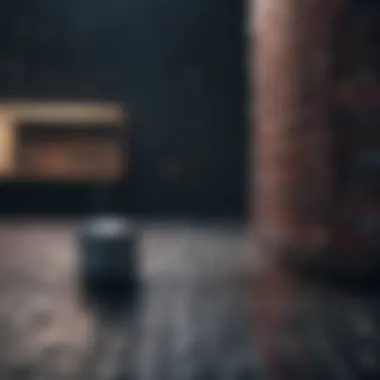
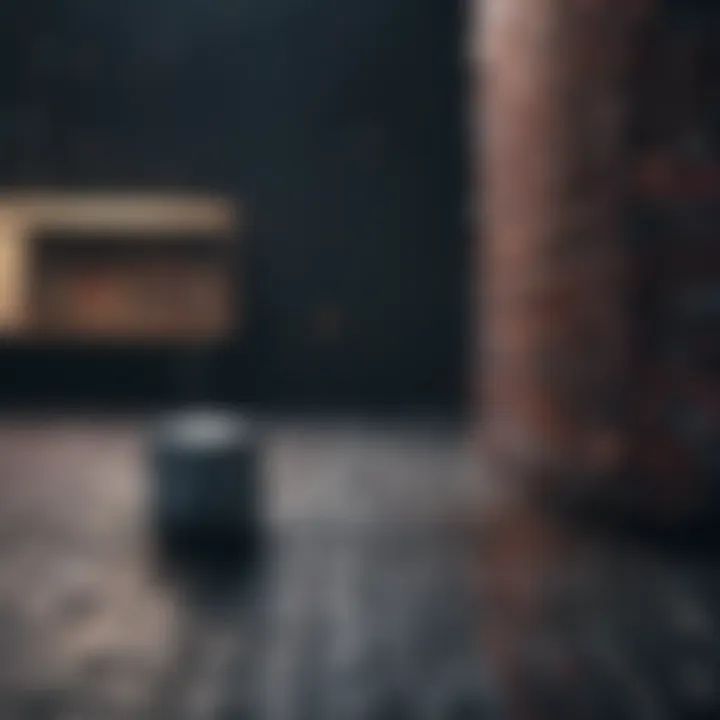
Distributions
Distributions illustrate how random variables can behave under varied circumstances. They describe the likelihood of different outcomes and are crucial in creating models. For instance, a normal distribution can represent user scores on a test, helping predict overall success rates.
Another significant feature is the breadth of distributions available, including binomial, Poisson, and uniform distributions. Each serves different kinds of problems, adding versatility to statistical modeling. However, the downside is that misusing the wrong distribution can lead to incorrect conclusions and inefficiencies in algorithms, which is a pitfall that computer scientists must be aware of.
In summary, statistics and probability are not just academic concepts but practical tools. They empower computer scientists to analyze data effectively, derive meaningful insights, and streamline processes across various applications. Ignoring their relevance could leave one ill-prepared for many challenges in the field.
The Interaction Between Math and Algorithms
Mathematics and algorithms share a fundamental relationship that is often underappreciated. At their core, algorithms are procedures or formulas that provide step-by-step instructions for calculations. Meanwhile, mathematics supplies the necessary tools and models that make these algorithms not just valid, but also efficient and optimized. This synergy is crucial as computer scientists engage in tasks ranging from data sorting to network routing, all of which rely on mathematical principles to function correctly and efficiently.
Understanding how math interacts with algorithms not only enhances their design but also fosters a deeper comprehension of performance metrics. Mathematical concepts help assess how algorithms behave as the size of the input data increases. They also aid in establishing limits and refining solutions for computational problems—adding a layer of depth to the programmer's toolbox.
Complexity Analysis
Complexity analysis is a key aspect of algorithm design, focusing on measuring the efficiency and performance of algorithms. It encompasses both time complexity—how long an algorithm takes to complete as a function of the input size—and space complexity, which measures the amount of memory space an algorithm uses in relation to the input size.
Each algorithm can exhibit different behaviors depending on the nature of its inputs and the operations it performs. For instance, a sorting algorithm like QuickSort may perform splendidly on average but could degrade to O(n²) time complexity with a specific type of input. This kind of analysis allows programmers to choose the right algorithms for their needs.
To better understand complexity analysis:
- Big O Notation: This common measure describes the upper bound on the time or space requirements of an algorithm. For example, O(n), O(log n), and O(n log n) characterize different growth rates.
- Empirical Analysis: Sometimes, theoretical models fall short; running experiments in real scenarios can uncover performance characteristics that are not apparent in mathematical analysis alone.
Sorting Algorithms Metrics
Sorting algorithms are among the most studied topics in computer science, and their evaluation often hinges on specific metrics derived through mathematical analysis. Essential factors to consider include:
- Time Efficiency: This includes average case, best case, and worst-case scenarios. Metrics like average case time complexity provide insights into expected performance.
- Stability: A stable sorting algorithm maintains the relative order of records with equal keys. This aspect often has underlying mathematical properties that dictate behavior.
- In-place Sorting: Some algorithms sort data without additional space. Understanding the mathematical implications behind this efficiency can lead to better decision-making.
Graph Algorithms and Their Mathematical Basis
Graph algorithms play a vital role in a multitude of applications, from social network analysis to pathfinding in maps. The mathematical foundations behind these algorithms rely heavily on graph theory—a domain distinguishable through concepts such as vertices, edges, and paths.
Important mathematical principles include:
- Graph Representations: Understanding various ways to represent graphs (e.g., adjacency lists, adjacency matrices) informs the selection of an algorithm suited for a specific task. Each representation has distinct advantages concerning the mathematical operations it facilitates.
- Traversal Algorithms: Depth-first search (DFS) and breadth-first search (BFS) are fundamental algorithms that traverse graphs and heavily depend on their underlying mathematical properties. Performance analysis often reveals trade-offs in complexity based upon the input structure.
- Shortest Path Algorithms: Algorithms like Dijkstra's and A* pave the way for efficient route calculation by leveraging mathematical heuristics. The interplay of distance calculations and data structures shapes their effectiveness.
In summary, the interaction between mathematics and algorithms is intricate and mutually reinforcing. A deeper understanding of complex mathematical concepts enhances the capability to design, analyze, and optimize algorithms, allowing computer scientists and programmers to tackle challenges with confidence. This connection forms the backbone of computational theory and practical programming, underscoring the importance of a robust mathematical foundation in the field.
Combining Mathematics with Practical Programming
Mathematics isn’t just about numbers and equations; it’s at the heart of effective programming. By merging mathematics with practical programming, developers can enhance the efficacy and efficiency of their coding practices. This intersection is not merely complementary but necessary, especially when tackling complex problems that require precise solutions. Mathematics provides the logical framework necessary for understanding algorithms, which ultimately translates into better code.
Using Mathematical Libraries
In the realm of computing, mathematical libraries are an invaluable resource. They encapsulate optimized algorithms and complex computations, allowing programmers to harness sophisticated mathematical functions without reinventing the wheel.
- Efficiency and Optimization: Libraries such as NumPy provide efficient operations on large arrays and matrices, simplifying computations that would otherwise need extensive coding.
- Accessibility of Advanced Functions: Many libraries come with pre-built functions, enabling programmers to easily perform intricate tasks like linear algebra or statistical analysis without deep diving into the underlying math. For instance, utilizing SciPy for integration or differentiation is straightforward and saves time.
- Community Support: The popularity of libraries often means they are well-supported by a community of users. If an issue arises, or if there's a need to optimize usage, one can easily find solutions or enhancements shared by other developers.
By using these libraries, programmers can devote more time to developing the larger application instead of getting bogged down in the complexities of mathematical computations.
Writing Mathematical Functions
When it comes to programming, writing functions that can handle mathematical computations is crucial, especially for tasks involving data manipulation or algorithm implementation.
To write effective mathematical functions, keep the following in mind:
- Clarity is Key: While it may be tempting to compact code to save space, clear and descriptive functions are essential. Naming conventions matter; a function called instantly conveys its purpose.
- Understand the Mathematics: Before coding any mathematical function, it’s vital to grasp the mathematics behind it. For instance, if you're implementing a function for calculating standard deviation, ensure you understand the statistical principles involved.
- Testing and Validation: It’s not enough to write the function; testing it under various scenarios is imperative. Ensuring that it handles edge cases and returns expected results is part of writing robust code.
Case Studies in Computational Mathematics
Examining case studies offers a tangible insight into the power of blending mathematics with programming. Here are a few instances where this combination flourishes:
- Machine Learning Algorithms: Applying linear algebra and calculus in neural networks can lead to significant improvements in performance. For example, gradient descent, a cornerstone of many machine learning algorithms, employs calculus for optimization and convergence.
- Data Analysis with Statistics: Many organizations utilize programming to analyze large sets of data. Libraries designed for statistical analysis can assist in interpreting data to make informed decisions. A case study involving data mining illustrates how algorithms rooted in probability can extract meaningful patterns from massive datasets.
"Without a solid understanding of the fundamental mathematical principles, developing deep learning models can become a daunting task."
In summary, blending mathematics with practical programming is not just beneficial; it’s essential in computer science. Utilizing libraries, crafting clear functions, and learning from case studies pave the way for innovations that stand the test of time. The relevance of mathematics in this field cannot be overstated, as it anchors the theoretical concepts in real-world applications, thereby allowing for greater creativity and efficiency in programming.
Epilogue: Emphasizing the Importance of Math in Computer Science
In the world of computer science, mathematics is more than just a subject confined to classrooms. It acts as the invisible thread weaving throughout various applications, from algorithms to machine learning. The ability to think mathematically enables computer scientists to design more efficient algorithms and analyze data effectively. Thus, understanding the fundamental concepts of mathematics is essential for anyone wishing to be successful in this field.
Mathematics provides the necessary tools to tackle complex problems and find optimal solutions. When you grasp mathematical principles, you can apply them practically, creating models that abstract real-world phenomena. This is crucial for advancing technology and developing new software solutions. For instance, optimization problems regularly appear in tasks ranging from resource allocation to routing algorithms.
Future Trends and Developments
As we look to the horizon, the integration of mathematics within computer science continues to evolve. Data science is becoming a high-demand field, and expertise in statistics, along with mathematical modeling, is becoming increasingly valuable. The rise of artificial intelligence also underscores the indispensable role of math in developing machine learning algorithms that rely on probabilities, statistics, and calculus.
Data privacy concerns raise interesting questions that blend mathematics with ethics, prompting deeper exploration into cryptography and secure communications. Big data projects harness algorithms derived from linear algebra and statistics to process immense datasets, bringing forth new trends in data management. Moreover, mathematical modeling plays a role in predicting outcomes in various fields, making it a vital skill for developers and analysts alike.
Encouraging Lifelong Learning in Mathematics
In an age where information evolves rapidly, the journey doesn’t end with formal education. Lifelong learning in mathematics is critical for keeping skills sharp and relevant. Continuous self-improvement through advanced courses, workshops, or even online platforms like Coursera or edX can be a game changer for individuals in tech. Engaging in communities—be it through forums, discussion groups, or local meetups—can also significantly enhance your understanding and application of mathematical concepts.
Mathematics is not a one-way street; it’s a dynamic field that invites curiosity and innovation. Whether you are diving into new areas such as quantum computing or exploring better machine learning techniques, maintaining a growth mindset toward mathematics ensures that you stay abreast of the latest trends and technologies.
"Mathematics is the language with which God has written the universe." This quote elegantly encapsulates the essence of mathematics in our technological world. Every line of code we write and every data set we analyze rests on mathematical foundations, making it imperative for computer scientists to grasp its significance deeply.
In summary, recognizing the importance of mathematics enriches the computer science experience and opens doors to new opportunities. Whether future trends intersect with ethical considerations or need for continual learning, mathematics will always remain integral to the discipline.